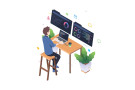
- 17th Nov 2023
- 21:37 pm
- Admin
C++ Programming Structures and Unions empower programmers to organize diverse data types within a single entity. Structures allow bundling variables of various types, facilitating data organization, easy access, and flexibility. On the other hand, unions optimize memory usage by sharing memory among members, making them more memory-efficient but requiring careful use to avoid data overwrite. The choice between structures and unions depends on specific program requirements.
What is a Structure?
In C++ programming, a structure is a user-defined data type designed for bundling variables of different data types under a unified name. It enables the creation of a composite data type, resembling a record, where each member holds distinct information. Structures enhance code organization by grouping related variables together, promoting readability and maintainability. With a clear syntax and the ability to represent complex entities, structures provide a valuable tool for programmers to design and manage diverse data within a single, cohesive entity.
Syntax of Structure
In C++, creating a structure involves specifying a novel data type that consolidates variables of diverse data types using a single name. The structure declaration begins with the keyword 'struct,' followed by the structure name and a block containing individual members.
Each member has its own data type and name. This syntax allows the creation of a cohesive entity that can hold and organize various data elements within a program.
struct StructureName {
datatype1 member1;
datatype2 member2;
// ... more members
};
What is a Union?
A union in C++, is a custom data type that allows the grouping of variables with distinct data types within a common memory space. Unlike structures, unions allocate memory only large enough to accommodate their largest member, promoting efficient memory usage.
Unions are particularly useful when only one member needs to be active at a given time. This allows programmers to conserve memory by sharing resources, making unions a valuable tool for optimizing space in scenarios where variable interchangeability is necessary.
Syntax of Union
In C++ programming, a union is defined using the 'union' keyword, followed by the union name and a block containing various members. Each member within the union can have different data types. The memory allocation for a union in C++ is determined by the size of its largest member, ensuring optimal use of space.
union UnionName {
datatype1 member1;
datatype2 member2;
// ... more members
};
Advantages of Structure
Structures contribute to the creation of well-organized and readable code structures in C++ programs.
Here are some advantages of structures in C++:
- Data Organization: Structures enable the grouping of variables with different data types under a single, meaningful name.
- Readability: The organized structure enhances code readability, making it easier for programmers to understand and maintain.
- Easy Access: Members within a structure can be accessed using the dot notation, simplifying data manipulation.
- Flexibility: In C++, structures allow the grouping of variables of different types, providing a flexible way to represent real-world entities.
Disadvantages of Structure
While structures in C++ offer various benefits, they come with certain drawbacks.
Here are some disadvantages of structures in C++:
- Higher Memory Consumption: Each member of a structure requires its own memory space, potentially leading to increased memory usage.
- No Default Initialization: Unlike arrays, structures lack a default way of initializing their members.
- Less Convenient Initialization: Structures do not provide default initialization methods, making them less convenient to work within certain situations.
Advantages of Union
The advantages of unions in C++ are significant.
Here are some advantages of unions in C++:
- Memory Efficiency: Unions allocate memory only large enough to hold their largest member, promoting efficient memory utilization.
- Single Allocation: As all members share the same memory space, unions can be more memory-efficient when only one member is utilized at a time.
- Space Optimization: Unions are suitable for scenarios where variable interchangeability is necessary, allowing programmers to conserve memory by sharing resources.
Disadvantages of Union
While unions in C++ provide memory efficiency, they come with potential drawbacks.
Here are some disadvantages of unions in C++:
- Data Overwrite Risk: Modifications to one member of a union can unintentionally impact the values of other members.
- Limited Use Cases: Unions are most suitable for scenarios where only one member is actively used at a time, limiting their applicability.
- Complexity: The potential for data overwrite and the need for careful usage introduces complexity, requiring developers to be cautious in their implementation.
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.