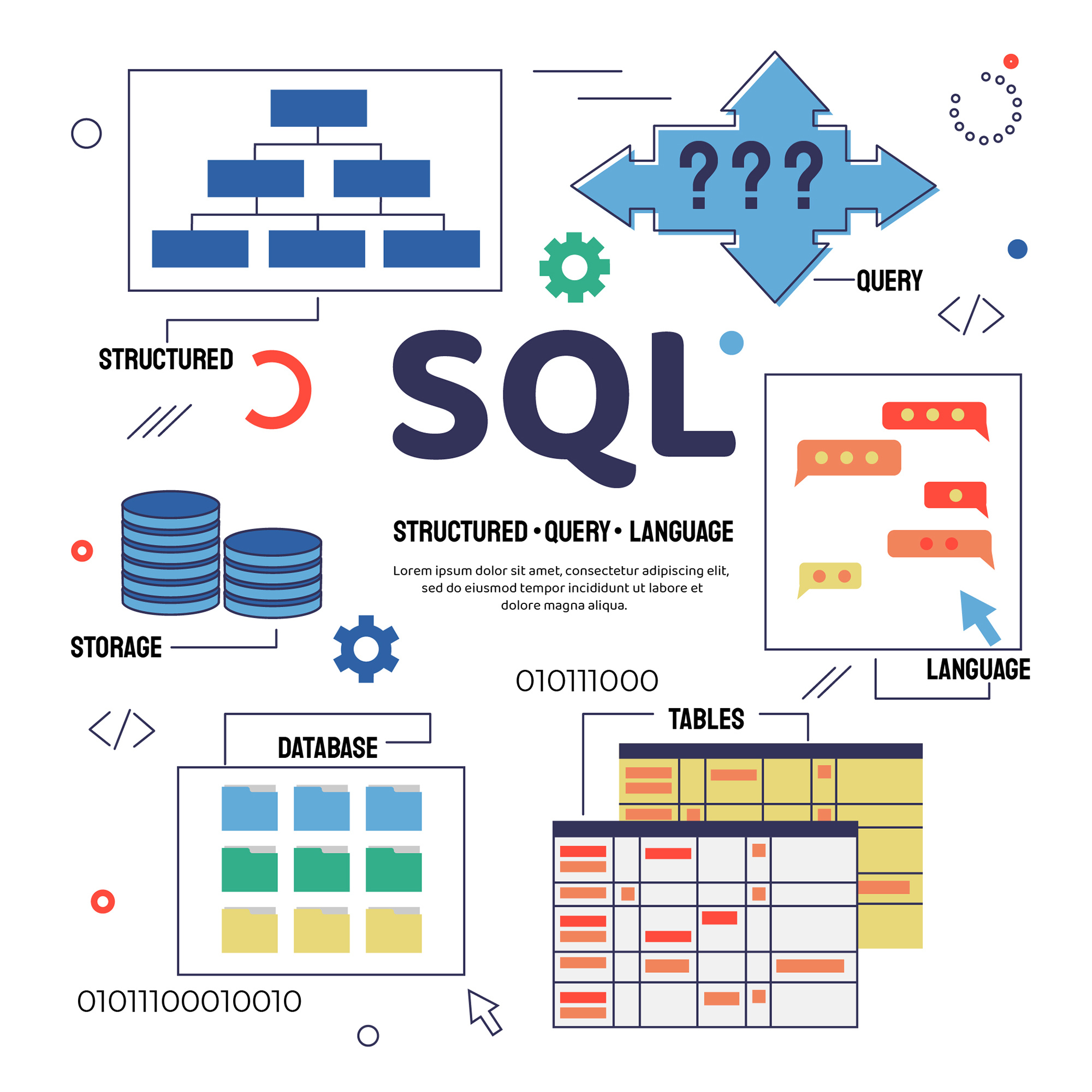
- 1st Dec 2023
- 20:48 pm
- Admin
SQL queries are fundamental tools in the dynamic field of data management, serving as the linchpin that enables smooth interactions with databases. SQL, known as Structured Query Language, emerges as the universal tool for interacting with relational databases, becoming a crucial proficiency for those involved in data manipulation. This in-depth manual explores the nuances of SQL queries, addressing a spectrum that spans from foundational principles to advanced methodologies.
What are SQL Queries?
SQL queries are powerful commands that facilitate communication with relational databases. Databases are crucial for storing, organizing, and retrieving vast amounts of information, making them an integral part of various applications and systems. The ability to interact with databases is a fundamental skill for individuals working with data, and SQL provides a standardized language for this purpose.
SQL queries encompass diverse components, incorporating clauses like WHERE, ORDER BY, and GROUP BY, offering users a methodical way to customize queries according to their specific requirements. These commands establish a structured framework for accessing and manipulating the extensive information housed within databases, forming the foundation for efficient data retrieval and management.
Components of SQL Queries:
- SELECT Statement:
The SELECT statement stands out as the primary tool for querying databases, granting users the ability to retrieve data from single or multiple tables. Its syntax, characterized by simplicity, renders it approachable for beginners while maintaining the potency necessary for handling intricate queries.
-- Basic SELECT statement
SELECT column1, column2 FROM table_name;
-- SELECT statement with WHERE clause
SELECT column1, column2 FROM table_name WHERE condition;
- Filtering Data with WHERE Clause:
The WHERE clause refines query results by imposing conditions. Logical operators (AND, OR) and comparison operators (<, >, =) enhance the WHERE clause's flexibility, enabling precise data retrieval.
-- INSERT statement
INSERT INTO table_name (column1, column2) VALUES (value1, value2);
-- UPDATE statement
UPDATE table_name SET column1 = value1 WHERE condition;
-- DELETE statement
DELETE FROM table_name WHERE condition;
Sorting Data with ORDER BY: ORDER BY brings a semblance of order to query results. Ascending (ASC) and descending (DESC) options provide control over the arrangement of data, catering to specific presentation requirements.
Advanced SQL Queries:
- JOIN Operations: Join operations allow the combination of data from multiple tables. INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN offer versatile options for merging datasets, providing a comprehensive view of interconnected information.
- Subqueries: Subqueries introduce a layer of complexity by embedding one query within another. This technique enhances query flexibility, enabling the retrieval of data based on conditions derived from another set of results.
- Aggregate Functions: Aggregate functions (AVG(), COUNT(), SUM()) play a pivotal role in statistical analysis. These functions offer insights into data trends, providing valuable information for decision-making.
Optimizing SQL Queries:
- Indexing: Indexing significantly impacts query performance by expediting data retrieval. Well-designed indexes enhance the efficiency of SELECT statements, contributing to a more responsive database.
- Normalization: Database normalization ensures that data is organized efficiently, minimizing redundancy and enhancing query performance. Normalized databases facilitate seamless querying by maintaining data integrity.
SQL Queries - Additional Aspects and Considerations
- Subqueries and Joins:
Explore the importance of subqueries in SQL and how they contribute to improving query functionality when incorporated into SELECT, FROM, and WHERE clauses.
Dive into the concept of joins in SQL, encompassing INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN, and understand how they facilitate the combination of data from multiple tables. Highlight scenarios where each type of join is most applicable.
-- Subquery in SELECT clause
SELECT column1, (SELECT column2 FROM another_table WHERE condition) FROM table_name;
-- INNER JOIN
SELECT column1, column2 FROM table1 INNER JOIN table2 ON table1.column = table2.column;
- Grouping and Aggregation:
Elaborate on the GROUP BY clause, illustrating how it is employed to group rows based on specified columns.
Introduce aggregate functions (SUM, AVG, COUNT, etc.) within the context of GROUP BY to perform calculations on grouped data.
-- GROUP BY and AVG
SELECT column1, AVG(column2) FROM table_name GROUP BY column1;
-- COUNT function
SELECT column1, COUNT(column2) FROM table_name GROUP BY column1;
- Nested Queries:
Provide examples and use cases for nested queries, demonstrating how these queries are embedded within other queries to achieve more complex operations.
-- Nested SELECT statement
SELECT column1 FROM table_name WHERE column2 IN (SELECT column3 FROM another_table WHERE condition);
- Transaction Management:
Touch upon the concept of transactions in SQL, emphasizing the importance of maintaining data integrity through COMMIT and ROLLBACK statements.
-- BEGIN TRANSACTION
BEGIN;
-- COMMIT
COMMIT;
-- ROLLBACK
ROLLBACK;
- Stored Procedures:
Introduce the concept of stored procedures, explaining how they encapsulate a series of SQL statements into a reusable and modular unit.
- Security Considerations:
Discuss best practices for securing SQL queries, including parameterized queries to prevent SQL injection attacks and the principle of least privilege.
-- Parameterized query to prevent SQL injection
DECLARE @input_value NVARCHAR(50);
SET @input_value = 'user_input';
SELECT column1, column2 FROM table_name WHERE column3 = @input_value;
- Optimization Techniques:
Provide insights into optimizing SQL queries for performance, addressing indexing, query execution plans, and other optimization strategies.
-- Creating an index
CREATE INDEX index_name ON table_name (column1, column2);
-- Viewing query execution plan
EXPLAIN SELECT column1, column2 FROM table_name WHERE condition;