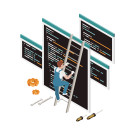
- 11th Dec 2023
- 16:45 pm
In PySpark, `pivot()` is a function that allows you to rotate or pivot a DataFrame, changing the layout of the data by transforming values from one column into multiple columns. This operation is useful for reshaping and summarizing data.
The `pivot()` function typically involves three main parameters:
- pivot_col: This is the column whose unique values will become the new column headers after the pivot operation.
- values: This is the column whose values will be aggregated and placed in the new columns created by the pivot operation.
- aggfunc: This parameter specifies the aggregation function to be applied when there are multiple values for a single combination of the pivot and value columns. Common aggregation functions include 'sum', 'avg', 'max', 'min', etc.
Here's a simple example to illustrate how `pivot()` works in PySpark:
```
from pyspark.sql import SparkSession
from pyspark.sql.functions import col
# Create a Spark session
spark = SparkSession.builder.appName("example").getOrCreate()
# Sample data
data = [("Alice", "Product_A", 10),
("Bob", "Product_B", 20),
("Alice", "Product_A", 15),
("Bob", "Product_C", 25)]
# Define the schema
schema = ["Name", "Product", "Amount"]
# Create a DataFrame
df = spark.createDataFrame(data, schema=schema)
# Pivot the DataFrame
pivot_df = df.groupBy("Name").pivot("Product").sum("Amount")
# Show the result
pivot_df.show()
```
The 'pivot()' operation is used on the "Product" column in this example, and the values in the "Amount" column are aggregated using the'sum()' function. The resulting DataFrame will comprise columns for each distinct product, with values representing the sum of dollars for each individual and product combination.
Keep in mind that the `pivot()` function is available starting from PySpark version 1.6.0.
What are the uses of PySpark Pivot() DataFrame Function
The `pivot()` function in PySpark is a powerful tool for reshaping and summarizing data in a DataFrame. Some of the common use cases for the `pivot()` function include:
- Reshaping Data: The main purpose of 'pivot()' is to reshape data by changing values from one column to many columns. When you wish to change the layout of the data for better analysis or visualisation, this can be useful.
- Creating Pivot Tables: The 'pivot()' function can be used to generate pivot tables, which are a method of summarising data in a tabular format. You can create a summary table that provides insights into your data by selecting selected columns to act as rows, columns, and values.
- Aggregating Data: When used in conjunction with aggregation methods such as'sum()', 'avg()','max()', and so on, 'pivot()' allows you to aggregate data for each combination of values in the pivot and values columns. This is handy for summarising data depending on specific criteria.
- Handling Categorical Data: If you have categorical data that you want to convert into columns, the `pivot()` function can be useful. It's a way to transform categorical values into a more structured format suitable for analysis.
- Analysing Time Series Data: When analysing time series data, you may need to pivot the data to see trends or patterns over time. Use the 'pivot()' technique to rearrange time series data for better analysis.
- Data Preparation for Machine Learning: You may need to change your data in some cases to make it appropriate for machine learning techniques. Pivoting can help you reorganise your data so that it meets the input requirements of various Machine Learning models.
Advantages of PySpark pivot() DataFrame Function
The `pivot()` DataFrame function in PySpark offers several advantages, making it a valuable tool for reshaping and summarizing data in distributed computing environments. Here are some advantages of using the `pivot()` function in PySpark:
- Changing the Data Structure: The ability of 'pivot()' to modify the structure of the DataFrame is its key advantage. It enables you to convert long-format data (with many rows and few columns) into a wide-format Data Structure for simpler analysis and visualisation.
- Flexible Aggregation: When combined with aggregation methods (for example,'sum()', 'avg()','max()'), 'pivot()' allows you to conduct flexible aggregations on the values in the DataFrame. This is especially handy for summarising data depending on several parameters.
- Categorical Data Handling: The function is useful for handling categorical data by converting unique values in a column into separate columns. This can simplify the representation of categorical variables for analysis or machine learning tasks.
- Creation of Pivot Tables: With `pivot()`, you can easily create pivot tables, which are common tools for summarizing and presenting data. Pivot tables are especially useful for multidimensional analysis and reporting.
- Efficient Parallel Processing: PySpark is built on top of Apache Spark, a distributed computing framework. The `pivot()` function leverages Spark's parallel processing capabilities, enabling efficient processing of large-scale datasets across a cluster of machines.
- Improved Data Analysis and Exploration: Reshaping data with `pivot()` can lead to more efficient Data Analysis and exploration. It can help uncover patterns, trends, and relationships within the data that might be challenging to observe in its original format.
- Time Series Analysis: The 'pivot()' function can be used to organise time-dependent data into a structure suited for trend analysis, forecasting, and other time-related tasks.
- Integration with the Spark Ecosystem: Because PySpark is part of the Apache Spark ecosystem, the 'pivot()' function works seamlessly with other Spark components like Spark SQL and MLlib, allowing for end-to-end data processing and analysis.
- Scalability: PySpark is designed to handle large-scale distributed data processing. The `pivot()` function is scalable and can efficiently process massive datasets by leveraging the parallel processing capabilities of Spark.
- Consistent API: PySpark provides a consistent API for working with distributed data processing. The 'pivot()' method adheres to the PySpark DataFrame API norms, making it simple for PySpark users to work with and integrate into their data workflows.
While the 'pivot()' function provides these benefits, it is crucial to remember that its application is dependent on the unique needs of your data analysis or processing jobs. It is always best to select the appropriate tools and procedures based on the type of your data and the objectives of your study.
Program using PySpark's `pivot()` DataFrame Function
In this example, we'll create a PySpark DataFrame, pivot the data based on the "Subject" column, and calculate the average score for each student and subject combination.
```
from pyspark.sql import SparkSession
from pyspark.sql.functions import col
# Create a Spark session
spark = SparkSession.builder.appName("PivotExample").getOrCreate()
# Sample data
data = [("Alice", "Math", 90),
("Bob", "Math", 85),
("Alice", "Physics", 88),
("Bob", "Physics", 92),
("Alice", "Chemistry", 78),
("Bob", "Chemistry", 80)]
# Define the schema
schema = ["Student", "Subject", "Score"]
# Create a DataFrame
df = spark.createDataFrame(data, schema=schema)
# Pivot the DataFrame to calculate the average score for each student and subject
pivot_df = df.groupBy("Student").pivot("Subject").avg("Score")
# Show the result
pivot_df.show()
```
In this program:
- We create a Spark session using `SparkSession.builder.appName("PivotExample").getOrCreate()`.
- We define sample data with information about students, subjects, and their scores.
- We use'spark.createDataFrame(data, schema=schema)' to create a DataFrame 'df'.
- We pivot the DataFrame depending on the "Subject" column using the 'pivot()' method, and we calculate the average score for each student and subject combination using 'df.groupBy("Student").pivot("Subject").avg("Score")'.
Finally, we show the resulting DataFrame using `pivot_df.show()`.
The resulting DataFrame ('pivot_df') will have columns for each distinct subject, with values representing the average score for each student and subject combination. This is only an example; you can modify the program to meet your individual data and analytic needs.