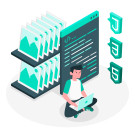
- 15th Nov 2023
- 23:37 pm
- Admin
What is Thread in Java
A thread is the smallest unit of execution within a Java program. Threads provide concurrent execution, allowing several processes to execute at the same time. They are lightweight instruction sequences that share the same process and memory space but run independently. Threads are instrumental in empowering Java for multitasking, concurrent user interactions, and efficient resource management, facilitating both concurrency and parallelism. Java provides robust support for multithreading, enabling the creation, management, and synchronization of threads. Threads can execute concurrently, improving performance and responsiveness in applications like as web servers, gaming, and scientific simulations.
Multithreading in Java
Multithreading is a crucial concept in Java that allows an application to run multiple threads simultaneously, handling multiple tasks at once. Java's intrinsic tools and features offer robust support for multithreading, making it a versatile language for creating responsive and efficient applications.
In Java, there are two techniques for producing threads:
- Adding to the Thread Class: A class that extends the 'Thread' class is one approach to generating threads in Java. Subclasses of 'Thread' override the 'run()' function to specify the code that will run simultaneously. The'start()' function is then used to create and start these custom thread classes.
- Utilizing the Runnable Interface: Implementing the 'Runnable' interface provides an alternative approach to creating threads. This technique separates the task's logic from the thread class, resulting in a more modular architectural design.
Multithreading in Java improves performance in programs that may benefit from parallelism. It is very useful in circumstances such as concurrent processing, parallel calculation, and developing dynamic user interfaces. Because Java supports multithreading, it is a flexible language for developing a broad range of applications, from basic utilities to sophisticated server systems.
Extending the Thread Class
One of the ways in Java for creating and managing threads is to extend the Thread class. By extending the built-in 'Thread' class and overriding its 'run()' function, you may construct bespoke thread classes. This is how it works:
- Designing a Custom Thread Class:
Using this method, you declare a new class that extends the 'Thread' class to create a bespoke thread. In the 'run()' function of this class, you give the precise logic that you want the thread to perform. When the thread is launched, this procedure includes the code that will execute simultaneously.
```
class MyThread extends Thread {
public void run() {
// Thread logic goes here
}
}
```
- Initiating and starting the Thread:
After you've established your own thread class, you may use it to generate instances in your application. To begin the thread's execution, use the thread object's'start()' function.
```
public class Main {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
thread1.start(); // This initiates the concurrent execution of the thread
}
}
```
- Concurrency and Parallelism:
Creating and managing threads that operate concurrently with other segments of your program can be achieved by extending the `Thread` class. This method is especially advantageous when striving for concurrent execution and parallelism, enabling the simultaneous execution of multiple tasks.
Utilizing the `Thread` class for thread creation in Java is common, but an alternative method using the `Runnable` interface is also available. Some developers favor the `Runnable` implementation because it separates task logic from the thread class, offering a more modular and reusable design. The decision between these two methods relies on your application's needs and your preferences in design.
Implementing the Runnable Interface
Another approach in Java for establishing and maintaining threads is to implement the 'Runnable' interface. This method isolates the job logic from the thread class, allowing for a more modular and adaptable architecture. This is how it works:
- Creating a Runnable-Implementing Class:
You design a class that implements the 'Runnable' interface to build a custom thread using this technique. This class must implement the 'run()' function, which includes the precise code that the thread will run.
```
class MyTask implements Runnable {
public void run() {
// Task logic goes here
}
}
```
- Creating a Thread Object:
To execute the task outlined in the 'MyTask' class concurrently, you can create a 'Thread' object and assign it an instance of 'MyTask'. The constructors in the 'Thread' class accept a 'Runnable' object as a parameter.
```
public class Main {
public static void main(String[] args) {
MyTask task = new MyTask();
Thread thread = new Thread(task);
thread.start(); // This initiates the concurrent execution of the thread
}
}
```
- Concurrency and Parallelism:
Concurrency and parallelism may be achieved in Java programs by implementing the 'Runnable' interface. It decouples the work at hand from thread management, resulting in a more modular and manageable approach. You can run many instances of 'MyTask' concurrently by assigning them to distinct threads.
- Flexibility and Reusability:
One advantage of introducing 'Runnable' is that it fosters worry separation. The same 'Runnable' instance can be utilized in numerous threads, increasing its flexibility and reusability.
When you have numerous tasks to run concurrently, implementing the 'Runnable' interface allows you to handle threads in a clearer and more structured manner. Because of its flexibility and superior adherence to object-oriented programming concepts, this method is frequently used in Java multithreading.
Advantages of multithreading in Java
Multithreading in Java has various advantages, including the ability to execute multiple tasks concurrently inside a single application. Among its many advantages are:
- Improved Performance and Responsiveness: Multithreading allows applications to do numerous activities at the same time, improving performance by effectively using available CPU resources and keeping the system responsive.
- Improved Resource usage: Using multiple threads enables for improved resource usage since it efficiently uses available CPU cores, boosting the system's capability.
- Effective Handling of I/O Operations: Threads aid in the management of I/O-bound operations by letting the software to do other tasks while waiting for input/output, hence increasing system throughput.
- Concurrent Task Execution: Multithreading enables multiple tasks to run concurrently, enhancing parallel processing and significantly expediting task completion.
- Better User Experience: Applications employing multithreading offer a more responsive and engaging user experience by enabling concurrent user input, background operations, and simultaneous interface updates.
- Resource Sharing and Optimization: Threads provide for effective resource sharing and synchronization, promoting cooperation and coordination between processes inside a program.
- Scalability and Efficiency: Multithreading adds to application scalability by enabling the development of more complex, high-performance systems and improving overall computing efficiency.
- Asynchronous Operations: Threads enable asynchronous programming paradigms by permitting non-blocking execution and promoting efficient management of background and long-running processes.
Multithreading in Java allows you to conduct concurrent and separate operations, which improves system efficiency, resource consumption, and overall responsiveness in applications.
Example of Multithreading in Java
A Java program that demonstrates multithreading using two threads to perform concurrent tasks:
```
class MyThread extends Thread {
public void run() {
for (int i = 1; i <= 5; i++) {
System.out.println(Thread.currentThread().getName() + ": " + i);
try {
Thread.sleep(500); // Sleep for 500 milliseconds
} catch (InterruptedException e) {
System.out.println(e);
}
}
}
}
public class MultiThreadExample {
public static void main(String[] args) {
MyThread thread1 = new MyThread(); // Thread 1
MyThread thread2 = new MyThread(); // Thread 2
thread1.setName("Thread 1");
thread2.setName("Thread 2");
thread1.start(); // Start Thread 1
thread2.start(); // Start Thread 2
}
}
```
Explanation:
- The program defines the 'MyThread' class, which extends the 'Thread' class. The 'run()' function is altered to define the tasks that each thread will undertake.
- Within the 'run()' method, each thread counts from 1 to 5, showing its thread name and current count while waiting for 500 milliseconds between counts with 'Thread.sleep()'.
- Two instances of 'MyThread' are generated in the'main' function to represent two threads - 'thread1' and 'thread2'.
- To differentiate these threads in the output, their names are set using the'setName()' function.
- The function'start()' is called on both threads to start their execution concurrently.
This application shows how to generate and execute several threads in Java at the same time. The threads operate independently, concurrently doing tasks as stated by the 'run()' function, demonstrating the fundamental notion of multithreading.