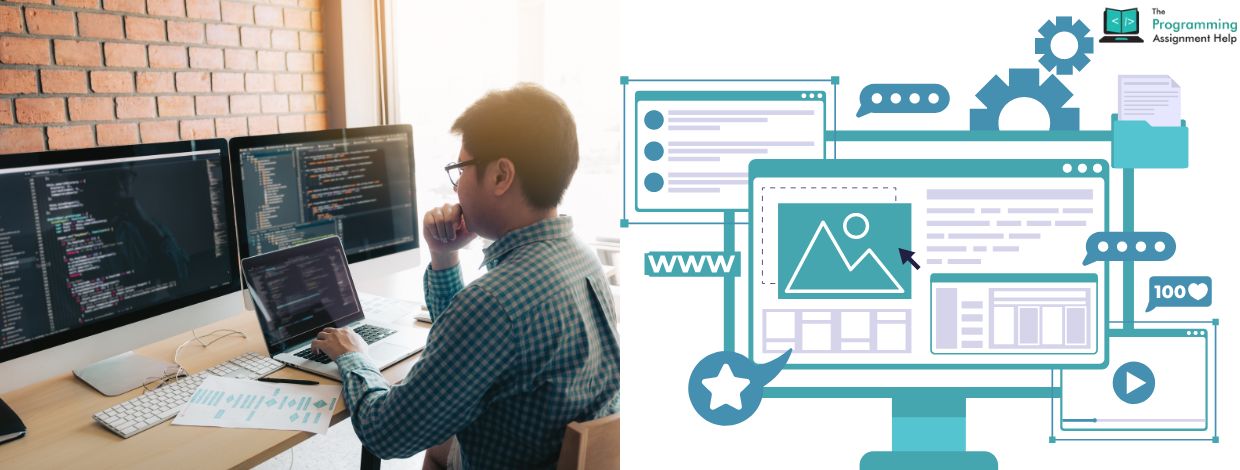
- 1st Oct 2024
- 17:48 pm
- Stephen
Squaring a number can be defined as one of the most fundamental mathematical operations you’ll come across in programming. When you square a number, you multiply it by itself. For example, squaring 4 means calculating 4×4=16. This operation is widely used in various fields like data analysis, machine learning, physics calculations, and more. In Python, there are several ways to perform this simple task, each offering flexibility depending on the context of your project.
Here are six different ways to square a number in Python:
1. Using the Exponentiation Operator (**)
The exponentiation operator is the most common and direct way to square a number in Python. It is simple, intuitive, and commonly used for raising any number to a power. In this approach, raising a number to the power of 2 results in its squared value. This method is versatile and works with both integers and floating-point numbers.
2. Using the pow() Function
Python's built-in `pow()` function enables you to raise a number to any specified power, making it easy to square a number by simply passing 2 as the exponent. This approach is particularly useful when you're already working with exponentiation in your code, providing added flexibility for various mathematical operations. It also handles negative bases and can be useful when you need a generalized approach to exponents.
3. Using Multiplication
One of the easiest ways to square a number is by multiplying it by itself. Although this method might seem less elegant than using an operator, it is just as effective. This approach can be useful in cases where you want to avoid using specific mathematical functions or operators.
4. Using List Comprehension (Advanced Use Case)
If you're dealing with lists or arrays of numbers, Python's list comprehension feature allows you to square each element in a list efficiently. This approach is especially handy when you need to perform the operation on multiple numbers simultaneously. It's also a more Pythonic way to handle such tasks and is widely used in data processing applications.
5. Using numpy Library
If you're working on projects that involve large-scale mathematical calculations or data analysis, the numpy library is a highly efficient tool. With numpy, squaring a number or even an array of numbers becomes simple. This method is particularly beneficial in cases where performance is crucial, such as machine learning or scientific computing projects.
6. Using a Lambda Function
Another flexible method to square numbers in Python is by using a lambda function. Lambda functions are anonymous functions defined at runtime. You can quickly define a function to square a number using this approach, especially when you need to apply the squaring operation dynamically or inline in your code.
Conclusion
Whether you're working on basic programming tasks or more complex mathematical computations, Python offers several methods to square a number. From simple operators like ** to more specialized tools like the numpy library, each method has its use case depending on the complexity and scale of your project. Understanding these various methods can enhance your ability to write efficient and effective Python code tailored to your specific needs. If you find yourself facing challenges or needing help with Python assignments, The Programming Assignment Help website is a great resource that provides expert guidance and solutions to help you overcome your coding hurdles with confidence.