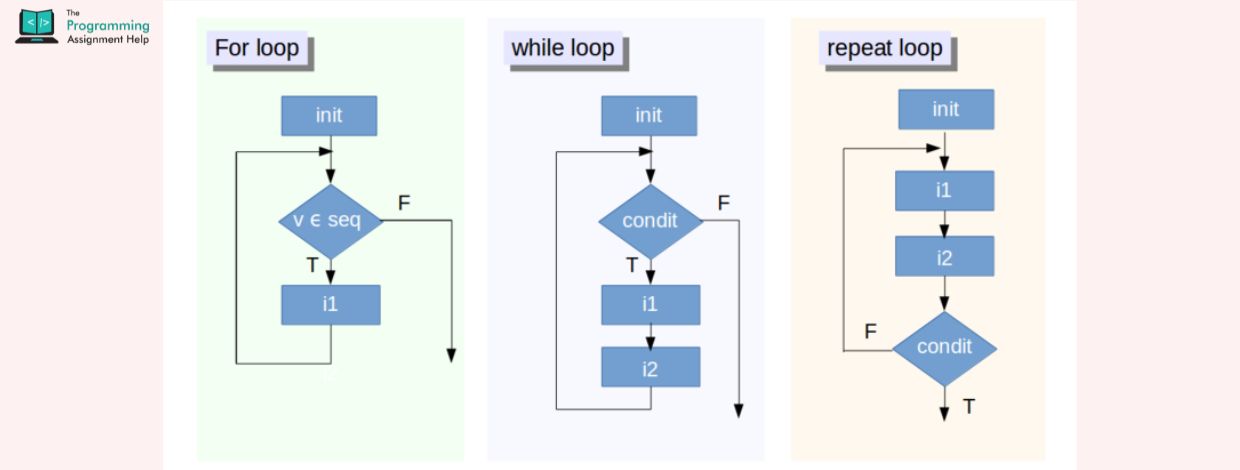
- 31st Jul 2024
- 18:28 pm
- Adan Salman
In this assignment you are tasked with building Python code to solve some sort of problem that plagues you. This can be an academic problem or a work-related problem. On some level, it should be related to accounting, finance, or business in general. At a minimum, your code must incorporate:
- Variables,
- Looping,
- and Logic.
You should give detail info about your problem that builds Python code to solve that problem.
Free Assignment Solution - Incorporating Variables, Looping, And Logic
def transact(funds, stocks, qty, price, buy=False, sell=False):
#A bookkeeping function to help make stock transactions.
if buy is False and sell is False:
raise ValueError("Ambiguous transaction!"
" Can't determine whether to buy or sell!")
return funds, stocks
elif buy is True and sell is True:
raise ValueError("Ambiguous transaction!"
" Can't determine whether to buy or sell!")
return funds, stocks
elif buy is True and sell is False:
cost = price * qty
if cost > funds:
raise ValueError(f"Insufficient funds to purchase "
f"{qty} stock at ${price:0.2f}!")
return funds, stocks
else:
funds = (funds - cost)
stocks += qty
return funds, stocks
elif buy is False and sell is True:
sell_amount = qty * price
if qty > stocks:
raise ValueError(f"Insufficient stock owned "
f"to sell {qty} stocks!")
return funds, stocks
else:
funds = (funds + sell_amount)
stocks -= qty
return funds, stocks
else:
raise ValueError("Ambiguous transaction!"
" Can't determine whether to buy or sell!")
return funds, stocks
#create a class to store the trader details
class Trader:
def __init__(self,balance_,traderName_,qty_=10,stockOwned_=0):
self.cash_balance=balance_
self.traderName=traderName_
self.qty = qty_
self.stocks_owned=stockOwned_
def setBalance(self,balance):
self.cash_balance=balance
def getBalance(self):
return self.cash_balance
def getStockOwned(self):
return self.stocks_owned
def alg_moving_average(self,filename):
"""This function implements the moving average stock trading algorithm.
- Trading must start on day 21, taking the average of the previous 20 days.
- You must buy shares if the current day price is 5%, or more, lower
than the moving average.
- You must sell shares if the current day price is 5%, or more, higher,
than the moving average.
- You must buy, or sell 10 stocks, or less per transaction.
- You are free to choose which column of stock data to use (open, close,
low, high, etc)
- When your algorithm reaches the last day of data, have it sell all
remaining stock. Your function will return the number of stocks you
own (should be zero, at this point), and your cash balance.
- Choose any stock price column you wish for a particular day you use
(whether it's the current day's "open", "close", "high", etc)
"""
# Remember, all your stocks should be sold at the end!
file = open(filename, "r")
read = file.readlines()
read_split = read[0].split(",")
high = read_split.index("High")
val_ = []
total_ = [0]
avg_ = []
for i in range(len(read[1:])):
read_split = read[i+1].split(",")
outcome = float(read_split[high])
val_.append(outcome)
num = 20
for i, a in enumerate(val_, 1):
total_.append(total_[i-1] + a)
if i >= num:
avg = (total_[i] - (total_[i-num]))/num
avg_.append(float(avg))
for i in range(len(avg_)-1):
c1 = val_[i+20] >= (avg_[i] + (0.05 * avg_[i]))
c2 = self.stocks_owned >= 10
c3 = val_[i+20] <= (avg_[i] - (0.05 * avg_[i]))
c4 = self.cash_balance >= (10 * val_[i+20])
if c1 and c2:
self.cash_balance, self.stocks_owned = transact(self.cash_balance, self.stocks_owned, self.qty, val_[i+20], buy=False, sell=True)
elif c3 and c4:
self.cash_balance, self.stocks_owned = transact(self.cash_balance, self.stocks_owned, self.qty, val_[i+20], buy=True, sell=False)
else:
continue
self.cash_balance = self.cash_balance + self.stocks_owned * val_[-1]
self.stocks_owned = 0
def main():
trader=Trader(1000,"Pronab")
# My testing will use AAPL.csv or MSFT.csv
stock_file="Stock Data.csv"
# Call your moving average algorithm, with the filename to open.
trader.alg_moving_average(stock_file)
# Print results of the moving average algorithm, returned above:
print("The results are {0} stocks "
"and ${1:0.2f} balance.". format(trader.getStockOwned(), trader.getBalance()))
if __name__=='__main__':
main()
Get the best Incorporating Variables, Looping, And Logic assignment help and tutoring services from our experts now!
Our team of Python experts has successfully finished this sample Python assignment. The provided solutions are intended solely for research and reference. If you find the reports and code useful, our Python tutors would be happy to help.
- For a complete solution package that includes code, reports, and screenshots, please check out our Python Assignment Sample Solution page.
- For personalized online tutoring sessions to address any questions you have about this assignment, reach out to our Python tutors.
- For additional insights, explore the partial solution available in the blog above.
About The Author - Jordan Lee
Jordan Lee is a skilled Python developer with a focus on solving real-world problems in accounting, finance, and business. With expertise in crafting efficient code, Jordan excels in applying variables, looping, and logical operations to address complex issues. His work often involves creating solutions documented in Word or Excel, ensuring clarity and practical application for both academic and professional needs.