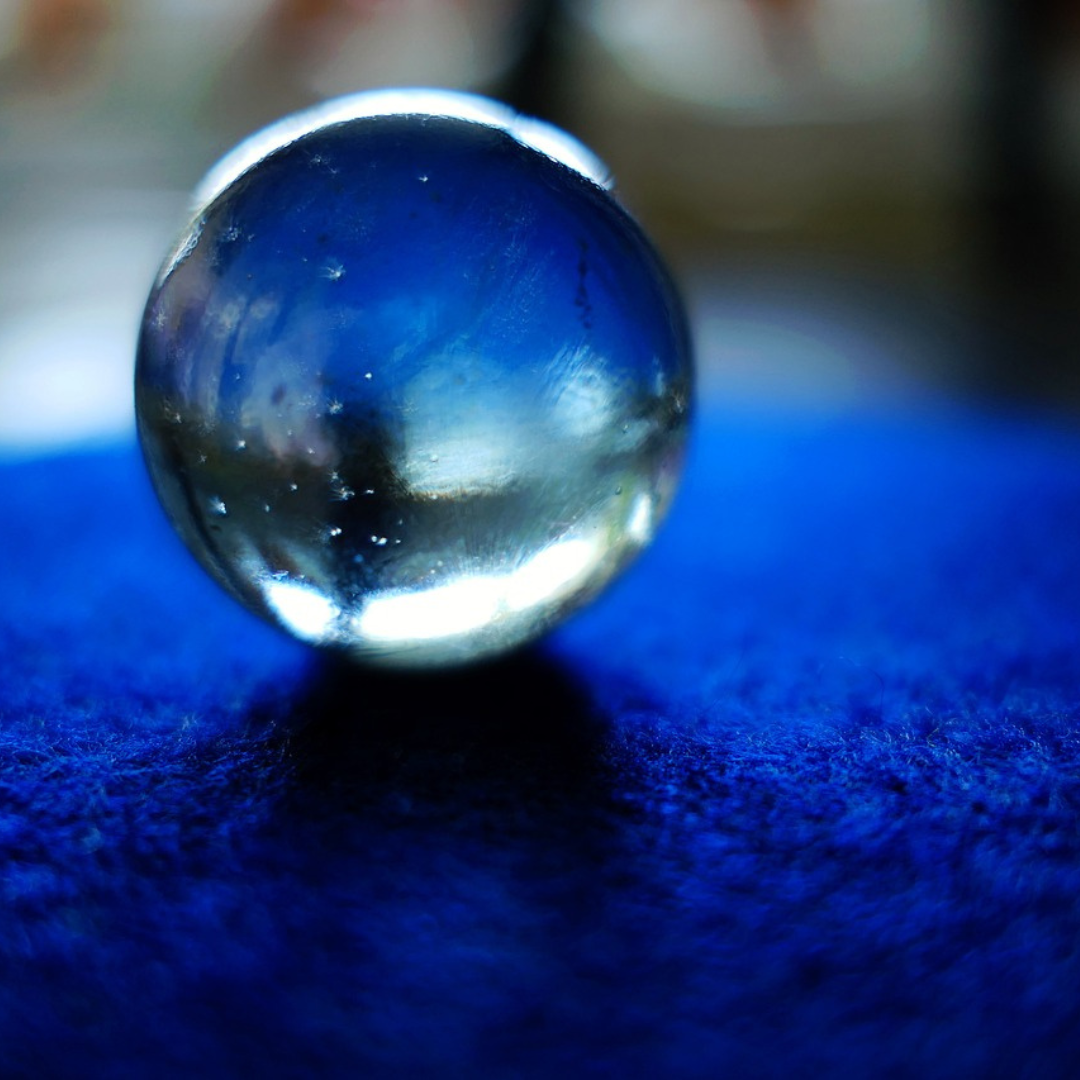
- 3rd Apr 2023
- 09:00 am
- Admin
Java Homework Question - Volume and Surface of Sphere using Math Class - Write an app that reads the radius of a sphere and prints its volume and surface area. Print the output to four decimal places.
Java Homework Solution
Here's the Java code for the app that reads the radius of a sphere and prints its volume and surface area using the Math class:
import java.util.Scanner;
public class SphereVolumeAndSurface {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Read the radius of the sphere
System.out.print("Enter the radius of the sphere: ");
double radius = input.nextDouble();
// Calculate the volume of the sphere using the formula V = (4/3) * pi * r^3
double volume = (4.0 / 3.0) * Math.PI * Math.pow(radius, 3);
// Calculate the surface area of the sphere using the formula A = 4 * pi * r^2
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
// Print the volume and surface area of the sphere to four decimal places
System.out.printf("The volume of the sphere is %.4f\n", volume);
System.out.printf("The surface area of the sphere is %.4f\n", surfaceArea);
input.close();
}
}When you run this code and enter the radius of the sphere, the program calculates the volume and surface area of the sphere using the Math class and prints them to four decimal places. For example:
Enter the radius of the sphere: 5.5
The volume of the sphere is 696.3205
The surface area of the sphere is 379.7338
Note that we used the Math.pow() method to calculate the power of a number, and the printf() method with the %f format specifier to print the volume and surface area to four decimal places.