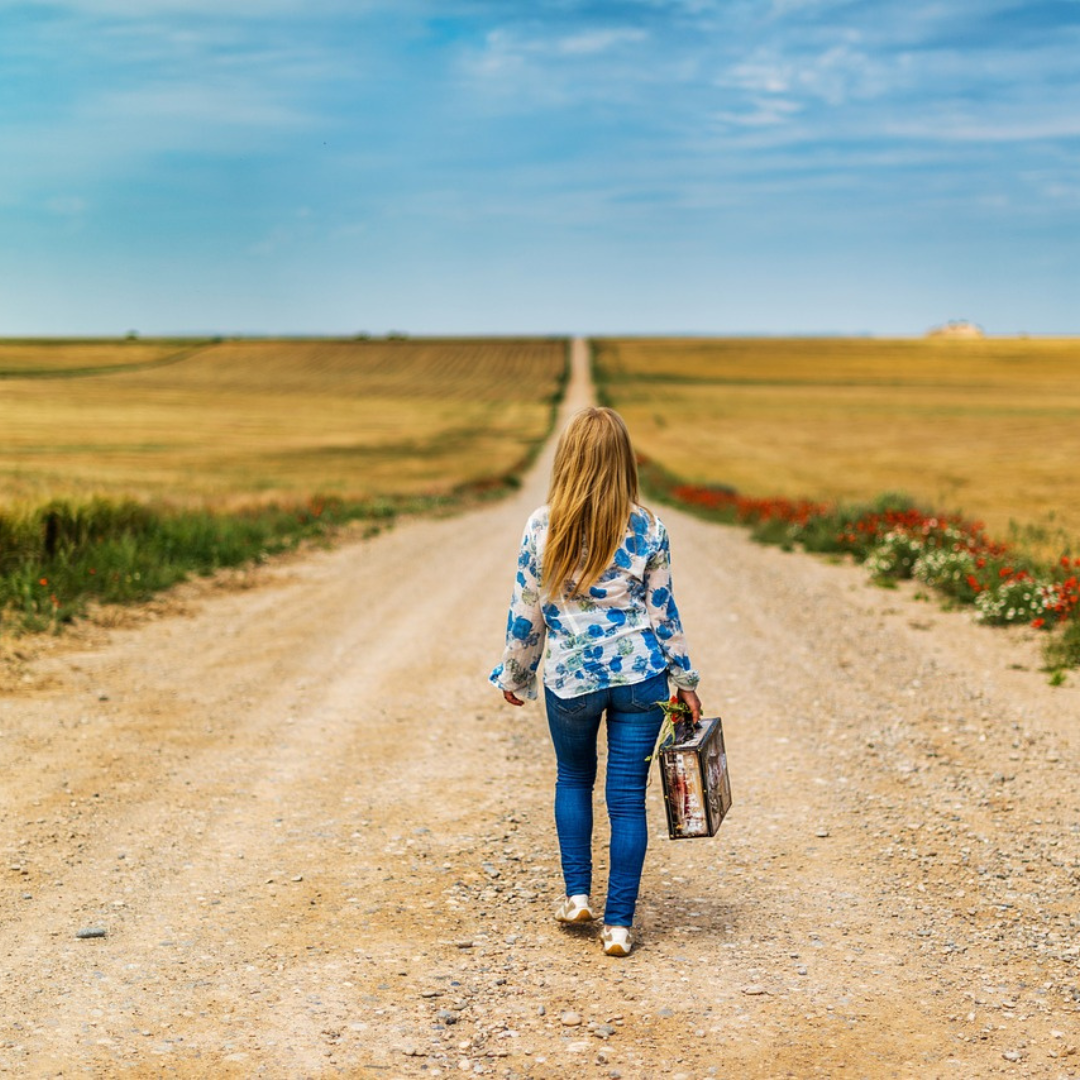
- 3rd Apr 2023
- 09:10 am
Java Homework Question
Distance Between Two Points using Math Class- - Write an application that reads the (x,y) coordinates for two points. Compute the distance between the two points using a formula
Java Homework Solution
Here's the Java code for the app that reads the (x,y) coordinates for two points and computes the distance between them using the Math class:
import java.util.Scanner;
public class DistanceBetweenPoints {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Read the coordinates for the first point
System.out.print("Enter the x-coordinate of the first point: ");
double x1 = input.nextDouble();
System.out.print("Enter the y-coordinate of the first point: ");
double y1 = input.nextDouble();
// Read the coordinates for the second point
System.out.print("Enter the x-coordinate of the second point: ");
double x2 = input.nextDouble();
System.out.print("Enter the y-coordinate of the second point: ");
double y2 = input.nextDouble();
// Calculate the distance between the two points using the formula
double distance = Math.sqrt(Math.pow(x2 - x1, 2) + Math.pow(y2 - y1, 2));
// Print the distance between the two points
System.out.printf("The distance between the two points is %.2f\n", distance);
input.close();
}
}
When you run this code and enter the coordinates for the two points, the program calculates the distance between them using the Math class and prints it to two decimal places. For example:
Enter the x-coordinate of the first point: 3
Enter the y-coordinate of the first point: 4
Enter the x-coordinate of the second point: 6
Enter the y-coordinate of the second point: 8
The distance between the two points is 5.00
Note that we used the Math.pow() method to calculate the power of a number and the Math.sqrt() method to calculate the square root of a number.