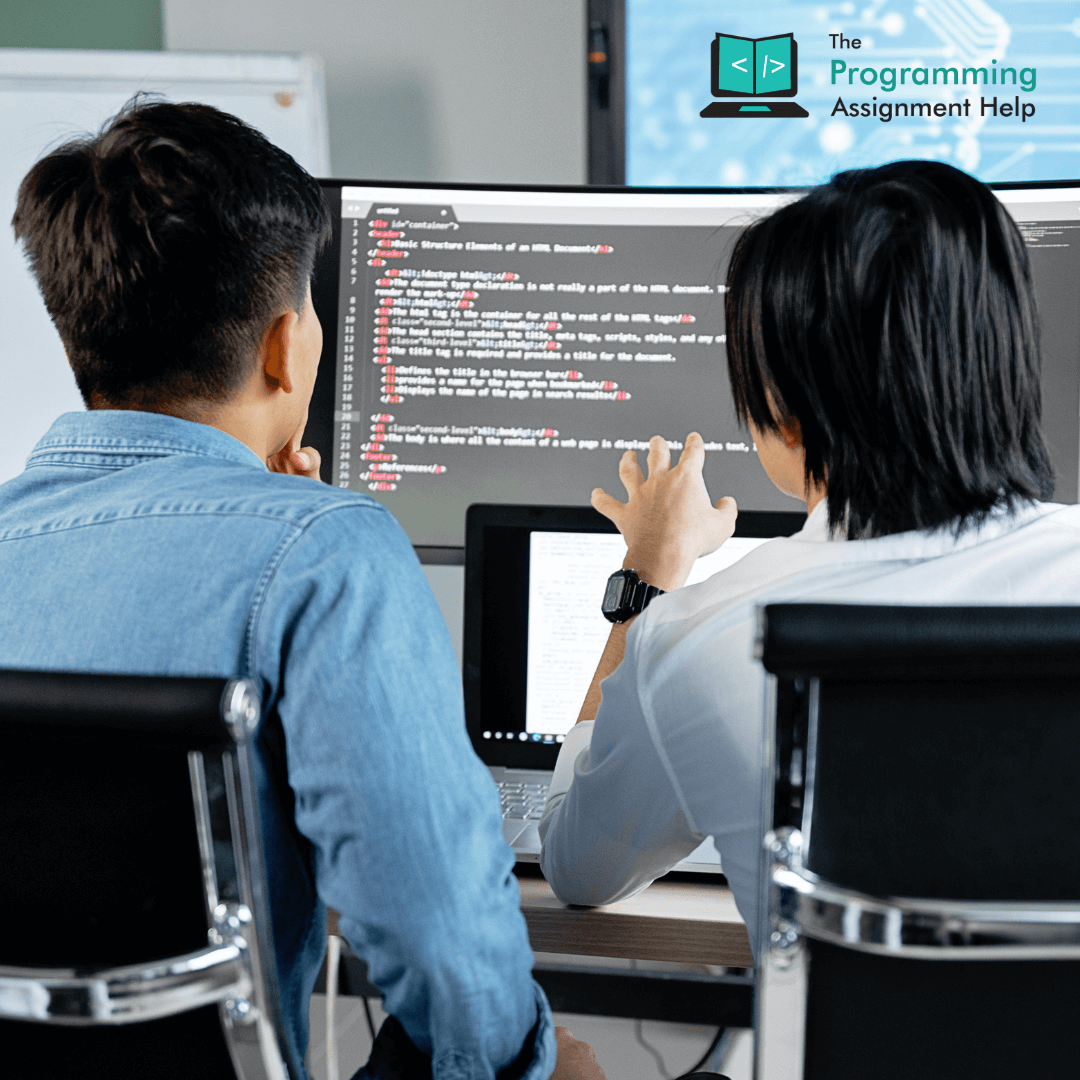
- 2nd Nov 2021
- 03:37 am
- Adan Salman
Below is a C++ program that demonstrates writing and reading classes into a file using serialization and deserialization. The program defines a class called Student
that stores information about a student, such as name, roll number, and marks. The program uses serialization to write the object of the Student
class into a file and then reads and deserializes the object from the file.
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
public:
Student() : rollNumber(0), marks(0) {}
Student(int roll, const string& name, double marks)
: rollNumber(roll), name(name), marks(marks) {}
int getRollNumber() const { return rollNumber; }
string getName() const { return name; }
double getMarks() const { return marks; }
void setRollNumber(int roll) { rollNumber = roll; }
void setName(const string& n) { name = n; }
void setMarks(double m) { marks = m; }
private:
int rollNumber;
string name;
double marks;
};
int main() {
// Writing a Student object into a file
Student student(101, "John Doe", 85.5);
ofstream outFile("student.txt", ios::binary);
if (outFile) {
outFile.write(reinterpret_cast<const char*>(&student), sizeof(Student));
outFile.close();
cout << "Student data written to the file.\n";
} else {
cout << "Error opening the file for writing.\n";
}
// Reading and deserializing a Student object from the file
Student readStudent;
ifstream inFile("student.txt", ios::binary);
if (inFile) {
inFile.read(reinterpret_cast<char*>(&readStudent), sizeof(Student));
inFile.close();
cout << "Student data read from the file.\n";
cout << "Roll Number: " << readStudent.getRollNumber() << endl;
cout << "Name: " << readStudent.getName() << endl;
cout << "Marks: " << readStudent.getMarks() << endl;
} else {
cout << "Error opening the file for reading.\n";
}
return 0;
}
Our Programming Exam Helper's take on this Solution
In this program, the Student
class is used to represent a student's information. It has private data members for the roll number, name, and marks. It also provides public member functions to access and modify these data members.
In the main
function, a Student
object is created with some sample data. It is then written into the file "student.txt" using serialization. Serialization involves converting the binary representation of the Student
object into a series of bytes and writing it to the file.
Next, the program reads and deserializes the Student
object from the same file "student.txt". The data is read from the file, and the object is reconstructed using the deserialized data. The student's information is then displayed on the console.
Please note that this program uses a binary file to store the serialized data. If you want to store the data in a human-readable format, you can use other serialization methods like JSON or XML. Also, in a real application, error handling and proper file handling should be implemented to ensure the file operations are successful.