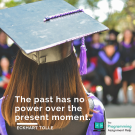
- 29th Oct 2023
- 22:09 pm
- Admin
What is Python Class
A class in Python is an object creation blueprint or template. It outlines the composition and actions of objects built using that class. A class contains both the operations on the data (methods) and the data itself (attributes).
The following are some essential qualities and elements of a Python class:
- Attributes: Within a class, attributes are variables that hold data. The qualities or properties of the objects derived from the class are specified by these data components.
- Methods: Functions defined within a class are called methods. They provide the kind of actions and behaviors that class objects are capable of. Methods have access to and control over the class's attributes.
- Constructor: In Python, the constructor is a special method known as __init__. It plays a crucial role in initializing the attributes of an object when it is created. Upon creating an object, the constructor is automatically invoked to set its initial properties.
- The 'self' argument is utilized within methods to access and manipulate the object's characteristics. This 'self' reference enables methods to interact with the object's attributes, making it a fundamental component of object-oriented programming in Python. It is a reference to the class instance.
- Object: Typically, an object is a class instance. It is a concrete representation of the class with a distinct set of attribute values. Objects can do various activities by calling the methods defined in the class.
Here's a simple example of a Python class:
class Dog:
def __init__(self, name, breed):
self.name = name
self.breed = breed
def bark(self):
return "Woof!"
In this example, the Dog class defines attributes (name and breed) and a method (bark). You can create objects of the Dog class with specific values for the attributes and call the bark method on those objects.
How to create a Python Class
The general steps to creating a Python class are as follows:
- Define the class first: To declare the class, use the `class} keyword and the class name. 'class MyClass:' is an illustration.
- Attributes: Specify which characteristics (data members) the class shall possess. The qualities or traits of objects derived from the class are represented by these attributes. Generally, methods define attributes in the constructor.
- Constructor: Make `__init__` the name of your constructor method. When a class object is created, this method is invoked. It sets the initial values of the object's attributes and may accept arguments.
- Methods: Specify the behaviors and activities that class objects are capable of by defining methods within the class. Methods are functions that may access and change the characteristics of an object by taking {self} as the first parameter.
- Object Creation: By using the class as a function, you may call it to generate objects, or instances, of the class once it has been defined. 'obj = MyClass()', for instance.
- Accessing Attributes and Methods: The dot notation may be used to invoke methods and access attributes of an object. Use 'obj.attribute' to access an attribute, and 'obj.method()' to invoke a method, for example.
- Inheritance: A base class's methods and properties can be passed down to subclasses. You may build customized classes via inheritance by extending preexisting classes.
- Usage: In your code, use the class and its objects to represent real-world items or to model and solve certain issues.
In object-oriented programming, creating a Python class is a basic step that allows you to properly structure and organize your code by encapsulating data and action into reusable, well-defined components.
Objects of Python Class
Python defines an object as an instance of a class. Consider a class as a blueprint and an object as a tangible reality of the blueprint to have a better understanding of this idea. A class specifies the properties (state) and functions (behavior) that objects will have, much like a blueprint describes the layout and composition of a building. Creating an object from a class is similar to building a real building with all of its own features and complexities based on that blueprint.
Consider a class named "Dog," for example. An object built with this class could include characteristics like as breed, age, and name that correspond to a particular dog. The actual values of these attributes—that is, that this dog is a seven-year-old pug with the name Buddy—are included in the state of this object. Through methods defined in the class, such fetching or barking, this object displays behavior.
Three fundamental components make up an object:
- State: This represents the attributes and properties of the item. The state properties for our dog object would be age, breed, and name.
- Behavior: This is how the item behaves, showing how it reacts to events or interactions. Behavior techniques in our case may include playing fetch or barking.
- Identity: Every item in the program has a separate identity that allows it to communicate with other things and keep its distinct existence. When an object is derived from a class, this identity is established.
Similar to real-world things, objects in Python are the building blocks that let you carry out operations, represent information in your code, and describe and operate complicated systems.
__init__() method and __str__() method in Python Classes
`__init__()` Method (Constructor):
The `__init__` method, sometimes known as the constructor, is a unique method. Its main function is to initialize an object's attributes upon creation from a class. Setting initial values for an object's properties is possible using this method, which is automatically used when an object is created. You can provide extra arguments to pass values while constructing objects. It takes'self' as its first parameter, which corresponds to the instance of the class.
The '__init__' method's significance resides in its function in making sure that objects begin with meaningful initial states. It's essential to designing items that faithfully resemble actual entities in order to make them immediately useable. The '__init__' method, for instance, can establish the initial values of a person's name, age, and other characteristics in a 'Person' class.
`__str__()` Method (String Representation):
Another unique Python way for providing a human-readable string representation of an object is called `__str__` Python searches for this method to specify how an object should be turned to a string when you use `str(obj)` on it.
The `__str__` technique is significant since it improves debugging and readability of code. It enables you to design an understandable and informative string representation for things, so that when they are printed or recorded, it is simpler to comprehend their characteristics or condition. To improve readability when shown or recorded, the `__str__` function in a `Book` class, for instance, can return a string including the book's title, author, and ISBN.
Both of these special methods contribute to creating more usable and maintainable classes and objects in Python.
Example of Python Class and it’s explanation
Let's create a simple Python class, `Person`, and explain its components. This class will represent a person with attributes like name and age, and it will have a method to introduce themselves.
```
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def introduce(self):
return f"Hi, I'm {self.name}, and I'm {self.age} years old."
# Create an object of the Person class
john = Person("John", 30)
# Access attributes and call methods
print(john.name) # Output: John
print(john.age) # Output: 30
print(john.introduce()) # Output: Hi, I'm John, and I'm 30 years old.
```
Now, let's break down the components of this `Person` class:
- Class Declaration (`class Person`): The `class` keyword is used to declare a class. In this case, we're '__str__' is another special method in Python that gives an object a human-readable string representation. When you use `str(obj)` on an object, Python looks for this method to define how it should be converted to a string.
The '__str__' approach is important since it enhances code readability and debugging. It allows you to create a clear and informative string representation for objects so that their properties or states are easier to understand when they are printed or recorded. The '__str__' function in a {Book} class, for example, can return a string including the book's title, author, and ISBN to improve readability when exhibited or recorded.
- Methods ({def introduce(self)}): Class-defined functions are called methods. They reflect the activities or behaviors the thing is capable of. The `introduce` method in this example returns a greeting along with the individual's name and age.
- Object Creation ({john = Person("John", 30)}): The {Person} class is used to construct an object named {john}. The supplied values are used by the constructor to initialize the attributes.
- Accessing Attributes and Calling Methods ({john.name}, {john.age}, {john.introduce(){): The dot notation is used to access the attributes of the object and to call its methods.
The development and use of a Python class are demonstrated in this example. It enables you to create and interact with instances of that class by encapsulating data (name and age) and behavior (introduction) within a class.