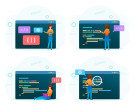
- 13th Nov 2023
- 23:31 pm
- Admin
What are sets in Python?
A set in Python is a collection of distinct and unsorted items. It is defined by surrounding comma-separated components with curly brackets ''. The following are the main properties of sets:
- Uniqueness: Sets have unique elements, which means that each element appears only once in the set. If you attempt to add an element that already exists, it will not be duplicated.
- Unordered Collection: Because sets are stored randomly, the order of the items is lost. The components are sorted according to their hash value.
- Dynamic and mutable: Sets are changeable, which means that components can be added and removed after the set is established. A set's contents can be changed.
- Data Type Support: Sets in Python may include items of many data types such as integers, texts, floats, and even other sets.
- Set Operations: Sets provide a variety of operations for data processing, including union, intersection, difference, and symmetric difference.
Here is an example of defining a set in Python:
```
my_set = {1, 2, 3, 4, 5} # Creating a set with unique elements
print(my_set) # Output: {1, 2, 3, 4, 5}
```
The uniqueness property of sets is particularly useful when you need to handle collections with distinct elements and perform set operations like finding common elements or removing duplicates.
Types of sets in Python
In Python, there are primarily three types of sets:
1. Set
A fundamental data structure in Python, symbolized by curly braces `{}`. It depicts a collection of distinct and haphazard pieces. The following are important characteristics:
- Uniqueness: Sets only include elements that are unique. If you add a duplicate element to a set, it will not be saved multiple times; it will only display once.
- Unordered Nature: Because sets are stored randomly, the order of the components is lost. This implies that items are sorted based on their hash value rather than in any particular order.
- Mutable: Sets are mutable, enabling elements to be added and removed after the set is constructed. A set's contents can be changed by adding or deleting components.
Support for Multiple Data Types: Sets can include items of several data kinds, including integers, texts, floats, and even other sets.
Example of using a set in Python:
```
my_set = {1, 2, 3, 4, 5} # Creating a set with unique elements
print(my_set) # Output: {1, 2, 3, 4, 5}
```
Sets are very helpful for storing distinct components and executing set actions such as locating common elements, deleting duplicates, and detecting differences across collections. They provide efficiency when dealing with unique and unordered data in Python.
```
my_set = {1, 2, 3, 4}
```
2. Frozen Set:
In Python, a frozen set is comparable to an ordinary set with the important feature of being immutable. A frozen set's elements cannot be changed or altered after they are generated. The 'frozenset()' method denotes it. A frozen set's major properties are as follows:
- Variability: The elements of a frozen set cannot be changed after it has been formed. Once the frozen set is defined, you cannot add, remove, or edit elements.
- Unusual and Disorganized: A frozen set, like a conventional set, comprises unique and unordered pieces. It retains discrete components, which are not placed in any specific sequence.
Example of using a frozen set in Python:
```
my_frozen_set = frozenset([2, 4, 6, 8]) # Creating a frozen set
print(my_frozen_set) # Output: frozenset({8, 2, 4, 6})
```
Frozen sets are very handy when you need to verify that a collection of components remains constant or unmodified during the program's execution. Because they are immutable and hashable, they may be used as keys in dictionaries or as elements in other sets. This immutability attribute maintains the data's integrity by avoiding unintentional changes.
3. Mutable Set:
In Python, the term "mutable set" is generally used to refer to the standard, mutable set. In Python, the default set (defined using curly braces `{}`) is mutable by nature. This means that after its creation, elements within the set can be modified - you can add or remove elements.
A changeable set has the following important characteristics:
- Variability: After the set is formed, elements inside it can be changed. You can use the 'add()' method to add items and the'remove()' or 'discard()' methods to delete elements.
- Uniqueness and Unpredictability: A changeable set, like other sets, keeps only unique items and does not guarantee any particular order for its components.
Example of using a mutable set in Python:
```
mutable_set = {5, 7, 9, 11} # Creating a mutable set
print(mutable_set) # Output: {5, 7, 9, 11}
mutable_set.add(13) # Adding an element
print(mutable_set) # Output: {5, 7, 9, 11, 13}
mutable_set.remove(9) # Removing an element
print(mutable_set) # Output: {5, 7, 11, 13}
```
The major difference between a mutable set and a frozen set is that the former enables changes to its items after they are created, whilst the latter is immutable. A mutable set is a dynamic collection that allows for the modification of its components as needed during the execution of a Python program.
Advantages of Sets in Python
Advantages of Sets in Python Because of their unique qualities and functions, Python sets provide various advantages:
- Unique Elements: Sets can only store unique elements. This is useful when working with collections that need different values, as it automatically eliminates duplicates.
- Efficient Set Operations: Because sets offer operations such as union, intersection, difference, and symmetric difference, it is simpler to conduct operations typically employed in mathematical set theory in an efficient manner.
- Membership Testing: Sets provide rapid membership testing with the use of the 'in' operator, which allows for quick tests for the presence of an element in a set.
- Data Integrity: The uniqueness attribute protects data integrity by prohibiting element duplication. This is especially beneficial in situations when data must be separate.
- Frozen Set Immutability: Frozen sets provide immutability, which means that once generated, the set's elements cannot be changed. This can be useful in situations when preserving consistent data is critical.
- Hashability for Quick Lookup: Sets store elements in a hash table, allowing for quick lookup and retrieval. This is very useful when working with enormous datasets.
- Effective Duplicate Removal: Converting lists or other collections containing duplicates to sets can effectively reduce duplicate entries.
Overall, the distinct properties and activities of sets make them useful for organizing collections and conducting a variety of tasks, such as testing for uniqueness, data processing, and quick element lookup and retrieval.
Uses of Sets in Python
Because of its distinctive qualities and operations, Python sets are used in a variety of settings. Among the most popular applications are:
- Removing Duplicates: Sets are effective in removing duplicate elements from a collection, leaving only unique components.
- Membership Assessment: They provide for rapid and straightforward membership verification using the 'in' operator, allowing for quick tests for the existence of items in a set.
- Arithmetic Set Operations: Sets are helpful for executing set-theoretic operations because they support operations such as union, intersection, difference, and symmetric difference.
- Data Filtering: Sets may be used to filter and extract unique items from huge datasets or lists, decreasing duplication.
- Checks for Data Integrity: They may be used to validate data by recognizing unique pieces and highlighting duplicates in a collection.
- Design of Algorithms: When uniqueness or set-based operations are required, sets play an important part in algorithm design, frequently assisting in increasing performance and efficiency.
- Handling Unique Collections: They are appropriate for preserving collections of separate items, with each element appearing only once.
- Set-based Computer Science Problems: They are used to solve numerous computer science issues, such as graph theory and network algorithms.
Overall, sets are adaptable Python data structures that may be used for a variety of applications that involve managing unique components, quick operations, and data integrity.
Write a program in Python using Sets
Python program that utilizes sets to remove duplicates from a list and explain its functionality:
```
# Create a list with duplicate elements
my_list = [1, 2, 2, 3, 4, 4, 5, 6]
# Convert the list to a set to remove duplicates
unique_elements = set(my_list)
# Display the original list and unique elements
print("Original List:", my_list)
print("Unique Elements:", list(unique_elements))
```
Explanation:
The program starts by making a list called'my_list' with duplicate items.
'set(my_list)' is used to turn the list into a set. Because sets only hold unique components, any duplicates in the list are deleted immediately.
The resulting 'unique_elements' collection contains only unique values.
The computer then shows the original list as well as the unique items. It shows how sets may efficiently eliminate duplicates from a collection, leaving only distinct pieces.
By transforming the list to a set, the duplicate elements are removed, leaving only unique elements, giving a rapid and effective approach for data deduplication. This basic example demonstrates how to use sets in Python to manage collections with unique items.