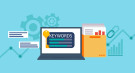
- 3rd Nov 2023
- 15:32 pm
The Python Global Keyword is a fundamental element of Python programming, enabling developers to interact with variables on a global scale. Understanding its use is essential for effective coding. This comprehensive guide dissects the global keyword, elucidating its rules, applications, and best practices.
What is the Python Global Keyword?
The Python Global Keyword is a foundational concept in Python programming, serving as a vital tool for managing variables on a global scale. In the Python language, a variable's scope delineates where it can be accessed and manipulated. Local variables are confined within the boundaries of specific functions, whereas global variables enjoy the liberty of accessibility from any part of the codebase.
Marked by the global keyword, the Python Global Keyword allows programmers to declare a variable as global within a local context. This declaration bestows upon the variable the status of being both accessible and modifiable from both local and global scopes. This distinction becomes crucial when a variable necessitates sharing, accessing, or modifying across different segments of a program, including various functions or modules.
Once a variable is declared as global within a function, it signals Python that the variable's scope extends beyond the local domain, enabling the function to interact with it. This proves especially advantageous when developers must work with global variables within functions while ensuring that any changes made within the function resonate beyond the local context.
In Python, the differentiation between local and global variables is of paramount importance since the interpretation of a variable's scope can profoundly impact code functionality. By harnessing the Python Global Keyword, developers attain a higher degree of control and flexibility concerning variable scope, ultimately nurturing effective and well-organized coding practices.
Rules of Python Global Keyword
The Python Global Keyword is a potent tool for managing variables with a global scope. It empowers developers to create and manipulate global variables within local functions and contexts. To effectively utilize the global keyword in working with global variables, a clear understanding of its rules is imperative:
- Declaration: To designate a variable as global within a local scope, the global keyword, followed by the variable's name, is employed. This declaration communicates to Python that the variable holds a global status, prevailing over any local variable bearing the same name.
- Accessing Global Variables: Once a variable is declared as global in a function using the global keyword, that specific function gains the ability to access and utilize the global variable. In the absence of this declaration, the function treats the variable as local, and any changes remain confined within the function's scope, without affecting the global variable outside.
- Updating Global Variables: Python's Global Keyword is instrumental in allowing functions to modify global variables. When a global variable is altered within a function through the global keyword, the changes endure in the global scope, influencing the variable's value across the entire program.
- Global Keyword in Local Functions: The global keyword can be harnessed within local functions to modify the value of global variables. This functionality is especially valuable when the objective is to adjust a global variable's value within a specific function while preserving its original value outside that particular function.
- Global Variables Across Modules: Python's global variables exhibit the capacity to transcend the boundaries of individual modules. By employing the global keyword, developers can not only access but also modify global variables across various segments of their programs. This versatility enhances code modularity and encourages the reuse of code components.
Use of Python Global Keyword
The Python Global Keyword plays a pivotal role in Python programming by offering developers the means to work seamlessly with variables across different scopes. This functionality is crucial in situations where variables must be accessed, modified, or shared among multiple segments of a program. Here are some key ways the global keyword is employed:
- Accessing Global Variables from Inside the Function
In Python, global variables are accessible from within functions. By using the global keyword, you can access these variables even from the local scope of a function. This accessibility ensures that you can retrieve global variable values within functions and use them to make decisions or perform computations.
global_variable = 10
def access_global():
print(global_variable)
access_global()
# Output: 10
- Updating/Modifying Global Variables from Inside the Function
The global keyword also allows developers to modify global variables from within a local function. By declaring a variable as global, any changes made to that variable inside a function will reflect in the global scope, affecting the variable's value throughout the entire program.
global_variable = 10
def modify_global():
global global_variable
global_variable = 20
modify_global()
print(global_variable)
# Output: 20
- Using the Global Keyword in Local Functions to Modify Global Variable Values
In Python, you can use the global keyword in a local function to modify the value of a global variable. This functionality enables you to control global variables' values dynamically within the local scope of a function, offering flexibility and precision in your code.
global_variable = 10
def local_modify_global():
global global_variable
global_variable = 20
local_modify_global()
print(global_variable)
# Output: 20
Global Variables Across Python Modules
Python is celebrated for its modular design, which enables developers to divide their programs into smaller, reusable components known as modules. Each module can encompass functions, classes, and variables, contributing to effective code organization. However, efficiently managing global variables spanning multiple modules demands a thoughtful approach.
The Python Global Keyword emerges as a vital tool in the context of global variables that necessitate access and modification across diverse modules. By employing the global keyword in different modules, developers can declare a variable as global, rendering it accessible throughout the entire program. Adherence to global keyword conventions is crucial to evade unexpected complications.
When a global variable is declared in one module using the global keyword, it can be accessed and altered from other modules where the same global keyword is utilized for its declaration. This inter-module communication streamlines data flow and guarantees the consistency of global variables, reflecting their most up-to-date values.
This aspect of Python enriches code reusability and simplifies the upkeep of extensive and intricate programs, facilitating uniform interaction with global variables across numerous modules. Nevertheless, it's imperative to maintain a structured and well-organized codebase to stave off potential conflicts or errors when dealing with global variables across modules.
- In module1.py:
global_variable = 10
- In module2.py:
import module1
print(module1.global_variable)
# Output: 10
Python Global in Nested functions
In Python, it's possible to nest functions within one another, creating a hierarchical structure where inner functions are encapsulated within outer functions. This nesting enables inner functions to access variables defined in the outer functions. The crucial role of the Python Global Keyword becomes evident when you're dealing with global variables within these nested functions.
To grasp this concept, consider an outer function that holds a global variable and an inner function attempting to access and alter that variable. By default, inner functions have access to their local scope and the global scope. However, to modify a global variable from an inner function, you need to employ the global keyword.
The presence of the global keyword inside the inner function signals to Python that the variable in question is a global variable, not a local one. This empowers the inner function to access and modify it, bridging the gap between local and global scopes and offering greater control over variable manipulation. This interaction is highly valuable when you need to work with global variables within nested functions and ensure that changes made in inner functions persist outside their local scope.
The Python Global Keyword ensures that variables maintain consistency throughout the nested structure of functions. It grants developers greater control over their code's organization and variable scope. By correctly using the global keyword, you can avoid scope-related issues and maintain a clear distinction between local and global variables, even within nested functions. This helps keep code organized and promotes effective coding practices.
global_variable = 10
def outer_function():
global_variable = 15
def inner_function():
global global_variable
global_variable = 20
inner_function()
print(global_variable) # Output: 20
outer_function()
print(global_variable)
# Output: 20