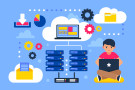
- 17th Nov 2023
- 22:57 pm
- Admin
Object-Oriented Programming (OOP) is a paradigm that focuses on structuring code around objects, allowing for a modular and organized approach to software development. In R Programming, OOP is implemented through various classes and objects. Let's explore two key classes: S3 and S4, along with commonly used objects in R.
Classes in R
R embraces Object-Oriented Programming with two primary classes: S3 and S4. S3, simple and flexible, supports inheritance, generic functions, and attributes. S4, more structured, introduces formal classes, strong typing, and multiple inheritance for comprehensive object-oriented design.
1. S3 Class
S3 class is a simple and flexible OOP system in R. It allows the creation of objects with associated attributes and methods.
- Creating S3 Class
Creating an S3 class involves assigning a class attribute to an object:
# Creating an S3 object
my_s3_object <- 42
class(my_s3_object) <- "my_s3_class"
- Generic Functions
Generic functions operate on S3 objects based on their class attribute. They provide a consistent interface for diverse object types:
# Defining a generic function
my_generic_function <- function(x) UseMethod("my_generic_function")
# Implementing methods for different classes
my_generic_function.my_s3_class <- function(x) x * 2
- Attributes
Attributes provide additional information about an object. They can be accessed using the attr() function:
# Adding an attribute
attr(my_s3_object, "description") <- "This is an S3 object."
- Inheritance in S3 Class
Inheritance allows a class to inherit properties and methods from another class. S3 supports a simple form of inheritance:
# Creating a subclass
setClass("my_subclass", contains = "my_s3_class")
2. S4 Class
S4 class is a more formal and structured OOP system in R. It introduces formal classes with a defined structure and strong typing.
- Creating S4 Class and Object
Creating an S4 class involves defining the class structure and creating an object from that class:
# Creating an S4 class
setClass("my_s4_class", slots = c(value = "numeric"))
# Creating an S4 object
my_s4_object <- new("my_s4_class", value = 42)
- Create S4 Objects from the Generator Function
S4 objects are often created using generator functions, ensuring proper initialization:
# Creating a generator function
generate_s4_object <- function(value) new("my_s4_class", value = value)
# Creating an S4 object
another_s4_object <- generate_s4_object(24)
- Inheritance in S4 Class
S4 supports multiple inheritance, allowing a class to inherit from multiple classes:
# Creating a subclass
setClass("my_s4_subclass", contains = c("my_s4_class", "another_class"))
Objects in R
R Programming boasts diverse built-in objects, each serving specific purposes. Vectors, matrices, lists, arrays, data frames, and factors cater to various data complexities. Understanding and manipulating these objects are pivotal in harnessing the power of R's object-oriented programming paradigm.
- Matrix
The matrix is a two-dimensional data structure organized in rows and columns, and it is generated using the matrix() function:
# Creating a matrix
my_matrix <- matrix(1:6, nrow = 2, ncol = 3)
- Lists
Lists can contain elements of different types, making them versatile data structures:
# Creating a list
my_list <- list(name = "John", age = 25, scores = c(90, 85, 92))
- Vectors
Vectors hold elements of the same data type. They can be numeric, character, logical, etc.:
# Creating a numeric vector
my_vector <- c(3, 5, 7, 9, 11)
- Arrays
Arrays are multi-dimensional extensions of vectors. They can have multiple dimensions:
# Creating a 3D array
my_array <- array(1:24, dim = c(2, 3, 4))
- Dataframe
A dataframe is a two-dimensional table where columns can be of different data types:
# Creating a dataframe
my_dataframe <- data.frame(name = c("Alice", "Bob"), age = c(28, 35))
- Factors
Factors are used to represent categorical data in R:
# Creating a factor
my_factor <- factor(c("High", "Low", "Medium", "High"), levels = c("Low", "Medium", "High"))
In summary, R's O
Applications of R- Object-Oriented Programming
The applications of Object-Oriented Programming (OOP) in R extend across diverse domains, contributing to code organization, scalability, and maintainability.
- Bioinformatics and Genomics: OOP aids in modeling biological entities and analysis pipelines, providing a structured approach to managing complex genomic data.
- Statistical Modeling: OOP facilitates the development of customized statistical models, enhancing modularity and reusability in various statistical analyses.
- Data Visualization: OOP principles are harnessed for building modular and extensible data visualization tools, allowing developers to create reusable and customizable plots.
- Machine Learning: In machine learning, OOP supports the creation of modular frameworks, making it easier to represent different algorithms, models, and preprocessing techniques.
- Package Development: OOP is integral in developing R packages, ensuring well-organized and user-friendly software that can be easily extended by other developers.
- Simulation Studies: OOP is valuable for simulating complex scenarios by modeling different entities and their interactions, providing a structured approach to simulation studies.
- Web Development (Shiny): OOP enhances the development of interactive web applications using Shiny, enabling the creation of modular components for streamlined and maintainable code.
- Geospatial Analysis: OOP aids in modeling spatial objects, contributing to the organized representation and analysis of geographic data in applications related to mapping and spatial analytics.
Advantages of R- Object-Oriented Programming
The advantages of Object-Oriented Programming (OOP) in R are significant, enhancing code efficiency, flexibility, and maintainability.
- Modularity and Reusability: OOP allows developers to encapsulate functionality into modular objects, promoting code reuse and making it easier to manage and maintain.
- Code Organization: Object-oriented programming (OOP) brings a structured approach to code organization, improving readability and facilitating a clearer understanding of the relationships between various components.
- Abstraction and Encapsulation: Abstraction simplifies the representation of complex ideas, while encapsulation restricts access to internal details, fostering a clear separation of concerns.
- Inheritance: Inheritance promotes code reuse by enabling new classes to inherit properties and behaviors from existing ones, minimizing redundancy in the codebase.
- Polymorphism: Polymorphism in OOP allows objects to take on multiple forms, providing flexibility and adaptability in diverse scenarios.
Disadvantages of R-Object-Oriented Programming
Despite its advantages, Object-Oriented Programming (OOP) in R has some potential drawbacks:
- Learning Curve: Transitioning to OOP requires learning new concepts and practices, which can be challenging for those unfamiliar with the paradigm.
- Overhead: OOP can introduce additional complexity and overhead, potentially impacting performance in certain scenarios.
- Design Complexity: Poorly designed object-oriented systems may lead to complex and hard-to-maintain code, especially when the principles of OOP are not followed appropriately.
- Resource Consumption: OOP might consume more memory and computational resources compared to procedural programming, impacting efficiency in resource-constrained environments.
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.