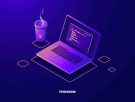
- 11th Dec 2023
- 15:44 pm
- Admin
PySpark GroupBy Function
In PySpark, the `groupBy()` function is used to group the rows of a DataFrame based on one or more columns. After grouping, you can perform various aggregate operations on the grouped data. The `groupBy()` function is a crucial step in many data analysis and processing tasks, especially when you need to summarize or aggregate data based on certain criteria.
Here's the basic syntax of the `groupBy()` function:
```
DataFrame.groupBy(*cols)
```
- **cols**: One or more column names or expressions that will be used to group the DataFrame. Multiple columns can be given as independent arguments or as a list.
After using 'groupBy(),' you can compute summary statistics for each group using aggregate methods such as'sum(),' 'avg(),''max(),''min(),' and so on.
The 'groupBy()' method in PySpark is a crucial aspect of data manipulation and summarization, allowing you to organise and analyse your data depending on particular criteria.
Uses of PySpark GroupBy function
The PySpark 'groupBy()' method is a useful tool for organising, aggregating, and summarising data in a DataFrame depending on one or more columns. The 'groupBy()' function is commonly used in the following scenarios:
- Aggregation and Summarization:
'groupBy()' is mostly used to aggregate and summarise data based on certain criteria. For each group in your DataFrame, you may compute summary statistics such as sums, averages, counts, min/max values, and so on.
```
# Example: Calculate the average score for each subject
df.groupBy("Subject").avg("Score").show()
```
- Group-wise Transformation: Group-wise transformations can be performed on columns within each group. For example, you may compute each row's % contribution to the total inside its group.
```
# Example: Calculate the percentage contribution of each student's score to the total score in their subject
df.groupBy("Subject", "Student").agg((col("Score") / sum("Score")).alias("Percentage")).show()
```
- Group Filtering: You can filter groups based on specified conditions, allowing you to focus on specific portions of your data.
```
# Example: Filter groups where the average score is greater than 85
df.groupBy("Subject").avg("Score").filter(col("avg(Score)") > 85).show()
```
- Combining with aggregating procedures: To do complicated analysis on your data, use 'groupBy()' with other aggregating procedures.
```
# Example: Calculate the total score and average score for each student
df.groupBy("Student").agg(sum("Score").alias("TotalScore"), avg("Score").alias("AverageScore")).show()
```
- Multi-column Grouping: Data can be grouped based on multiple columns, allowing for more granular analysis.
```
# Example: Group by both "Subject" and "Student" to calculate the total score for each subject and student combination
df.groupBy("Subject", "Student").agg(sum("Score").alias("TotalScore")).show()
```
- Statistical Analysis: To do statistical analysis on subsets of your data, use 'groupBy()'.
```
# Example: Calculate the standard deviation of scores for each subject
df.groupBy("Subject").agg(stddev("Score").alias("StdDev")).show()
```
- Time Series Analysis**: If your DataFrame contains a timestamp column, you can use 'groupBy()' to group data for time series analysis depending on time intervals.
```
# Example: Group by day and calculate the average score for each day
df.groupBy(window("Timestamp", "1 day")).avg("Score").show()
```
When paired with other PySpark DataFrame functions and aggregation methods, the 'groupBy()' function provides a versatile framework for analyzing and summarising data depending on various criteria. It's an important part of PySpark's expressive and functional distributed data processing API.
Advantages of PySpark GroupBy Function
The PySpark 'groupBy()' function has various characteristics that make it a useful tool for data processing and analysis in distributed computing settings. Here are some of the primary benefits:
- Data summarising: The primary function of 'groupBy()' is to enable data summarising by grouping rows according to certain criteria. This is critical for collecting aggregated statistics for subsets of data, such as sums, averages, counts, and so on.
- Grouping Flexibility: 'groupBy()' allows you to select one or more columns for grouping. This enables for more granular control over data grouping, allowing for more sophisticated analyses.
- Aggregation Functions: To execute computations on aggregated data quickly, the function can be simply combined with other aggregation functions (e.g.,'sum()', 'avg()','max()','min()', etc.).
- Effective Parallel Processing: PySpark, which is based on Apache Spark, is intended for distributed computing. The 'groupBy()' function takes advantage of Spark's parallel processing capabilities, making it appropriate for large-scale datasets and enhancing computational performance.
- Multi-Column Grouping: It allows you to group data by many columns at the same time, allowing you to analyse data across various dimensions. This is beneficial for more complex analytics and reporting.
- Combination with Window Functions: When used with window functions such as 'rank()', 'lead()', or 'lag()', 'groupBy()' allows for advanced time-series analysis and row-level computations inside each group.
- Statistical Analysis: The function 'groupBy()' can be used to run statistical studies on multiple groups, allowing users to gain insight into the distribution and variability of data within each group.
- Data Filtering Within Groups: This function allows you to filter data within each group depending on certain criteria. This is useful for focusing on data subsets that meet specific criteria.
While the 'groupBy()' function is strong, it must be used with caution, taking into account the structure of your data and the unique requirements of your research. Efficient use of 'groupBy()' can improve the speed and scalability of data processing activities in a distributed environment.
A program using PySpark GroupBy Function
In this example, we'll group the data by the "Subject" column and calculate the average score for each subject.
```
from pyspark.sql import SparkSession
from pyspark.sql.functions import col, avg
# Create a Spark session
spark = SparkSession.builder.appName("GroupByExample").getOrCreate()
# Sample data
data = [("Alice", "Math", 90),
("Bob", "Math", 85),
("Alice", "Physics", 88),
("Bob", "Physics", 92),
("Alice", "Chemistry", 78),
("Bob", "Chemistry", 80)]
# Define the schema
schema = ["Student", "Subject", "Score"]
# Create a DataFrame
df = spark.createDataFrame(data, schema=schema)
# Group by the "Subject" column and calculate the average score for each subject
grouped_df = df.groupBy("Subject").agg(avg("Score").alias("AverageScore"))
# Show the result
grouped_df.show()
```
In this program:
- We start a Spark session by calling SparkSession.builder.appName("GroupByExample").getOrCreate()'.
- We define sample data as data about students, subjects, and their grades.
- We use'spark.createDataFrame(data, schema=schema)' to create a DataFrame ('df').
- We organise the DataFrame by the "Subject" column using the 'groupBy()' function.
- Using 'avg("Score"), we calculate the average score for each subject using the 'agg()' function.alias("AverageScore")'.
- Finally, we use 'grouped_df.show()' to display the resulting DataFrame ('grouped_df').
The end result will be a DataFrame with the average score for each subject. You can customise the program to meet your individual use case and data analysis needs.