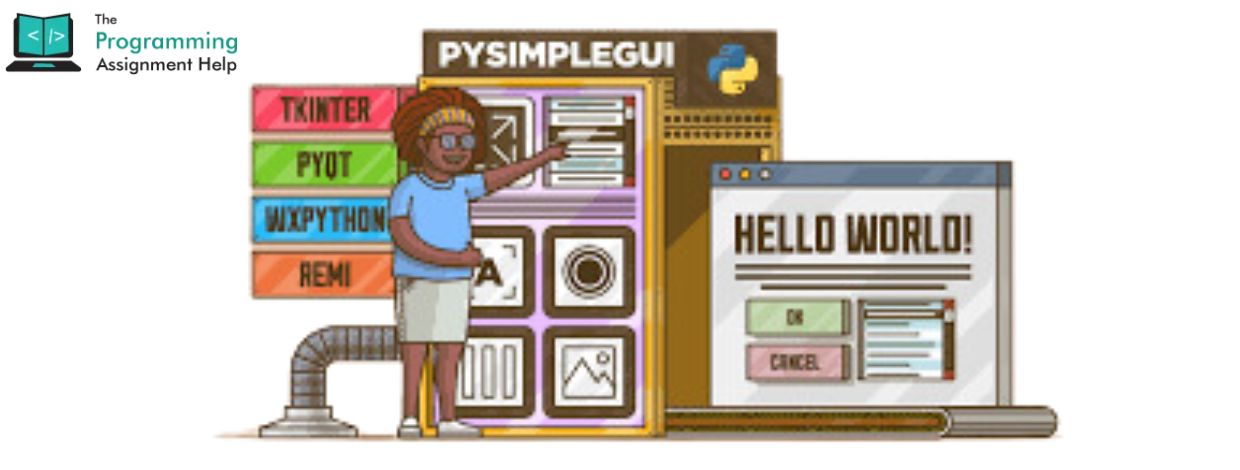
- 9th Jul 2024
- 18:20 pm
- Adan Salman
You have given a working Python coding with Pysimplegui for watermarking app and you need modification to include: 1) Total Image Scanned 2) Save Folder option 3) (Most important) Process status/Progress bar and also the report related to it: Total Image Watermarked Processing Time: Finished at: This is the full Python coding combine with Pysimplegui for everything else other than:
- Total Image Scanned
- Save Folder option
- Process Status with:
- Total Image Watermarked:
- Processing Time
- Finished at:
Python Coding With Pysimplegui For Watermarking App - Get Assignment Solution
Please note that this is a sample assignment solved by our Python Programmers. These solutions are intended to be used for research and reference purposes only. If you can learn any concepts by going through the reports and code, then our Python Tutors would be very happy.
- To download the complete solution along with Code, Report and screenshots - Please visit our Programming Assignment Sample Solution page
- Reach out to our Python Tutors to get online tutoring related to this assignment and get your doubts cleared
- You can check the partial solution for this assignment in this blog below
Free Assignment Solution - Python Coding With Pysimplegui For Watermarking App
"""
Python Script for the images watermarking application.
"""
#importing necessary libraries
import cv2
import numpy as np
import PySimpleGUI as sg
import os
import time,datetime
# function that creates the watermark
"""
Arguments description:
folder: the folder where the images are stored
file: the file name of the image
text: the text to be watermarked
opacity: the opacity of the watermark
fontSize: the font size of the text
"""
def watermark(folder,file,folderOutput,text,opacity,fontSize):
fontSize = fontSize//10 #converting the font size to the size of scale as cv2 only supports image scale of 1 to 10(very large)
opacity = opacity/100 # converting the opacity to the scale of cv2
img = cv2.imread(os.path.join(folder,file)) # reading the image
overlay = img.copy() # copying the image for overlay purpose (used later for the opacity)
font = cv2.FONT_HERSHEY_SIMPLEX # keeping the font as FONT_HERSHEY_SIMPLEX
# Calculating the center coordinates for the text
textsize = cv2.getTextSize(text, font, fontSize, 2)[0]
textX = (img.shape[1] - textsize[0]) // 2
textY = (img.shape[0] + textsize[1]) // 2
# Adding the text to the image with color black and thickness of 2
cv2.putText(img, text, (textX,textY), font, fontSize, (0, 0, 0), 2)
# Alpha value for opacity of the overlay
alpha = 1 - opacity
cv2.addWeighted(overlay, alpha, img, 1 - alpha,0, img)
# the final watermarked file would be saved in the same folder as the image_name + _watermarked.[.jpg/.jpeg/.png]
file = file.split('.')
name = file[0]
extension = file[1]
# writing the image
cv2.imwrite(os.path.join(folderOutput,name+'_watermarked.'+extension),img)
"""
Below is the code for making the GUI using PySimpleGUI
"""
# choices list stores the image file names in the folder selected by the user
choices = []
logs = []
# column1
col1 = [
[sg.Text('Input Images Folder:'), sg.In(size=(25,1), enable_events=True ,key='-FOLDER-'), sg.FolderBrowse()],
[sg.Text('Image Files in the selected folder that would be watermarked:')],
[sg.Listbox(choices, size=(40, len(choices)), key='-FILES-')],
[sg.Text('Total Images Scanned: '), sg.Text('0', key='-TOTAL-',text_color='black',background_color='white'),],
]
# column2
col2 = [[sg.T("")],
[sg.Text('Font Opacity(0-100) '), sg.In(size=(18,1), enable_events=True ,key='-OPACITY-')],
[ sg.Text('Font Size(10-100) '), sg.In(size=(18,1), enable_events=True ,key='-FONT_SIZE-')],
[ sg.Text('Water Mark Text '), sg.In(size=(18,1), enable_events=True ,key='-TEXT-')],
[sg.T("")]]
# column3
col3 = [
[sg.Text('Save Folder:'), sg.In(size=(25,1), enable_events=True ,key='-SAVE_FOLDER-'), sg.FolderBrowse()],
[sg.Text('Process Status: '), sg.Text('Idle', key='-PROCESS_STATUS-',text_color='black',background_color='white'),],
[sg.Listbox(logs, size=(40, len(choices)), key='-LOGS-'),sg.Button('Start', key='-START-'),sg.Button('Exit', key='-EXIT-')]
]
# final layout
layout = [
[sg.Column(col1),
sg.VSeperator(pad=(0, 0)),
sg.Column(col2),
sg.VSeperator(pad=(0, 0)),
sg.Column(col3)
]
]
# creating the window
window = sg.Window('Watermarking Application', layout,auto_size_text=True,
auto_size_buttons=True, resizable=True, grab_anywhere=False, border_depth=5,
default_element_size=(15, 1), finalize=True, element_justification='l')
# GUI event loop
while True:
event, values = window.read()
# if the event is to close the window
if event in (sg.WIN_CLOSED, '-EXIT-'):
break
# if the event is to browse the folder
if event == '-FOLDER-':
folder = values['-FOLDER-']
files = []
if folder is not None and folder != '':
files = os.listdir(folder)
choices =[]
for file in files:
if file.endswith(('.jpg', '.png', 'jpeg')):
choices.append(file)
window['-FILES-'].update( values=choices)
window['-TOTAL-'].update(len(choices))
# if the event is to start the watermarking
if event == '-START-':
text = values['-TEXT-']
opacity = (values['-OPACITY-'])
fontSize = (values['-FONT_SIZE-'])
# checks for the validity of the input
if text==None or fontSize==None or opacity==None:
sg.popup('Please enter all the fields!')
continue
try:
opacity = int(opacity)
if opacity<0 or opacity>100:
sg.popup('Please enter a valid opacity value!')
continue
except:
sg.popup('Please enter a valid opacity value!')
continue
try:
fontSize = int(fontSize)
if fontSize<10 or fontSize>100:
sg.popup('Please enter a valid font size value!')
continue
except:
sg.popup('Please enter a valid font size value!')
continue
folder = values['-FOLDER-']
folderOutput = values['-SAVE_FOLDER-']
if folder == None or folder == "":
sg.popup('Please select a folder to save the watermarked images!')
continue
# calling the watermark function to watermark the images
window['-PROCESS_STATUS-'].update('Processing Images...')
start = time.time()
for file in choices:
watermark(folder,file,folderOutput,text,opacity,fontSize)
end = time.time()
now = datetime.datetime.now()
current_time = now.strftime("%H:%M:%S")
logs = [
f"Total watermarked images: {len(choices)}",
f"Processing time: {end-start} seconds",
f"Finished at time: {current_time}"
]
window['-LOGS-'].update(values=logs)
window['-PROCESS_STATUS-'].update('Done...')
sg.popup('Watermarking Completed!')
"""
End of the script
"""
Get the best Python Coding With Pysimplegui For Watermarking App - Assignment help and tutoring services from our experts now!
About The Author - Emma Smith
Emma Smith is an experienced software developer skilled in Python and adept at using PySimpleGUI to create intuitive user interfaces. With a focus on application enhancement, Emma is dedicated to integrating advanced features such as progress bars and status reports to optimize user interaction and workflow efficiency.