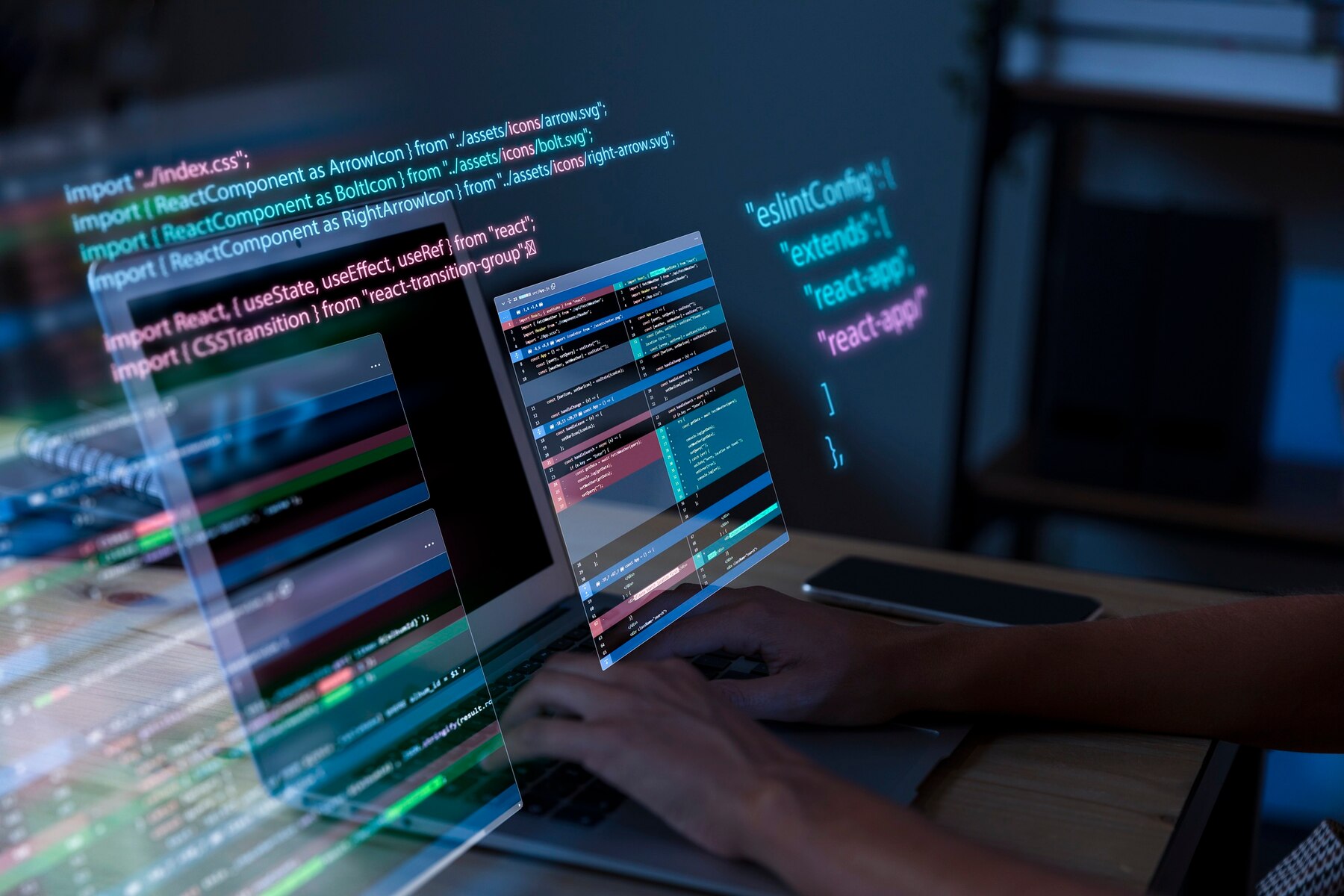
- 27th Dec 2023
- 15:07 pm
- Admin
In Python programming, handling data effectively is essential, and a common obstacle is managing duplicate elements within a list. Duplicate elements can affect the precision of computations and undermine the efficiency of algorithms.
Understanding Duplicates in Python Lists:
In Python, duplicates in a list occur when identical elements appear more than once within the collection. These repetitions may stem from diverse sources, including data input, list merging, or unintentional data duplication during processing. Detecting and managing duplicates is vital for ensuring data integrity and obtaining precise results from algorithms. Python offers various methods to identify and eliminate these duplicates, each tailored to specific scenarios and preferences concerning order preservation and computational efficiency.
Removing Duplicates from a List
Duplicate elements in a Python list can impact the accuracy of computations and complicate data processing. Efficiently removing duplicates is a common task, and Python offers several approaches to achieve this.
- Remove Duplicates from List using Set:
Utilizing the set data structure is a concise way to eliminate duplicates while disregarding their order. The set automatically discards duplicate elements.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(set(original_list))
- Using the Temporary List:
To preserve the order and eliminate duplicates, iterate through the original list, appending elements to a new list only if they haven't been encountered before. This ensures that the resulting list maintains the same order of elements.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = []
[unique_list.append(x) for x in original_list if x not in unique_list]
- Using Dict:
Another approach is to utilize the dict.fromkeys() method, taking advantage of the built-in uniqueness property associated with dictionary keys.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(dict.fromkeys(original_list))
- Using for-loop:
A traditional for-loop can be employed to build a new list without duplicates.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = []
for x in original_list:
if x not in unique_list:
unique_list.append(x)
- Using list comprehension:
Concise and readable, list comprehension achieves the same outcome as a for-loop.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = [x for x in original_list if original_list.count(x) == 1]
- Using Numpy unique() method:
For numerical data, the NumPy library provides the unique() method, simplifying the removal of duplicates.
import numpy as np
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(np.unique(original_list))
- Using Pandas methods:
When dealing with more complex data structures, the Pandas library offers convenient methods for duplicate removal.
import pandas as pd
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = list(pd.Series(original_list).drop_duplicates())
- Using enumerate() and list comprehension:
This method combines enumeration with list comprehension to maintain both index and value uniqueness.
original_list = [1, 2, 2, 3, 4, 4, 5]
unique_list = [value for index, value in enumerate(original_list) if value not in original_list[:index]]
The suitable method is selected based on factors like data complexity, the need for order preservation, and computational efficiency. Python developers can tailor their solution to specific project requirements due to the flexibility of these approaches.
Best Way to Remove Duplicates from a List
The best way to remove duplicates from a list in Python often depends on specific requirements. If preserving the original order is crucial, using a temporary list or employing the enumerate() method with list comprehension is recommended. For numerical data, the NumPy library's unique() method provides a concise solution. When working with more complex data structures, Pandas methods offer convenience. Ultimately, the choice between methods hinges on factors such as simplicity, order preservation, and computational efficiency, allowing developers to tailor their approach to the unique needs of their projects.
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.