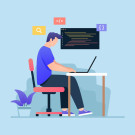
- 16th Nov 2023
- 14:18 pm
- Admin
Wrapper classes in Java act as a bridge between primitive data types and objects. They allow primitives to be treated as objects and enable the usage of primitives in situations that require objects. Each primitive type has a corresponding wrapper class—like Integer for int, Boolean for boolean—offering a way to work with primitives as objects. Autoboxing and unboxing simplify the conversion process between primitives and their wrapper class objects.
What are Wrapper classes in Java?
Wrapper classes in Java serve as a means to convert primitive data types into objects. Each primitive type in Java has a corresponding wrapper class—like Integer for int, Character for char, and Double for double. These classes allow primitives to be utilized as objects, enabling their use in situations where objects are essential. Wrapper classes offer methods to convert primitives into objects (boxing) and objects back into primitives (unboxing).
Additionally, they provide functionalities beyond what primitives can offer, such as parsing and converting string values to numeric types or handling null values that primitives cannot directly accommodate. This capability to treat primitive types as objects widens their applicability in object-oriented contexts, allowing for seamless integration in Java’s object-centric design.
Autoboxing
Autoboxing in Java simplifies the conversion process from primitive types to their corresponding wrapper classes. When assigning a primitive value to its wrapper class object, the compiler handles the conversion automatically. For instance, an int to Integer conversion is managed seamlessly by the compiler. This feature enhances code readability by providing a cleaner and more straightforward way to work with primitives as objects.
int num = 10; // Primitive int
Integer numObject = num; // Autoboxing - int to Integer
Unboxing
Unboxing refers to the automatic process of converting wrapper class objects back to their primitive data types. This feature allows for the extraction of primitive values from wrapper objects without explicitly invoking conversion methods. When a wrapper object is used as a primitive type, the Java compiler automatically manages the conversion, simplifying the process and contributing to clearer, more concise code. Unboxing facilitates the utilization of wrapper objects in scenarios that require primitive data types.
Integer numObject = 20; // Integer object
int num = numObject; // Unboxing - Integer to int
Custom Wrapper Classes in Java
Developers can create custom wrapper classes in Java, enabling the encapsulation of primitive data types within specialized classes of their own. These custom wrappers are designed to meet specific requirements beyond the standard wrapper classes provided by Java. Developers can define custom functionalities, add specialized methods, and incorporate unique attributes within these wrapper classes.
By creating custom wrappers, developers can tailor functionalities to address domain-specific needs or complex data structures. For instance, a custom wrapper might handle advanced mathematical operations, specific data manipulations, or unique data validations.
These custom wrappers enable a more intricate and tailored approach to handling primitive types within the context of specialized applications, providing a way to encapsulate complex logic and specific behaviors within the wrapper, which is not feasible with the standard Java wrapper classes.
class CustomInteger {
private int value;
public CustomInteger(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
CustomInteger customInt = new CustomInteger(15); // Custom wrapper class
int extractedValue = customInt.getValue();
Convert Primitive Type to Wrapper Objects
Converting primitive types to their corresponding wrapper objects in Java involves the utilization of wrapper classes such as Integer, Double, or Boolean. This process, termed "boxing," allows the representation of primitive types as objects. The wrapper classes provide methods like valueOf() to convert primitive types to their respective object representations. For example, the Integer.valueOf(int) method transforms an int into its Integer object equivalent. This conversion mechanism is crucial in scenarios where object-oriented paradigms are required, enabling the usage of primitives within object-centric functionalities, such as collections or methods that demand object types.
int num = 25; // Primitive int
Integer wrapperNum = Integer.valueOf(num); // Convert int to Integer
Wrapper Objects into Primitive Types
The process of converting wrapper objects back to their underlying primitive data types in Java, known as "unboxing," involves retrieving the primitive value from its corresponding wrapper object. Wrapper classes such as Integer, Boolean, and others provide methods like intValue(), booleanValue(), which allow the extraction of the primitive value from the wrapper object. For instance, the intValue() method retrieves the int value from an Integer object. Unboxing plays a crucial role in scenarios where the application requires primitive data types instead of objects, allowing the seamless transition from objects to primitives when necessary. This conversion process ensures compatibility with methods or operations that specifically demand primitive types, enhancing flexibility within the Java language.
Integer wrapper = new Integer(30); // Integer object
int value = wrapper.intValue(); // Convert Integer to int
Need of Wrapper Classes in Java
Wrapper classes in Java fulfill a critical role in scenarios where objects are required, but primitive data types are involved.
- Compatibility with Collections: Wrapper classes aid in storing primitive data types within collection frameworks like Lists, Sets, and Maps, which necessitate object types.
- Utilization in Generics: Within generics, where only object types can be used, wrapper classes are essential for incorporating primitive data types.
- Handling Null Values: Wrapper classes offer an object-based representation, aiding in handling null values when dealing with primitive data types.
- Scenarios Requiring Objects: In contexts where objects are required, such as certain APIs or methods, wrapper classes enable the usage of primitives by converting them into objects.
Advantages of Wrapper Classes in Java
Wrapper classes in Java Programming offer numerous advantages, enhancing the flexibility and compatibility of the language:
- Object-Based Operations: Wrapper classes enable primitive types to be used in scenarios that require objects, facilitating operations like collection handling or interactions with methods that specifically demand objects.
- Enhanced Collection Frameworks: They allow primitive data types to be included in collection classes (e.g., Lists, Sets) that mandate object types, enhancing compatibility with these essential Java components.
- Compatibility with Generics: In generic contexts where only object types are permissible, wrapper classes become crucial for integrating primitive data types.
- Null Value Handling: Wrapper classes provide object representations for managing null values in primitive data types, addressing scenarios where null is required as a value.
- Support for APIs and Methods: In certain scenarios that necessitate object types, wrapper classes assist by allowing primitives to be used as objects.
Disadvantages of Wrapper Classes in Java
Wrapper classes in Java, while providing significant advantages, also carry certain limitations:
- Performance Overhead: Wrapper classes incur a performance cost due to the additional memory allocation and object creation processes associated with them. This overhead impacts the memory footprint of the application.
- Boxing/Unboxing Overhead: The process of converting primitives to objects (boxing) and objects back to primitives (unboxing) introduces computational overhead, potentially impacting performance.
- Increased Complexity: Usage of wrapper classes can introduce additional complexity to the codebase, especially when considering scenarios involving boxing, unboxing, and object-oriented operations with primitives.
- Potential for NullPointerExceptions: The introduction of wrapper classes brings the potential for NullPointerExceptions when working with null values in scenarios where primitives do not naturally allow for null values.