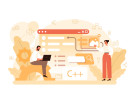
- 16th Nov 2023
- 22:25 pm
C++ operators are fundamental tools used to perform operations on data. They enable a wide range of computations and manipulations on variables, constants, and expressions. These operators fall into different categories, including arithmetic, assignment, relational, logical, bitwise, and conditional operators.
Each category serves specific purposes, allowing programmers to execute mathematical calculations, comparison operations, logical evaluations, and bitwise manipulations within their programs. Understanding and utilizing these operators effectively is essential for proficient programming in C++, providing the means to perform diverse tasks efficiently and accurately.
Types of C++ Operators
In C++, operators are classified into distinct types, each serving specific purposes. These types encompass a range of functionalities:
- Arithmetic Operators: Handle mathematical calculations, including unary and binary operations.
- Assignment Operators: Assign values to variables and execute operations simultaneously.
- Relational Operators: Compare relationships between operands to determine equality or inequality.
- Logical Operators: Evaluate logical conditions, commonly used in decision-making statements.
- Bitwise Operators: Perform operations at the bit level within variables.
- Ternary or Conditional Operators: Provide a concise way to handle conditional statements using three operands for decision-making in code.
C++ Arithmetic Operators
C++ arithmetic operators are vital in performing mathematical computations. They are categorized as unary, acting on a single operand, and binary, functioning with two operands, and offering various operations.
1. Unary Operators
Unary operators work with a single operand, modifying its value.
- Increment (++) and Decrement (--): Increase or decrease the value of an operand by one, respectively.
- Unary Plus (+) and Unary Minus (-): Alter the sign of the operand.
2. Binary Operators
Binary operators function with two operands.
- Addition (+) and Subtraction (-): Perform addition and subtraction operations.
- Multiplication (*) and Division (/): Execute multiplication and division operations.
- Modulus (%): Provides the remainder of a division operation.
#include
using namespace std;
int main() {
int a = 10, b = 4;
int addition = a + b;
int subtraction = a - b;
int multiplication = a * b;
int division = a / b;
int modulus = a % b;
cout << "Addition: " << addition << endl;
cout << "Subtraction: " << subtraction << endl;
cout << "Multiplication: " << multiplication << endl;
cout << "Division: " << division << endl;
cout << "Modulus: " << modulus << endl;
return 0;
}
C++ Assignment Operators
C/C++ assignment operators serve the dual purpose of assigning values to variables and performing operations in a concise manner.
Here are the C++ assignment operators section:
- Functionality: C++ assignment operators perform dual functions: assigning values to variables and performing operations simultaneously.
- Basic Assignment Operator (=): This operator assigns the value on the right-hand side to the variable on the left-hand side.
- Compound Assignment Operators: Combine operations with assignments in a single step, enhancing code readability and compactness.
- Examples: Include '+=', '-=', '*=', '/=', and '%=', used for addition, subtraction, multiplication, division, and modulus operations, respectively.
- Concise Operations: Simplify operations by executing an arithmetic operation and directly assigning the result to the variable.
#include
using namespace std;
int main() {
int a = 5;
a += 3; // Equivalent to a = a + 3
a -= 2; // Equivalent to a = a - 2
a *= 4; // Equivalent to a = a * 4
a /= 2; // Equivalent to a = a / 2
a %= 3; // Equivalent to a = a % 3
cout << "Value of a: " << a << endl;
return 0;
}
C++ Relational Operators
C/C++ relational operators are crucial for making comparisons between two operands, assessing their relationship, and determining conditions of equality or inequality.
Here are the points regarding C++ relational operators presented in bullet format:
- Purpose: Relational operators in C++ facilitate comparisons between two operands, determining their relationship.
- Operators: Include less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), equality (==), and inequality (!=).
- Usage: Integral in conditional statements and loops to establish logical conditions for controlling program flow.
- Function: Evaluate the truth value of expressions to guide the execution of specific code segments based on the relationship between operands.
- Critical Role: Integral in decision-making and conditional constructs, enabling programmers to define conditions that dictate the execution of code based on the comparison results.
#include
using namespace std;
int main() {
int x = 5, y = 10;
if (x > y) {
cout << "x is greater than y" << endl;
} else {
cout << "x is less than or equal to y" << endl;
}
return 0;
}
C++ Logical Operators
C++ logical operators are pivotal for evaluating logical conditions and determining the truth value of expressions. Logical operators are extensively utilized in decision-making structures, including conditional statements and loops, to manage the program's flow based on the logical assessment of operands.
Key points about C++ logical operators:
- Purpose: C++ logical operators, such as && (logical AND), || (logical OR), and ! (logical NOT), evaluate logical conditions.
- Decision-Making: Used in decision-making constructs to establish conditions that guide the program's execution.
- Logical AND (&&) and Logical OR (||): Combine conditions and evaluate to either true or false based on specific criteria.
- Logical NOT (!): Reverses the logical state of its operand, converting true to false and vice versa.
- Integral Role: Essential for creating logical conditions that control program flow based on the evaluation of logical expressions.
#include
using namespace std;
int main() {
int p = 5, q = 7;
if (p > 3 && q < 10) {
cout << "Both conditions are true" << endl;
}
if (p > 3 || q > 10) {
cout << "At least one condition is true" << endl;
}
if (!(p > 3)) {
cout << "The logical NOT operator reverses the result" << endl;
}
return 0;
}
C++ Bitwise Operators
C++ bitwise operators perform operations at the binary level, manipulating individual bits of operands. These operators are fundamental in low-level programming and device driver development.
Here are the points organized in bullet format:
- Bitwise Operations: Manipulate individual bits within variables using operators like &, |, ^, ~, <<, and >>.
- Operators Used: Bitwise AND (&), Bitwise OR (|), Bitwise XOR (^), Bitwise NOT (~), Left Shift (<<), and Right Shift (>>).
- Binary Level Manipulation: Perform operations at the bit level, altering the binary representation of numbers.
- Low-Level Programming: Essential in low-level programming tasks, including embedded systems, device drivers, and network protocols.
- Bitwise Shift Operations: Include left (<<) and right (>>) shifts, moving bits to the left or right within binary representations, effectively multiplying or dividing by powers of two, respectively.
#include
using namespace std;
int main() {
int a = 5, b = 9;
cout << "Bitwise AND: " << (a & b) << endl;
cout << "Bitwise OR: " << (a | b) << endl;
cout << "Bitwise XOR: " << (a ^ b) << endl;
cout << "Bitwise NOT: " << (~a) << endl;
cout << "Left Shift: " << (a << 1) << endl;
cout << "Right Shift: " << (b >> 1) << endl;
return 0;
}
C++ Ternary or Conditional Operators
C++ features a unique conditional operator known as the ternary operator, denoted by the symbols '?' and ':'. It's a concise way to express conditional statements involving three operands.
Here's the information organized into bullet points:
- Ternary Operator Syntax: Comprises a conditional expression followed by a question mark ('?'), a true value, a colon (':'), and a false value.
- Conditional Decision-Making: Evaluates the condition, returning one of the two values based on whether the condition is true or false.
- Example: Syntax - (condition) ? true_value : false_value
- Compactness and Readability: Provides a concise way to handle conditional expressions.
- Common Use: Often used for quick decision-making within expressions, especially when assigning values to variables based on a condition's truth value.
#include
using namespace std;
int main() {
int x = 5, y = 10;
int result = (x > y) ? x : y;
cout << "Result: " << result << endl;
return 0;
}