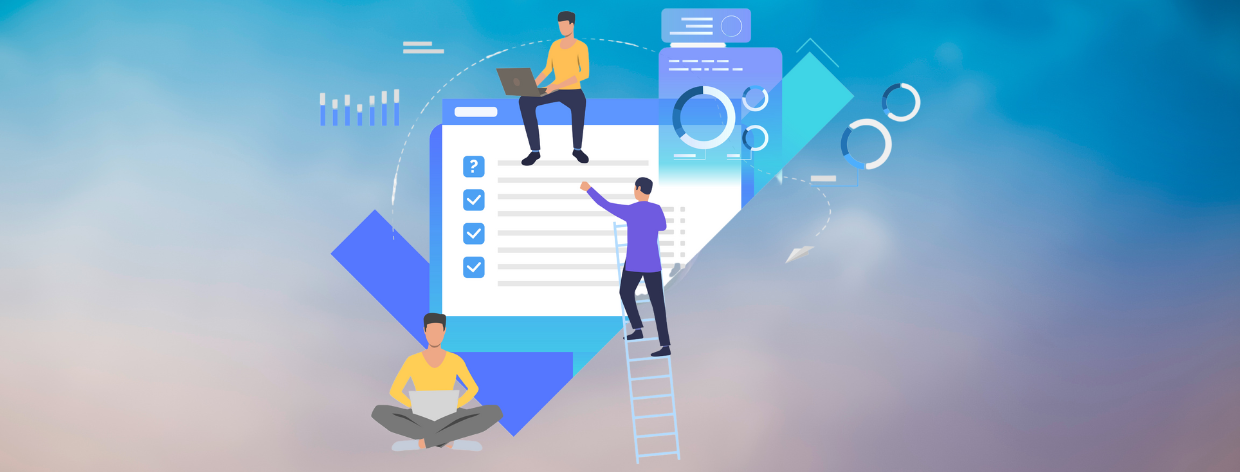
- 20th Feb 2024
- 23:30 pm
- Adan Salman
Numerical data forms the backbone of countless programming tasks, from calculations to machine learning. But this valuable information doesn't always arrive ready-made. Often, it hides within textual strings, disguised as words. Converting these strings to "floats," a special type of number in Python, becomes crucial for analyzing and working with the data.
Converting these strings to floats allows us to perform calculations, identify trends, and uncover hidden patterns. Several methods tackle this conversion, each with its own pros and cons. The built-in float() function works directly, but it only accepts strings in specific formats and might lose some data.
To handle potential errors, the try-except block acts as a safety net, catching issues and preventing crashes. The isinstance() function further helps by ensuring we only try to convert genuine strings.
For more control and stricter checks, regular expressions, inspecting strings and confirming they match valid float formats before conversion. This ensures data accuracy and prevents problems later.
Powerful libraries like numpy and pandas joins, offering speedy conversions on large datasets using special techniques. They're ideal for handling tons of textual data efficiently.
Master string-to-float in Python for powerful data analysis! Explore methods, experiment, and seek guidance from our expert Python Assignment Help and Python Homework help. Remember, true learning comes from independent problem-solving and active engagement.
Built-in String-to-Float Conversions Method
In the realm of Python programming, numerical data reigns supreme. From intricate calculations to machine learning algorithms, numbers form the lifeblood of countless tasks. However, this valuable information doesn't always arrive in its most readily usable form. Often, numerical data hides within textual strings, posing a conversion challenge. This is where mastering built-in string conversion methods becomes crucial, empowering you to unlock the true potential of numerical data.
1. float():
The built-in float() function stands as the first line of defense, offering direct string-to-float conversion. It readily accepts strings representing numbers in standard decimal or scientific notation, making it convenient for basic conversions. However, be wary of its limitations:
- Strict format requirements: Only specific formats like "123.45" or "1.2e3" are accepted, leading to errors for non-compliant strings.
- Potential data loss: During conversion, trailing zeros after the decimal point might be truncated, causing slight data loss.
2. try-except Block:
When dealing with unpredictable data, the try-except block emerges as your guardian angel. It allows you to gracefully handle potential ValueError exceptions that might arise due to invalid string formats:
try:
float_value = float("invalid_string")
except ValueError:
print("Error: Invalid string format for conversion.")
With this approach, you can ensure your program doesn't crash even when encountering unexpected input, making it more robust and user-friendly.
3. isinstance():
Before wielding the conversion tools, the isinstance() function acts as a vigilant sentinel, verifying that the input is indeed a string. This simple check can prevent unnecessary errors and streamline your conversion process:
if isinstance(data, str):
float_value = float(data)
else:
print("Error: Input must be a string.")
By incorporating this check, you avoid attempting to convert non-string data, saving you from potential debugging headaches later.
4. Regular Expressions:
For intricate control and pre-processing, regular expressions become your detectives. They meticulously scrutinize strings using defined patterns, ensuring they adhere to valid float formats before conversion:
import re
pattern = r"^-?\d+(\.\d+)?(e[+-]?\d+)?$"
if re.match(pattern, data):
float_value = float(data)
else:
print("Error: Invalid string format for float.")
This approach offers enhanced control and data integrity, particularly useful when dealing with non-standard or user-generated data formats.
Third-Party Libraries and Custom Functions
While built-in methods provide a solid foundation, ventures into third-party libraries and custom functions can unlock even more efficient and versatile string-to-float conversions in Python.
1. numpy and pandas:
For large datasets, the vectorized operations of numpy and pandas shine. Imagine converting thousands of sensor readings from strings to floats:
import numpy as np
strings = ["12.34", "56.789", "-4.56e2"]
floats = np.array(strings, dtype=np.float32)
print(floats) # Output: [ 12.34 56.789 -456. ]
Advantages:
- Blazing speed for large datasets, leveraging vectorized operations.
- Convenient integration with data manipulation tools within libraries.
Limitations:
- Not ideal for single-string conversions, additional library setup required.
2. pandas:
Beyond efficiency, pandas offers specific string conversion features:
import pandas as pd
data = {"sensor_data": ["12.34mV", "56.78A", "-4.56e2W"]}
df = pd.DataFrame(data)
df["sensor_data"] = pd.to_numeric(df["sensor_data"].str.replace("[^-\d\.]", ""))
print(df)
Advantages:
- Handles multiple strings within DataFrames efficiently.
- Offers pre-processing options like unit removal with regular expressions.
Limitations:
- Library overhead for smaller datasets.
3. Custom Functions:
For unique needs and complex conversions, crafting custom functions empowers you to tailor the process:
def custom_converter(string):
if "K" in string:
return float(string[:-1]) * 1000
else:
return float(string)
data = ["12.34K", "56.78", "-4.56e2"]
floats = [custom_converter(d) for d in data]
print(floats) # Output: [12340.0, 56.78, -456.0]
Advantages:
- Complete control over conversion logic for specific requirements.
- Reusable solution for repeated complex conversions.
Limitations:
- Development and testing overhead compared to built-in methods.
Remember, the "best" approach depends on your specific scenario. For large datasets and efficiency, libraries like numpy and pandas rule. For tailored conversions and intricate logic, custom functions reign supreme. Experiment and explore to find the perfect tool for bridging the gap between text and numbers in your Python projects!
Mastering String-to-Float Conversions in Python
While seemingly simple, the act of converting strings to floats in Python carries hidden complexities and demands thoughtful consideration. Choosing the right method empowers you to navigate diverse data scenarios effectively, ensuring accuracy, efficiency, and robust code. Here, we delve into best practices to illuminate your path:
1. Method Selection:
The "one-size-fits-all" approach seldom prevails in the realm of data manipulation. Consider these factors when selecting your conversion method:
- Performance: For massive datasets, vectorized operations in numpy or pandas reign supreme. For smaller conversions, built-in methods like float() suffice.
- Data Size: When dealing with thousands of strings, prioritize efficiency. Libraries like numpy can handle bulk conversions seamlessly.
- Error Handling: Anticipate potential issues. Utilize try-except blocks to gracefully handle invalid formats and prevent program crashes.
2. Locale Considerations:
Remember, the world doesn't revolve solely around periods and commas as decimal separators. Be mindful of locale-specific formatting. Regular expressions or dedicated libraries can help pre-process strings based on regional conventions.
3. Precision Matters:
Conversion can sometimes lead to precision loss, particularly when dealing with very large or very small numbers. Be aware of the potential impact on your analysis and choose methods (like the decimal library) that can handle high precision if necessary.
4. Invalid Input:
Not all strings are created equal. Implement robust checks to identify and handle invalid input gracefully. Use isinstance() to ensure you're converting strings and employ regular expressions to validate specific format requirements.
5. Readability and Maintainability:
Write clean, well-documented code that future you (or others) can understand. Choose meaningful variable names, comment your code, and break down complex logic into smaller functions. This enhances maintainability and promotes collaboration.
6. Testing:
Don't underestimate the power of testing. Write unit tests to ensure your conversion functions work as expected with various input formats and edge cases. This instills confidence in your code and prevents downstream errors.
7. Continuous Learning:
The world of data manipulation is constantly evolving. Stay updated on new libraries, techniques, and best practices. Explore advanced methods like custom functions for intricate requirements or leverage the power of online communities for guidance and support.
Applications of Strings to Floats in Python
While converting strings to floats might seem like a straightforward task, mastering this ability unlocks diverse applications in Python programming. It serves as a crucial bridge between textual data and numerical analysis, empowering you to extract valuable insights from various sources. Let's explore some compelling use cases:
- Analyzing Sensor Data: Imagine analyzing temperature readings stored as strings like "25°C" or "-10.4°F." Converting these strings to floats allows you to calculate averages, identify trends, and monitor sensor health. Custom functions with conditional logic can handle units and different temperature scales.
- Processing Financial Transactions: Financial records often come in textual formats like "$1,234.56" or "€-100.00." By converting these strings to floats, you can calculate totals, track expenses, and analyze spending patterns. Regular expressions can help extract relevant values while accounting for diverse currencies and formatting variations.
- Parsing Scientific Data: Scientific datasets frequently store measurements and calculations as strings. Converting these strings to floats enables further analysis, statistical methods, and data visualization. Advanced regular expressions can tackle scientific notation, while lambda functions can handle conditional conversions based on units or data types.
- Preprocessing Text Data for Machine Learning: Natural Language Processing (NLP) tasks often require numerical representations of text. Converting words or phrases to numerical vectors (like TF-IDF) based on their frequency or semantic relationships unlocks machine learning applications like sentiment analysis or topic modeling. Libraries like pandas and custom functions can efficiently preprocess large text datasets.
- Cleaning and Validating User Input: User input frequently exists as strings and might contain errors or inconsistencies. Converting strings to floats with robust error handling and format validation helps ensure data integrity and prevents downstream issues in calculations or analysis. Techniques like try-except blocks and isinstance() functions can safeguard against invalid input.
- Working with Data from Different Sources: Data often comes from diverse sources, each with its own formatting conventions. Mastering string-to-float conversions empowers you to seamlessly integrate and analyze data from various formats, like CSV files, web scraping results, or APIs.