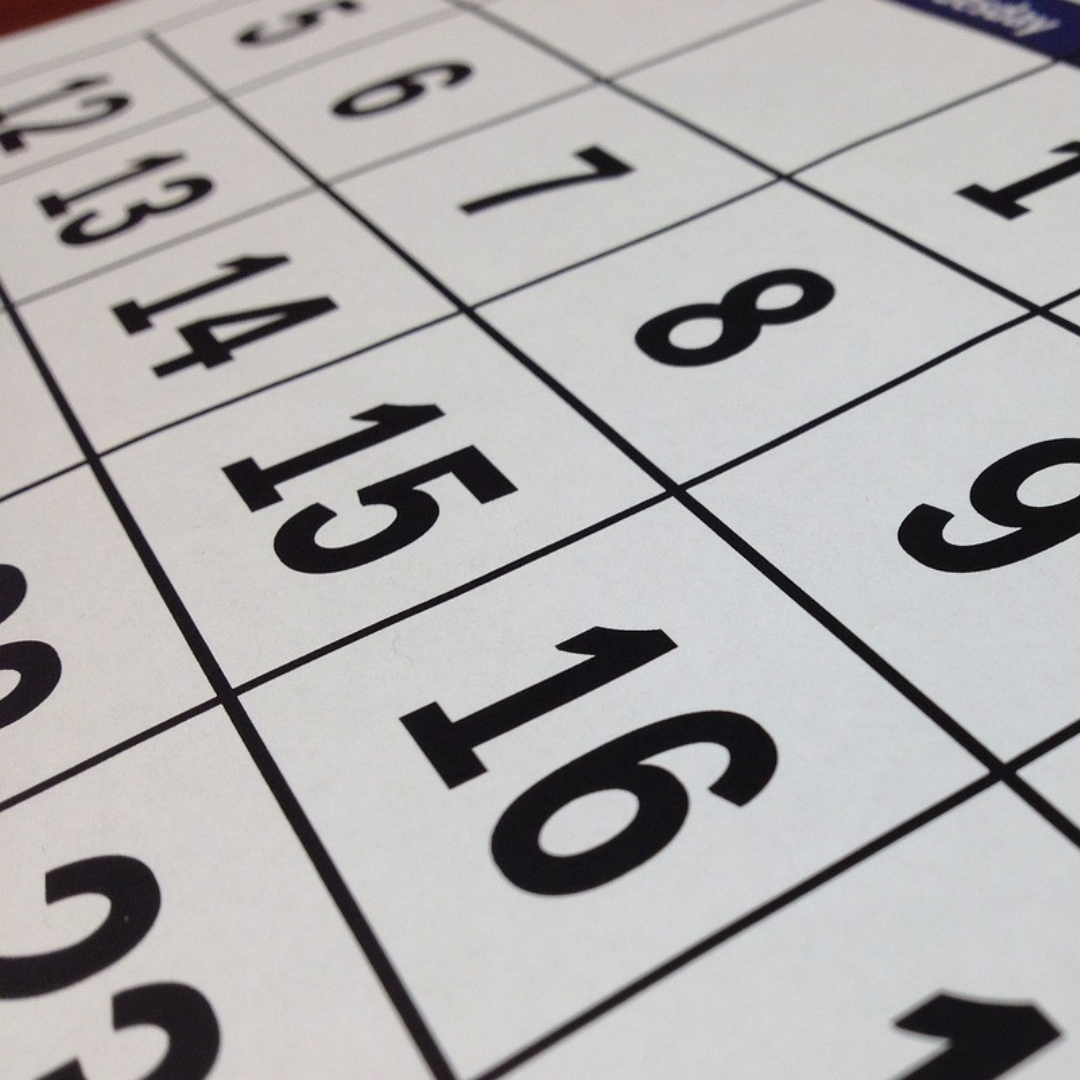
- 3rd Apr 2023
- 07:28 am
C++ Homework Question
C++ String Comparison (using String, Compare, String Methods, Loops)- Write a program that asks the user to enter two names and store them in string objects. It should then report whether they are the same or not, ignoring case, To help accomplish its task, it should use two functions in addition to main(). The functions are: StringUppercaseIt(string s) boolean samestring (string s1, string s2) The same string function, which receives the two strings to be compared, will need to call uppercaseIt for each of them before testing if they are the same. The uppercase function should use a loop so that it can call the toupper function for every character in the string it receives before returning it back to the samestring function.
C++ Homework Solution
#include
#include
using namespace std;
// function prototypes
string StringUppercaseIt(string s);
bool sameString(string s1, string s2);
int main()
{
string name1, name2;
// get user input
cout << "Enter the first name: ";
getline(cin, name1);
cout << "Enter the second name: ";
getline(cin, name2);
// compare names
if (sameString(name1, name2)) {
cout << "The names are the same." << endl;
} else {
cout << "The names are different." << endl;
}
return 0;
}
// function definitions
string StringUppercaseIt(string s)
{
for (int i = 0; i < s.length(); i++) {
s[i] = toupper(s[i]);
}
return s;
}
bool sameString(string s1, string s2)
{
// convert strings to uppercase
s1 = StringUppercaseIt(s1);
s2 = StringUppercaseIt(s2);
// compare strings
if (s1.compare(s2) == 0) {
return true;
} else {
return false;
}
}
The StringUppercaseIt function takes a string and returns a new string with all characters in uppercase. It uses a loop to iterate over each character and applies the toupper function to it.
The sameString function takes two strings and checks if they are the same, ignoring case. It first converts both strings to uppercase using the StringUppercaseIt function, and then compares them using the compare method of the first string. If the result of compare is zero, the strings are the same. Otherwise, they are different.
In the main function, the program gets two names from the user and passes them to the sameString function to check if they are the same. The result is displayed to the user.