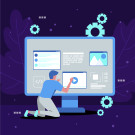
- 15th Nov 2023
- 14:55 pm
Java I/O (Input/Output) stands as a vital cornerstone in the Java programming language, offering a broad spectrum of classes and methods meticulously designed for the orchestration of input and output operations. In the heart of the 'java.io' package resides an extensive assembly of classes, meticulously crafted to address a spectrum of needs. These encompass vital aspects such as input streams, output streams, readers, writers, file management, byte-oriented I/O, and character-based I/O. This collective ensemble serves as the foundation for an array of file operations, diverse data transfer mechanisms, and the nuanced orchestration of stream-based input/output functionalities within Java programs.
This intricate toolkit, thoughtfully curated and encapsulated within the 'java.io' package, emerges as an indispensable component of Java's I/O capabilities. It bequeaths developers with the adaptability and precision necessary for the adept management of a myriad of input and output scenarios that their applications may encounter.
The Java I/O library facilitates file handling through diverse methods, enabling the creation, reading, writing, and manipulation of files and directories. It accommodates both binary and character-based data transfer, ensuring efficient processing of different data types.
Moreover, Java I/O offers a versatile and standardized approach to executing input and output operations, boasting platform independence and supporting seamless data transmission across systems. While the 'java.io' package furnishes fundamental I/O capabilities, the 'java.nio' package introduces advanced features under Java's NIO (New I/O). This includes non-blocking I/O and extended file handling capabilities, enhancing speed and scalability. In essence, Java I/O stands as a pivotal element in the Java programming language, equipping developers with a sophisticated toolkit for managing diverse input/output processes in their applications.
Standard I/O streams in Java
Java requires standard I/O streams to manage input and output operations. These streams allow for terminal interaction, file reading and writing, and communication with external devices or network connections. There are three standard I/O streams in Java:
- System.in (Standard Input): The standard input stream, denoted by 'System.in,' is generally associated with the keyboard.
It allows you to read data entered by the user or data piped in from another source into your Java program.
Sample Usage:
```
import java.util.Scanner;
public class StandardInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = scanner.nextInt();
System.out.println("You entered: " + number);
}
}
```
- System Output (System.out): 'System.out' is the standard output stream that is often attached to a console or terminal.
It is used to display information, messages, or results to the user.
Sample Usage:
```
public class StandardOutputExample {
public static void main(String[] args) {
System.out.println("Hello, Java Standard Output!");
}
}
```
- System.err (Standard Error): 'System.err' represents the standard error stream, which is the same as 'System.out' but is often used for error messages or uncommon conditions.
It is also tied to a terminal or console.
Sample Usage:
```
public class StandardErrorExample {
public static void main(String[] args) {
System.err.println("This is an error message.");
}
}
```
These standard I/O streams are part of Java's 'System' class and are important tools for input and output activities, allowing Java programs to interface with users, display information, and manage problems.
Types of streams in Java I/O
Streams in Java represent a data sequence. Streams are classified into two types based on the sort of data they carry: byte streams and character streams.
In Java, byte streams are an important part of the I/O system, processing raw binary data in the form of bytes. When working with binary data, these streams make byte-level reading and writing operations easier.
- InputStream and OutputStream:
InputStream: The superclass of all classes that describe a bytes input stream is InputStream. It serves as a base class for reading bytes from a source.
Methods such as'read(),'read(byte[])', and'skip()' are used to read bytes from the stream.
OutputStream: The superclass of all classes that describe a bytes output stream is OutputStream. It is the starting point for writing bytes to a destination.
To write bytes to the stream, methods such as 'write()', 'write(byte[])', and 'flush()' is used.
Examples of Byte Streams:
The 'FileInputStream' and 'FileOutputStream' classes are commonly used for byte-level file reading and writing.
You can read from and write to byte arrays in memory using the 'ByteArrayInputStream' and 'ByteArrayOutputStream' methods.
In Java, byte streams demonstrate proficiency in managing low-level I/O operations that revolve around bytes. Their versatility encompasses tasks like reading binary files, establishing network connections, and interfacing with hardware devices. These streams excel in applications that demand direct processing of binary data, operating efficiently without the need for character encoding or decoding.
- Character Streams:
Character streams in Java offer a more abstract approach compared to byte streams. They specialize in managing character-based data, specifically text, in the Unicode character format. These streams are tailored for tasks involving the reading and writing of textual information, along with facilitating operations at the character level.
Reader and Author: Reader: An abstract class that is the superclass of all character input stream classes. It is the starting point for reading characters from a source.
To read characters from the stream, methods such as'read()','read(char[])', and'skip()' are used.
Writer: Similarly, an abstract class is the superclass of all classes that describe a character output stream. It serves as a gateway for inscribing characters onto a destination. Methods like 'write()', 'write(char[])', and 'flush()' are employed to transcribe characters onto the stream.
Character Stream Examples: Notable instances include 'FileReader' and 'FileWriter', extensively utilized for character-level reading from and writing to text files. Introducing buffering for efficient character handling, 'BufferedReader' and 'BufferedWriter' stand out in the realm of character streams.
Character streams prove invaluable when dealing with text-based data entailing character encoding and decoding. Leveraging Unicode for platform-independent character data management, Java programs can seamlessly read and write characters in various encodings such as UTF-8 or UTF-16. For meticulous handling of text-based I/O operations, character streams ensure the correct treatment of multiple languages and character sets.
Uses of I/O stream in Java Programming
I/O streams in Java are critical for many jobs in Java programming because they provide a flexible approach to organizing input and output operations. Their principal applications include:
- File Handling: Reading and Writing Files: Streams let you to create, alter, and retrieve data by reading from and writing to files.
- User input and output: Console Interaction: Streams allow programs to interface with users by reading input from the console (keyboard) and displaying output to the console.
- Network Communication: Socket Operations: Streams facilitate data flow over networks by allowing programs to communicate over network connections.
- Object Serialization: Streams are used to serialize objects into byte streams and deserialize objects from the byte streams.
- Data Manipulation: Data Transformation: Streams provide data manipulation and conversion, allowing for the processing and transformation of various data formats.
- Buffering and Performance Improvement: Buffering enhances performance by reducing the amount of I/O operations and enhancing efficiency.
- Handling many Data Types: Streams give methods for dealing with many data types, such as bytes, characters, primitive data types, and objects.
Overall, I/O streams offer a foundation for handling data input and output from numerous sources, enabling Java programs to communicate with people, read and write files, transport data over networks, and handle a variety of data kinds effectively and fast.