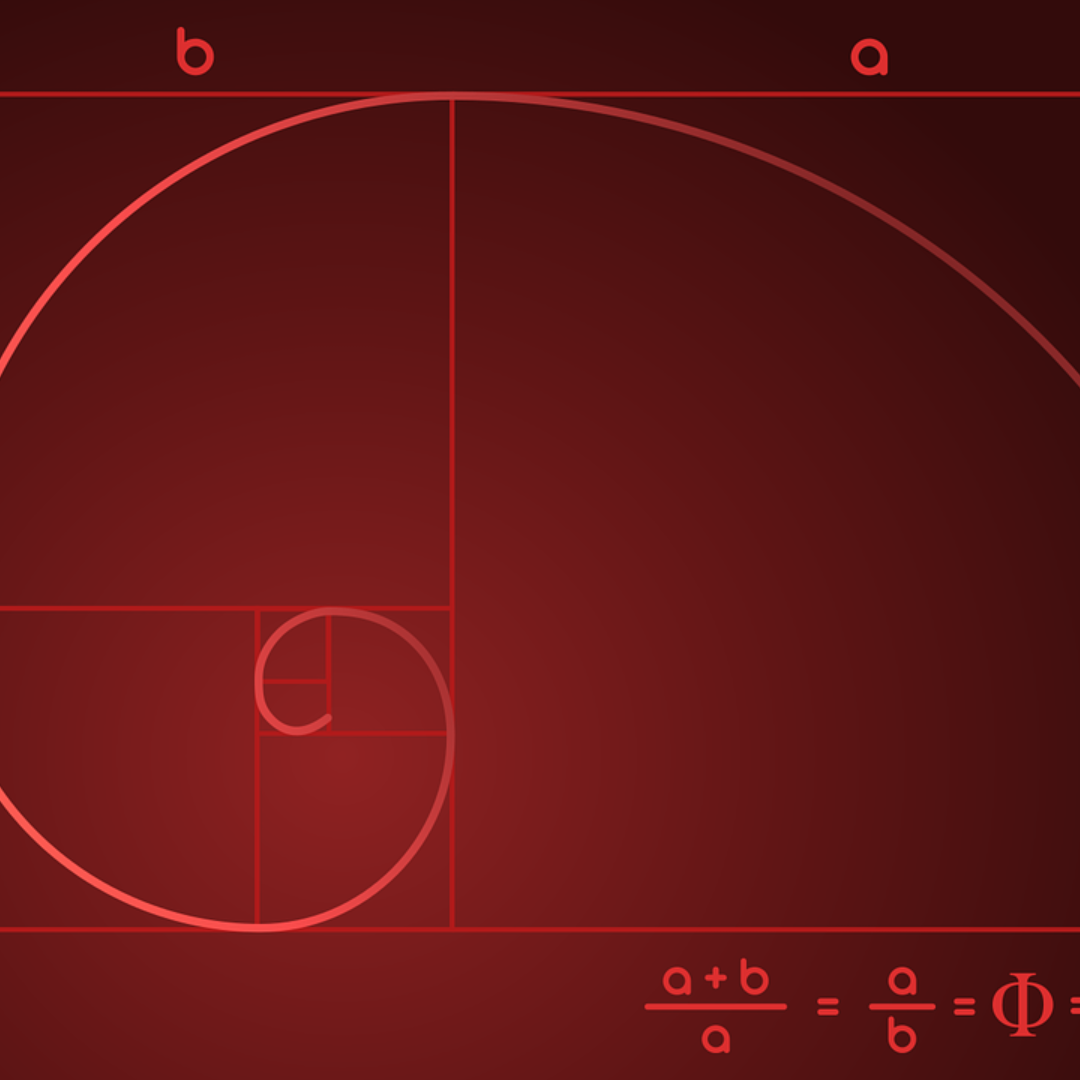
- 6th Apr 2023
- 09:16 am
C# Homework Question - C# Logical Puzzles, Games, and Algorithms: Fibonacci
C# Homework Solution
Here's an example C# program for generating Fibonacci sequence up to a specified limit using recursion:
using System;
public class Program
{
public static void Main()
{
int limit = 20; // Set the upper limit for the sequence
for (int i = 0; i < limit; i++)
{
Console.Write(Fibonacci(i) + " ");
}
}
public static int Fibonacci(int n)
{
if (n <= 1)
{
return n;
}
else
{
return Fibonacci(n - 1) + Fibonacci(n - 2);
}
}
}
This program uses a recursive function called Fibonacci to generate the Fibonacci sequence up to the specified limit. The Main method sets the upper limit for the sequence and loops through the sequence, printing each number. The Fibonacci method takes an integer n as input and recursively computes the n-th number in the Fibonacci sequence by adding the two previous numbers together. The base cases of the recursion are n=0 and n=1, which return 0 and 1, respectively.