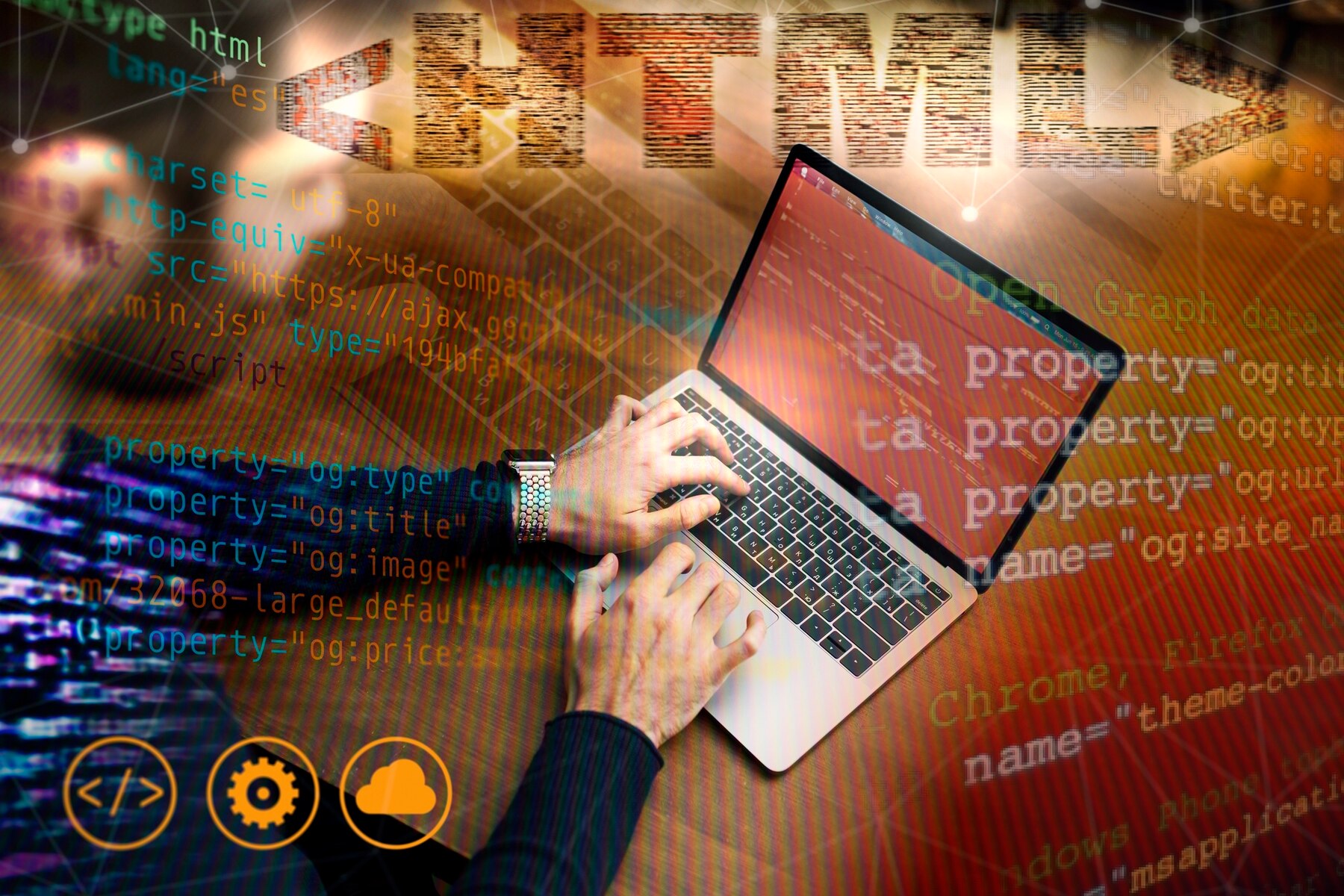
- 27th Dec 2023
- 15:00 pm
- Admin
In Python programming, gaining clarity on the distinctions between arrays and lists is crucial for crafting efficient and purpose-driven code. Arrays and lists, both fundamental data structures, possess unique features tailored to diverse requirements.
What is an Array in Python?
In Python, an array is a core data structure explicitly crafted to store elements of the same data type in a sequential and contiguous manner. In contrast to lists, which can accommodate diverse data types, arrays adhere to a strict uniformity, rendering them highly efficient for tasks that demand homogeneous data representation. The Python array module offers a dedicated 'array' type for creating and managing arrays, optimizing memory usage, and enabling quick access to elements.
Arrays prove particularly advantageous in situations involving numerical computations and large datasets. They leverage contiguous memory locations, resulting in faster and more streamlined operations. This characteristic not only improves performance but also contributes to a reduced memory footprint. In essence, arrays in Python function as specialized tools for tasks that prioritize data homogeneity and efficient memory utilization. Having a grasp of the characteristics of arrays is vital for making well-informed decisions when selecting the most suitable data structure for particular programming challenges.
What is a List in Python?
Lists offer a dynamic environment that facilitates easy modification, insertion, and deletion of elements, making them suitable for situations where data requirements may change during program execution. Their adaptability and simplicity make lists the favored choice for various programming tasks, including iterating through elements, implementing stacks and queues, and managing data structures with varying lengths and content. Understanding the characteristics of lists is fundamental for leveraging their flexibility and optimizing their use in Python programming.
Lists create a dynamic environment that facilitates effortless modification, insertion, and deletion of elements. This adaptability makes them well-suited for scenarios where data requirements may evolve during program execution. The versatility and straightforwardness of lists position them as the preferred choice for numerous programming tasks, such as iterating through elements, implementing stacks and queues, and managing data structures with varying lengths and content. Grasping the characteristics of lists is essential for harnessing their flexibility and optimizing their utilization in Python programming.
Array vs List in Python
Memory Efficiency:
- Arrays in Python are memory-efficient because they store elements of the same data type in sequential memory locations. This reduces the memory footprint, making arrays a better choice for handling large datasets.
- Lists, being dynamic and accommodating different data types, may require more memory due to the additional overhead of storing type information for each element.
Performance:
- Arrays generally offer better performance for numerical operations. Since elements are stored in contiguous memory, accessing and manipulating them is faster.
- Lists, while versatile, may incur a performance cost, especially when dealing with a large number of elements or extensive manipulations.
Type Constraint:
- Arrays enforce a strict data type constraint, allowing only elements of the same type. This constraint can be advantageous for tasks that require uniformity in data representation.
- Lists, being more flexible, allow for the storage of elements with different data types in the same list. This flexibility comes at the cost of potential type-related issues.
Flexibility and Functionality:
- Arrays, by design, are more specialized and rigid in terms of data type, providing efficiency in memory usage and numerical operations. However, this rigidity can limit their general-purpose functionality.
- Lists, due to their flexibility, provide a wider array of built-in functions and methods. This versatility makes lists well-suited for various tasks, ranging from straightforward data storage to intricate data manipulation and processing.
Ease of Use and Syntax:
- Arrays often require additional steps and syntax for common operations, given their specialization and focus on numerical data. This may lead to more complex code for tasks that are comparatively straightforward with lists.
- Lists, with their intuitive syntax and extensive built-in functions, are generally easier to work with, especially for tasks that involve diverse data types or require frequent modifications. The simplicity of list operations contributes to improved code readability and maintainability.
Examples of List and Array
List Example:
my_list = [1, 2, 'three', 4.5, True]
print(my_list)
Array Example:
from array import array my_array = array('i', [1, 2, 3, 4, 5])
print(my_array)
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.