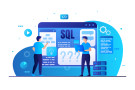
- 30th Nov 2023
- 21:28 pm
- Admin
Structured Query Language (SQL) functions are pivotal tools in database management systems, empowering users to perform diverse operations on stored data. This comprehensive guide explores various types of SQL functions, delving into their syntax, applications, and the ways they elevate database operations.
Types of SQL Functions
SQL functions play a crucial role in database management by facilitating various operations on stored data. Understanding the types of SQL functions is essential for efficient data manipulation.
Aggregate functions:
Aggregate functions are instrumental for summarizing data within a dataset. They operate on a set of values and return a single value.
Prominent aggregate functions include:
- AVG(): Calculates the average of a set of values.
- COUNT(): Determines the number of rows in a dataset.
- FIRST(): Retrieves the first value in a set.
- LAST(): Retrieves the last value in a set.
- MAX(): Identifies the maximum value in a set.
- MIN(): Identifies the minimum value in a set.
- SUM(): Calculates the sum of values in a set.
Scalar functions:
Scalar functions operate on individual values and return a single result. They are crucial for transforming and manipulating data at the individual level. Some essential scalar functions include:
- UCASE(): Converts a string to uppercase.
- LCASE(): Converts a string to lowercase.
- MID(): Extracts a substring from a given string.
- LEN(): Retrieves the length of a string.
- ROUND(): Rounds a numeric value to a specified number of decimal places.
- NOW(): Retrieves the current date and time.
- FORMAT(): Formats a value with the specified format.
Commonly Used SQL Functions
String Functions:
String functions are vital for manipulating character data. They enable users to transform and analyze text-based information. Some commonly used string functions include:
- CONCAT(): Concatenates two or more strings.
- SUBSTRING(): Extracts a substring from a string.
- CHAR_LENGTH(): Retrieves the length of a string in characters.
- TRIM(): Removes leading and trailing spaces from a string.
These string functions are invaluable when dealing with textual data and performing tasks such as data cleansing and formatting.
Mathematical Functions:
Mathematical functions in SQL facilitate numeric operations. They are crucial for performing calculations on numerical data. Some essential mathematical functions include:
- ABS(): Returns the absolute value of a numeric expression.
- CEIL(): Rounds up a numeric value to the nearest integer.
- FLOOR(): Rounds down a numeric value to the nearest integer.
These functions are indispensable in scenarios where mathematical calculations are required, such as financial analyses or scientific computations.
Date/Time Functions:
Date/Time functions are used for manipulating and analyzing temporal data. They provide a range of tools for working with dates and times. Some commonly used date/time functions include:
- GETDATE(): Retrieves the current date and time.
- DATEADD(): Adds a specified time interval to a date.
- DATEDIFF(): Calculates the difference between two dates.
- MONTH(): Extracts the month from a date.
SELECT MONTH('2023-11-08') AS Month;
YEAR(): Retrieves the year from a date.
SELECT YEAR('2023-11-08') AS Year;
GETUTCDATE(): Returns the current UTC date and time.
SELECT GETUTCDATE() AS CurrentUTCDateTime;
These functions are crucial in scenarios where tracking and analyzing time-based trends and patterns are essential.
Aggregate Functions:
Reiterating the significance of aggregate functions, they are extensively used to summarize and analyze data. Whether calculating averages, counting occurrences, or identifying extreme values, aggregate functions provide valuable insights into dataset characteristics.
Advanced SQL Functions
- CASE Statements
CASE statements introduce conditional logic into SQL queries. They enable dynamic data manipulation based on specified conditions.
SELECT
column1,
column2,
CASE
WHEN column3 > 10 THEN 'High'
WHEN column3 > 5 THEN 'Medium'
ELSE 'Low'
END AS Priority
FROM
tableName;
CASE statements enhance query flexibility by allowing conditional transformations based on specified criteria.
- Stored Procedures and User-Defined Functions
Stored procedures and user-defined functions bring modularity and reusability to SQL code. They allow the creation of custom code blocks that can be invoked whenever needed. This modularity simplifies code maintenance and improves overall database organization.
Example of a Stored Procedure:
CREATE PROCEDURE sp_GetEmployeeDetails
@EmployeeID INT
AS
BEGIN
SELECT
*
FROM
Employees
WHERE
EmployeeID = @EmployeeID;
END;
Example of a Scalar UDF:
CREATE FUNCTION fn_GetFullName
(
@FirstName NVARCHAR(50),
@LastName NVARCHAR(50)
)
RETURNS NVARCHAR(100)
AS
BEGIN
RETURN @FirstName + ' ' + @LastName;
END;
Stored procedures and UDFs simplify complex operations, enhance code maintainability, and facilitate code reuse.
- Recursive Functions
While SQL is not traditionally designed for recursion, some database systems support recursive queries. Recursive functions enable iterative processing and are often used in scenarios involving hierarchical data structures. The WITH RECURSIVE clause facilitates the creation of recursive queries.
WITH RECURSIVE EmployeeHierarchy AS (
SELECT
EmployeeID,
ManagerID
FROM
Employees
WHERE
ManagerID IS NULL
UNION ALL
SELECT
e.EmployeeID,
e.ManagerID
FROM
Employees e
INNER JOIN
EmployeeHierarchy eh ON e.ManagerID = eh.EmployeeID
)
SELECT
*
FROM
EmployeeHierarchy;
Advantages of SQL Functions:
- Reusability: Functions can be reused across various parts of a database or in different queries, promoting code efficiency and reducing redundancy.
- Modularity: Functions contribute to code modularity by encapsulating specific functionality, facilitating better management, updates, and troubleshooting.
- Abstraction: SQL functions offer a level of abstraction, enabling developers to concentrate on the logic and behavior of the function rather than the intricate details of its underlying implementation.
- Performance Optimization: Well-optimized functions contribute to improved query performance by encapsulating complex operations that can be precomputed or streamlined.
- Consistency: Functions help enforce consistency by ensuring that specific logic is consistently applied wherever the function is utilized, preventing errors and discrepancies.
Disadvantages of SQL Functions:
- Performance Overhead: Certain functions, particularly those handling complex computations, may introduce performance overhead. It is crucial for developers to find a balance between functionality and performance to ensure optimal application efficiency.
- Limited Portability: SQL functions might not always be portable across different database management systems, leading to potential issues when migrating or using multiple databases.
- Debugging Challenges: Debugging functions can be challenging, especially when dealing with complex logic or nested functions. Proper testing and debugging practices are crucial.
- Security Concerns: Poorly implemented functions may pose security risks, especially if they involve dynamic SQL or user inputs. Parameter validation and permission management are critical for security.
- Maintainability: Overuse of functions or poorly designed functions can lead to code that is difficult to maintain. Clear documentation and adherence to best practices help mitigate this challenge.