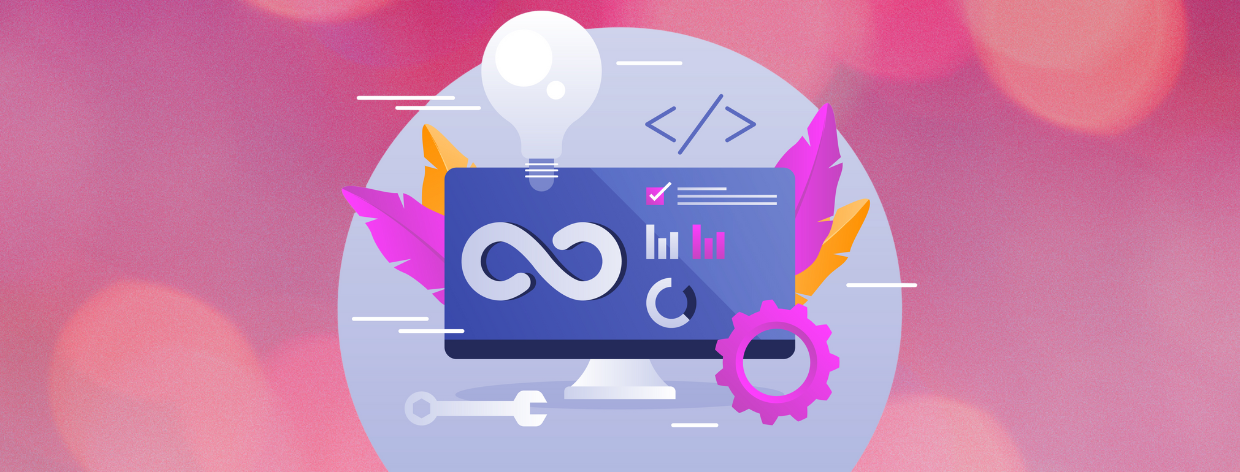
- 21st Feb 2024
- 21:52 pm
- Admin
The concept of infinity, stretching endlessly beyond our grasp, has captivated mathematicians and philosophers for centuries. But what about in the digital realm? In Python, the world of computers, does infinity exist? And if so, how do we deal with it?
While true mathematical infinity is impossible to represent on a computer due to its finite memory and processing power, Python offers ways to handle conceptual infinity. This "computational infinity" comes in two flavors: positive infinity (represented by float('inf')) and negative infinity (represented by float('-inf')). These values act as stand-ins for limitless magnitudes, far exceeding any number your computer can store.
Understanding infinity in Python is crucial for various reasons. It allows us to:
- Handle division by zero gracefully: Instead of crashing the program, we can use infinity to represent the undefined result, preventing errors and ensuring smooth program flow.
- Represent extremely large or small values: Beyond the limitations of regular numbers, infinity lets us model real-world phenomena with vast ranges, like the age of the universe or the size of subatomic particles.
- Optimize algorithms: In iterative algorithms like gradient descent, strategically using infinity can guide the search process towards an optimal solution.
- Analyze data effectively: Identifying missing values or outliers in datasets often involves using infinity as a placeholder, helping us understand the data distribution and potential anomalies.
Beyond the Basics:
While this introduction scratches the surface, exploring further allows you to delve deeper:
- Built-in Functions: Leverage functions like math.isinf() and math.isnan() to check for infinity and differentiate it from Not-a-Number (NaN) values.
- Custom Functions: Craft functions tailored to your specific needs, implementing custom logic for calculations or comparisons involving infinity.
- Advanced Topics: Explore the use of infinity in understanding limits and convergence, handling large datasets with unbounded values, and tackling optimization problems with infinity constraints.
Dive into Infinity in Python, an enigmatic concept, and unlock its power to handle vast ranges, optimize algorithms, and analyze data effectively. Master comparisons, operations, and advanced applications with our expert Python Assignment Help and Python Homework Help.
Representing Infinity
While the concept of infinity stretches endlessly beyond our grasp, Python, the land of computers, offers its own interpretation. This "computational infinity" plays a crucial role in various scenarios, but understanding its representation and limitations is key to using it effectively.
float('inf') and float('-inf')
Unlike true mathematical infinity, which is impossible to store on a computer, Python provides two special values: float('inf') for positive infinity and float('-inf') for negative infinity. These act as stand-ins for magnitudes exceeding any regular number you can store. Think of them as the two extremes of an infinitely long number line.
Comparing Infinity and Regular Numbers
Infinity behaves somewhat like a regular number in comparisons. For example, inf > 5 is True, and -inf < 0 is also True. However, when it comes to arithmetic, things get interesting:
- Adding or subtracting infinity with a finite number remains infinity itself. So, inf + 100 and inf - 5 are both just inf.
- Dividing a positive number by zero results in inf, while dividing a negative number by zero results in -inf. This reflects the undefined nature of such operations.
Limitations of Infinity in Calculations
While infinity provides valuable capabilities, it's crucial to remember its limitations:
- Loss of precision: Performing arithmetic with infinity can lead to significant loss of precision due to rounding errors. Be cautious when interpreting results involving infinity.
- Not a magic number: Infinity doesn't solve all problems. It's not a substitute for proper error handling or careful algorithm design.
- Overflow and underflow: Performing calculations with very large or small numbers along with infinity can lead to overflow or underflow errors, causing unexpected results.
Working with Infinity in Python
Infinity, that elusive concept representing boundless magnitude, finds a place in the digital realm of Python. But unlike its mathematical counterpart, Python's infinity serves as a conceptual representation, opening doors to various applications while demanding careful handling. This article delves into working with infinity in Python, equipping you with the tools and knowledge to navigate its potential and limitations.
Built-in Functions:
Python offers several built-in functions to interact with infinity effectively:
- math.isinf(x): This function verifies if a number is either positive or negative infinity. It returns True if x is inf or -inf, and False otherwise.
- math.isnan(x): This function helps differentiate between infinity and another special value, Not a Number (NaN). NaN represents an undefined or invalid numerical result, while infinity signifies a boundless value. Both math.isinf(x) and math.isnan(x) are crucial for proper interpretation of numerical operations.
Utilizing Mathematical Functions:
While tempting, using mathematical functions directly with infinity can lead to unexpected results due to their inherent limitations:
- math.log(inf): This returns positive infinity, as the logarithm of a very large number approaches positive infinity.
- math.exp(-inf): This returns zero, as the exponential of a very negative number approaches zero.
Remember, these functions are designed for finite numbers, and their behavior with infinity might not align with mathematical expectations.
Custom Functions:
Beyond built-in functions, creating custom functions empowers you to handle infinity precisely within your specific context:
- Custom comparisons: Implement logic beyond standard operators like > or < to compare infinity based on your application's requirements. For example, you might define "larger" infinity based on the specific use case.
- Specialized operations: Craft functions tailored to perform calculations or manipulations involving infinity that align with your domain's needs. This ensures control and clarity in your code.
Remember:
- Loss of precision: Arithmetic involving infinity can lead to significant rounding errors, impacting the accuracy of results.
- Potential errors: Overflow or underflow errors can occur when combining large/small numbers with infinity. Handle these gracefully using exception handling.
- Not a magic solution: Infinity doesn't solve all problems. It's a tool to be used judiciously and combined with sound programming practices.
Advanced Topics of Infinity in Python
Having mastered the fundamentals of working with infinity in Python, let's venture into more intricate realms:
Limits and Convergence:
Imagine a sequence of numbers approaching closer and closer to a specific value as they go on infinitely. We can use infinity to understand this concept of limits. By analyzing whether the sequence converges (approaches a finite value) or diverges (goes off to positive or negative infinity), we gain valuable insights into mathematical functions and numerical methods.
For instance, the limit of 1/n as n approaches infinity is zero. This tells us that as we divide one by larger and larger numbers, the result gets closer and closer to zero, never actually reaching it.
Large Data and Optimization:
When dealing with massive datasets, some values might be exceptionally large or even unbounded. Infinity comes to the rescue here, allowing us to efficiently handle such scenarios. For instance, representing missing values with infinity helps analyze the data distribution and identify potential anomalies.
Optimization problems often involve constraints or variables that can take on unbounded values. Utilizing infinity to represent these cases enables us to formulate and solve such problems effectively. Imagine optimizing a function with a constraint that a variable can be arbitrarily large – infinity becomes an essential tool in defining and solving this problem.
Remember:
- Deep understanding: Mastering the concepts of limits, convergence, and optimization is crucial before applying infinity in these areas.
- Customizable tools: Building custom functions tailored to your specific problem allows you to leverage infinity's power precisely.
- Computational limitations: Infinity in Python is a conceptual representation and has limitations. Be mindful of potential errors and loss of precision.
Applications of Infinity in Python
Infinity may not physically exist, but in the world of Python, it plays a surprisingly practical role. Beyond its mathematical fascination, understanding how to work with infinity unlocks diverse applications across various domains. Let's explore some key scenarios where infinity shines:
Numerical Operations:
- Division by Zero: Ever encountered that dreaded "division by zero" error? Infinity offers a graceful solution. By representing undefined results as infinity, we avoid program crashes and ensure smooth execution. For example, x / 0 can be assigned float('inf') to indicate the undefined nature of the division.
- Unbounded Values: Real-world phenomena often involve magnitudes exceeding the limitations of finite numbers. Imagine representing the age of the universe or the size of subatomic particles. Infinity allows us to model such vast ranges, providing valuable insights even when dealing with values outside the realm of regular numbers.
- Iterative Algorithms: Optimization algorithms like gradient descent rely on iterative steps to find optimal solutions. Strategically utilizing infinity can guide the search process toward a solution efficiently. For instance, setting a step size to infinity in certain directions can allow the algorithm to explore further along promising paths.
Data Analysis: Unveiling Hidden Patterns
- Missing Values: Datasets often have missing entries, represented as NaN in Python. However, for certain analyses, treating these as infinity can be advantageous. It allows us to understand the data distribution and identify potential anomalies or outliers that might otherwise be masked by NaN values.
- Unbounded Ranges: Some datasets might have inherent open-ended ranges, like income levels or website visit durations. Modeling these with infinity provides a more realistic representation compared to imposing arbitrary limits. This allows us to analyze the data comprehensively, capturing the full spectrum of values.
Error Handling: Preventing Nasties and Ensuring Stability
- Default Values: Functions often require input values, but what if the user doesn't provide any? Setting default values to infinity can represent undefined or unavailable data gracefully. This prevents errors and ensures the function executes smoothly with meaningful placeholders.
- Division by Zero Woes: Remember the division by zero error? Well, infinity comes to the rescue again. By checking for potential division by zero before performing the operation and setting the result to infinity, we can prevent program crashes and maintain code stability.