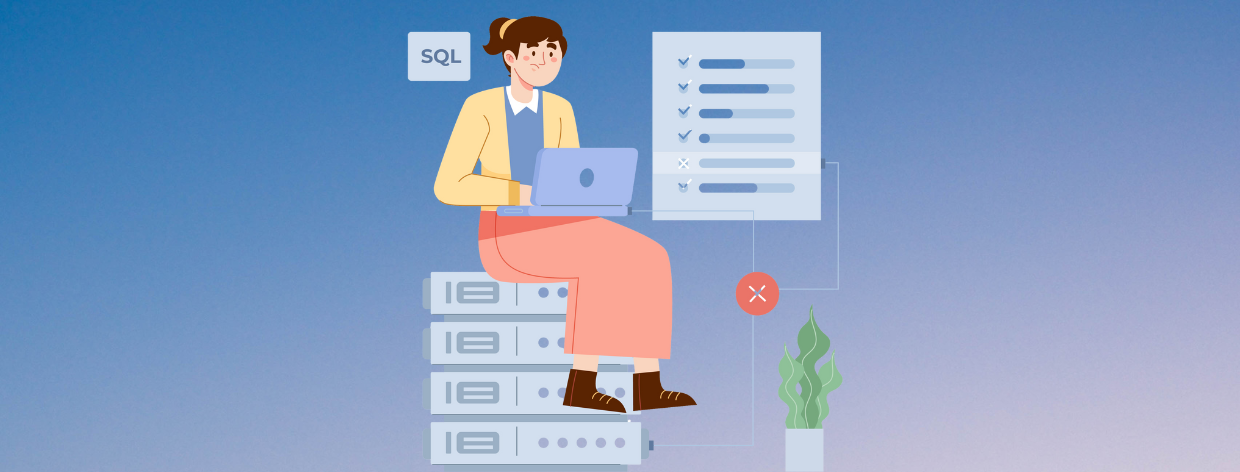
- 30th Nov 2023
- 14:37 pm
- Admin
Structured Query Language (SQL) forms the foundation of database operations, and PL/SQL (Procedural Language/Structured Query Language) enhances SQL by introducing procedural programming features. This powerful combination is widely employed in Oracle databases, offering a seamless integration of SQL queries with procedural constructs.
What is PL/SQL?
PL/SQL, short for Procedural Language/Structured Query Language, serves as an extension of SQL aimed at augmenting the functionalities of traditional SQL with the inclusion of procedural programming constructs. Originating from Oracle Corporation, PL/SQL seamlessly blends elements of procedural languages—like conditional statements, loops, and exception handling—with SQL statements. This integration empowers developers to craft resilient, efficient, and modular database applications within the Oracle Database framework. Known for harmonizing the simplicity and potency of SQL with the flexibility and control of procedural programming, PL/SQL offers a comprehensive toolkit for database development and management.
PL/SQL Variables and Constants
- PL/SQL Variables:
Variables in PL/SQL are containers that store data values. They are essential for temporarily holding values during the execution of a PL/SQL block. Defining a variable requires specifying its name, data type, and, if desired, an initial value.
- PL/SQL Constants:
Constants function similarly to variables; however, once assigned a value, they cannot be altered. Constants offer a means to store values that remain fixed throughout the execution of a PL/SQL block.
DECLARE
v_employee_id NUMBER := 101;
v_employee_name VARCHAR2(50) := 'John Doe';
BEGIN
DBMS_OUTPUT.PUT_LINE('Employee ID: ' || v_employee_id);
DBMS_OUTPUT.PUT_LINE('Employee Name: ' || v_employee_name);
END;
/
Control Statements in PL/SQL
- PL/SQL IF Statement
The IF statement in PL/SQL allows for conditional execution of code blocks based on a specified condition. It enhances the decision-making capability of PL/SQL programs.
DECLARE
v_salary NUMBER := 50000;
BEGIN
IF v_salary > 60000 THEN
DBMS_OUTPUT.PUT_LINE('High Salary');
ELSE
DBMS_OUTPUT.PUT_LINE('Low Salary');
END IF;
END;
/
- PL/SQL Case Statement
The CASE statement simplifies complex conditional logic by providing a concise way to evaluate multiple conditions and execute corresponding blocks of code.
DECLARE
v_day NUMBER := 3;
BEGIN
CASE v_day
WHEN 1 THEN
DBMS_OUTPUT.PUT_LINE('Sunday');
WHEN 2 THEN
DBMS_OUTPUT.PUT_LINE('Monday');
WHEN 3 THEN
DBMS_OUTPUT.PUT_LINE('Tuesday');
-- Add other cases as needed
ELSE
DBMS_OUTPUT.PUT_LINE('Invalid Day');
END CASE;
END;
/
- PL/SQL Loop Statements
- PL/SQL Exit Loop: The EXIT statement allows for the termination of a loop based on a specified condition, providing flexibility in controlling loop execution.
- PL/SQL While Loop: The WHILE loop runs a block of code as long as a specified condition remains true. It proves beneficial in scenarios where the number of iterations is not predetermined.
- PL/SQL For Loop: The FOR loop offers a convenient mechanism for iterating over a range of values, making it especially handy for repetitive tasks where the number of iterations is predetermined.
- PL/SQL Continue: The CONTINUE statement serves to bypass the remaining code within the current iteration of a loop, allowing the program to proceed to the next iteration.
- PL/SQL GOTO: The GOTO statement allows for unconditional branching to a labeled statement within the PL/SQL block. While its use is discouraged in modern programming, it offers a way to transfer control to a specific point.
DECLARE
v_counter NUMBER := 1;
BEGIN
-- Simple LOOP
LOOP
EXIT WHEN v_counter > 5;
DBMS_OUTPUT.PUT_LINE('Counter: ' || v_counter);
v_counter := v_counter + 1;
END LOOP;
END;
/
PL/SQL Architecture
- PL/SQL Function
A PL/SQL function is a reusable unit of code that performs a specific task and returns a value. Functions enhance modularity and code reusability within PL/SQL programs.
- PL/SQL Procedure
Procedures are similar to functions but do not return values. They are used to group a set of SQL and PL/SQL statements into a named block, enhancing code organization.
CREATE OR REPLACE PROCEDURE greet_employee(p_employee_name IN VARCHAR2) IS
BEGIN
DBMS_OUTPUT.PUT_LINE('Hello, ' || p_employee_name || '!');
END greet_employee;
/
- PL/SQL Package
A PL/SQL package is a logical grouping of related functions, procedures, variables, and other PL/SQL constructs. It provides a modular approach to code organization and management.
- PL/SQL Trigger
Triggers are special types of stored procedures that automatically execute in response to specific events, such as data modifications, in a specified table or view.
- PL/SQL Cursor
Cursors in PL/SQL provide a way to process a set of rows returned by a SQL query one at a time. They are crucial for iterating over query results within PL/SQL blocks.
DECLARE
CURSOR employee_cur IS
SELECT employee_id, employee_name FROM employees;
v_employee_id NUMBER;
v_employee_name VARCHAR2(50);
BEGIN
OPEN employee_cur;
LOOP
FETCH employee_cur INTO v_employee_id, v_employee_name;
EXIT WHEN employee_cur%NOTFOUND;
DBMS_OUTPUT.PUT_LINE('Employee ID: ' || v_employee_id || ', Employee Name: ' || v_employee_name);
END LOOP;
CLOSE employee_cur;
END;
/
- PL/SQL Exception Handling
Exception handling in PL/SQL involves the identification and management of errors during program execution. PL/SQL offers mechanisms like EXCEPTION blocks to gracefully handle errors and maintain program flow.
DECLARE
v_salary NUMBER := -5000;
BEGIN
IF v_salary < 0 THEN
-- Custom exception
RAISE_APPLICATION_ERROR(-20001, 'Salary cannot be negative.');
END IF;
EXCEPTION
WHEN OTHERS THEN
DBMS_OUTPUT.PUT_LINE('Error: ' || SQLCODE || ' - ' || SQLERRM);
END;
/
Advantages of PL/SQL
- Procedural Constructs: PL/SQL supports procedural constructs like conditional statements, loops, and exception handling, enhancing the expressiveness and flexibility of SQL.
- Modularity: The use of procedures, functions, and packages in PL/SQL promotes code modularity. Developers can encapsulate specific functionalities, making code easier to understand, maintain, and reuse.
- Performance: PL/SQL is frequently more efficient than SQL for specific operations because it can handle multiple SQL statements as a single block. This capability reduces the overhead of round trips between the application and the Database.
- Security: PL/SQL provides a layer of security by allowing developers to create stored procedures and functions. Access to tables can be restricted, and users may only interact with the data through these encapsulated components.
- Error Handling: PL/SQL offers robust error-handling mechanisms, enabling developers to capture and manage exceptions gracefully. This helps in identifying and resolving issues during runtime.
Disadvantages of PL/SQL
- Vendor Specificity: PL/SQL is primarily associated with Oracle databases. This vendor specificity may limit portability to other database systems, making applications tightly coupled with the Oracle ecosystem.
- Learning Curve: For developers accustomed to traditional SQL, the transition to PL/SQL may involve a learning curve. The procedural nature of PL/SQL introduces new concepts that need to be grasped.
- Resource Consumption: In certain scenarios, PL/SQL might consume more resources compared to SQL alone. This is particularly relevant for small-scale applications where the overhead of procedural constructs might outweigh the benefits.
- Debugging Challenges: Debugging PL/SQL code can be more challenging than debugging SQL queries. Comprehensive testing and debugging tools are essential to identify and resolve issues effectively.
- Limited Portability: Due to the vendor-specific nature of PL/SQL, applications developed using PL/SQL may face challenges when migrating to other database systems, restricting flexibility in choosing database platforms.