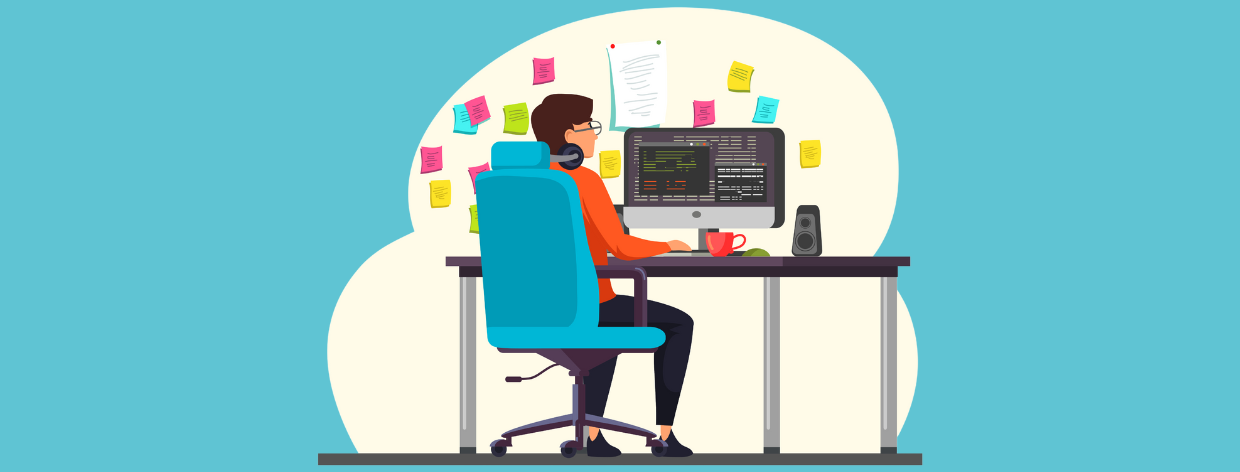
- 16th Feb 2024
- 00:34 am
- Adan Salman
In the versatile world of Python, objects dance their intricate dance. But sometimes, we need them to speak up! String conversions translate these objects into human-readable text, unlocking a realm of possibilities:
- Printing and logging: From debugging woes to tracking program flow, clear representations help us unravel what's happening under the hood.
- Data exchange: Sharing information across systems? Encoding objects as strings paves the way for seamless communication.
- User interfaces: Visualizing data is key to conveying insights. String conversions give objects a voice on the user interface stage.
What is tostring() in Python?
While Python lacks an explicit tostring() method like some other languages, its rich object system and various conversion approaches empower you to achieve similar functionality. Understanding these alternatives is crucial for crafting meaningful string representations of your data.
The "Missing" tostring():
Unlike Java or C++, Python doesn't offer a dedicated tostring() method directly attached to objects. However, this doesn't limit your ability to convert objects to strings.
Alternative Avenues:
- The Built-in str() Function:
The most fundamental approach, directly converts objects to strings using str(object). It provides basic conversion but might not capture all relevant details.
- The __str__() Method:
This special method allows you to define custom string representations for your objects. It offers fine-grained control over the output format, enabling you to include specific attributes and tailor the representation to your needs.
- Formatting Techniques:
Leverage f-strings, .format(), or string concatenation to craft informative strings by incorporating object attributes within the string itself.
Choosing the Right Tool:
a. For simple conversions, str() suffices.
b. For control and informative representations, __str__() shines.
c. Formatting techniques offer flexibility for specific use cases.
Beyond Conversion:
Remember, tostring()-like conversions go beyond just generating strings. They're about creating meaningful representations that serve your application's needs. Consider context, clarity, and maintainability when crafting your conversions.
Common String Conversion Methods
Converting objects to strings in Python forms the bedrock of various essential tasks, from printing and logging to data exchange and user interactions. While the built-in str() function offers a quick solution, it often lacks the nuance and customization needed for more intricate scenarios. Thankfully, Python provides a rich arsenal of methods to unlock the expressive power of string conversions. Let's delve into four popular methods, each with its unique strengths:
- Using str() Function:
Think of str() as the friendly neighborhood translator, readily converting basic data types into their string counterparts. Integers become numbers, floats get their decimal points, and even lists transform into comma-separated lists. It's simple, direct, and perfect for quick conversions:
number = 42
message = str(number) # message now holds "42"
However, str() offers limited control over formatting. Numbers always appear in their raw form, and complex data structures like dictionaries might not translate as intuitively.
- Using "%s" Keyword:
Once upon a time, the %s keyword reigned supreme in string formatting. It partnered with the % operator to weave variables and expressions into strings:
name = "Alice"
greeting = "Hello, %s!" % name # greeting becomes "Hello, Alice!"
While it served its purpose, readability suffered due to the cryptic syntax. Thankfully, modern methods like .format() and f-strings offer clearer and more maintainable alternatives.
- The Versatile .format() Function:
.format() brings structure and flexibility to string formatting. It employs placeholders ({}) for variables and expressions, offering various formatting options:
price = 19.99
formatted_price = "The price is ${:.2f}".format(price) # formatted_price becomes "The price is $19.99"
Positional and named placeholders provide further control, and advanced features like alignment and padding enable intricate formatting:
name = "Bob"
age = 30
greeting = "{age:>3} years young, {name} is ready for action!".format(age=age, name=name) # greeting becomes " 30 years young, Bob is ready for action!"
While powerful, .format() can sometimes feel slightly verbose. This is where f-strings step in.
- Using f-strings:
F-strings, introduced in Python 3.6, redefine string formatting with elegance and readability. They embed variables and expressions directly within the string using f-literals:
temperature = 25.5
message = f"The temperature is {temperature:.1f} degrees Celsius." # message becomes "The temperature is 25.5 degrees Celsius."
F-strings seamlessly integrate formatting options and expressions, offering an intuitive and concise syntax:
full_name = f"{name.title()} {surname.upper()}" # full_name becomes "Bob Smith" (assuming name and surname are variables)
Their readability and maintainability make f-strings a popular choice for modern Python development.
Advanced String Conversion Techniques
While the fundamental methods like str(), .format(), and f-strings provide the foundation for converting objects to strings in Python, venturing into advanced techniques unlocks remarkable potential to tailor and enrich your data's voice. This expanded exploration delves into three powerful tools that elevate your string conversion game, equipping you to:
1. Using the __str__() method:
Think of __str__() as a personal stylist for your objects, allowing you to define how they present themselves in text. Imagine crafting informative descriptions that go beyond generic output. You can:
- Embed key details: Use f-strings or concatenation to weave essential attributes into the string. For example, a Book object's __str__() might return "Title: {title}, Author: {author} ({year})".
- Prioritize user understanding: Keep it clear and concise. Avoid cluttering the string with unnecessary details, tailoring the output to your audience.
- Adapt to context: Consider different formats depending on where the string will be used, whether it's for logging, user interfaces, or data exchange.
class Book:
def __init__(self, title, author, year):
self.title = title
self.author = author
self.year = year
def __str__(self):
return f"Title: {self.title}, Author: {self.author} ({self.year})"
book = Book("The Lord of the Rings", "J.R.R. Tolkien", 1954)
print(book) # Output: Title: The Lord of the Rings, Author: J.R.R. Tolkien (1954)
2. Using str.isdigit() for validation:
While str.isdigit() offers a basic check for digits, it has limitations. For robust data validation, consider these options:
- Regular expressions: These patterns act like detailed blueprints, precisely matching specific formats like phone numbers or email addresses. Imagine a pattern like r"^\d{3}-\d{3}-\d{4}$" to validate US phone numbers.
import re
phone_regex = r"^\d{3}-\d{3}-\d{4}$" # Matches US phone numbers
def validate_phone(phone_number):
return bool(re.match(phone_regex, phone_number))
print(validate_phone("123-456-7890")) # True
print(validate_phone("abc-def-ghij")) # False
- Custom validation functions: Tailor validation logic to your exact needs. Create functions that not only check for digits but also consider other constraints like length, presence of symbols, or adherence to custom patterns.
def validate_username(username):
if len(username) < 6:
return False
if not username.isalnum():
return False
return True
print(validate_username("valid_user")) # True
print(validate_username("short")) # False
print(validate_username("user@123")) # False
3. Using the .join() method:
Think of .join() as a conductor, bringing together elements from an iterable (like a list) into a harmonious string. But it's not just about adding commas:
- Spice up your strings: Use different separators for personality! Instead of commas, try hyphens for product codes or semicolons for CSV-like formats.
- Choose what to join: Apply logic to decide which elements to include. Filter out specific values or control inclusion based on certain criteria, enabling dynamic string construction.
- Add special touches: Insert custom functions as separators for advanced formatting. Imagine inserting formatting or additional information between elements for informative strings.
items = ["apple", "banana", "orange"]
print(", ".join(items)) # Output: apple, banana, orange
print("-".join(items)) # Output: apple-banana-orange
filtered_items = [item for item in items if int(item) > 0] # Filter numbers
print(", ".join(str(item) for item in filtered_items)) # Output: 2, 3
Best Practices and Considerations
While mastering advanced techniques unlocks power, a solid foundation built on best practices ensures clarity, maintainability, and efficiency in your string conversions. Here are key considerations:
1. Clarity and Conciseness:
- Informative, not exhaustive: Include relevant attributes, but avoid cluttering the string with unnecessary details. Aim for a balance that informs without overwhelming.
- Consider your audience: Tailor the representation to resonate with the intended user, whether it's technical personnel or end users.
- Context matters: Adapt the format based on where the string will be used (logging, user interfaces, data exchange).
2. Choosing the Right Tool:
- Match complexity to method: Don't use advanced techniques like __str__() or regular expressions for simple tasks. Evaluate the complexity of your conversion needs and choose the most efficient method.
- Prioritize readability: Strive for code that is clear, well-commented, and easy to understand for future maintenance.
- Performance considerations: For large datasets, be mindful of the performance implications of different methods. str.join() might be faster than complex f-strings or custom functions.
3. Maintainability Matters:
- Adhere to coding conventions: Follow established PEP 8 guidelines for consistent and readable code.
- Descriptive variable names: Choose names that clearly communicate the purpose of variables holding string representations.
- Document your choices: Explain the rationale behind your string conversion methods, especially for complex scenarios, to aid future understanding.
Applications and Use Cases of __str__() in Python
While seemingly simple, the __str__() method in Python serves as a powerful tool for crafting informative and tailored representations of objects. Its applications extend far beyond the basic conversion to a string, providing versatility across various domains:
1. Debugging and Logging:
- Invaluable for inspecting object state during debugging. Custom __str__() can reveal key attributes, aiding in pinpointing issues and understanding object interactions.
- Logging benefits greatly from well-defined __str__() methods. By incorporating relevant details, logs become more informative and easier to analyze, facilitating error identification and system monitoring.
2. User Interfaces and Data Visualization:
- User interfaces rely heavily on string representations for displaying information. Tailoring __str__() allows you to control how objects are presented, ensuring clarity and user-friendliness.
- Data visualization tools often leverage __str__() to generate labels, axes, and tooltips. By customizing these representations, you can enhance the clarity and interpretability of your visualizations.
3. Data Persistence and Exchange:
- When objects need to be stored or transmitted as strings (e.g., in databases or network communication), __str__() defines the format. Well-designed __str__() ensures consistent and interpretable data even after deserialization.
- Different applications might require different levels of detail in the string representation. __str__() allows you to adapt the output based on the context and purpose of data exchange.
4. Custom Data Structures and Classes:
- When creating custom data structures or classes, defining __str__() becomes crucial for interacting with and debugging them effectively. It allows you to inspect the contents of your custom objects in a meaningful way.
- Complex data structures like graphs or trees can benefit from custom __str__() implementations that present their hierarchy and relationships clearly.
5. Testing and Code Readability:
- Unit tests often rely on string comparisons to verify object behavior. Well-defined __str__() ensures that these comparisons are meaningful and reliable, improving test clarity and maintainability.
- Reading and understanding code becomes easier when objects have informative __str__() methods. This can be especially helpful for debugging, refactoring, and collaborating with other developers.