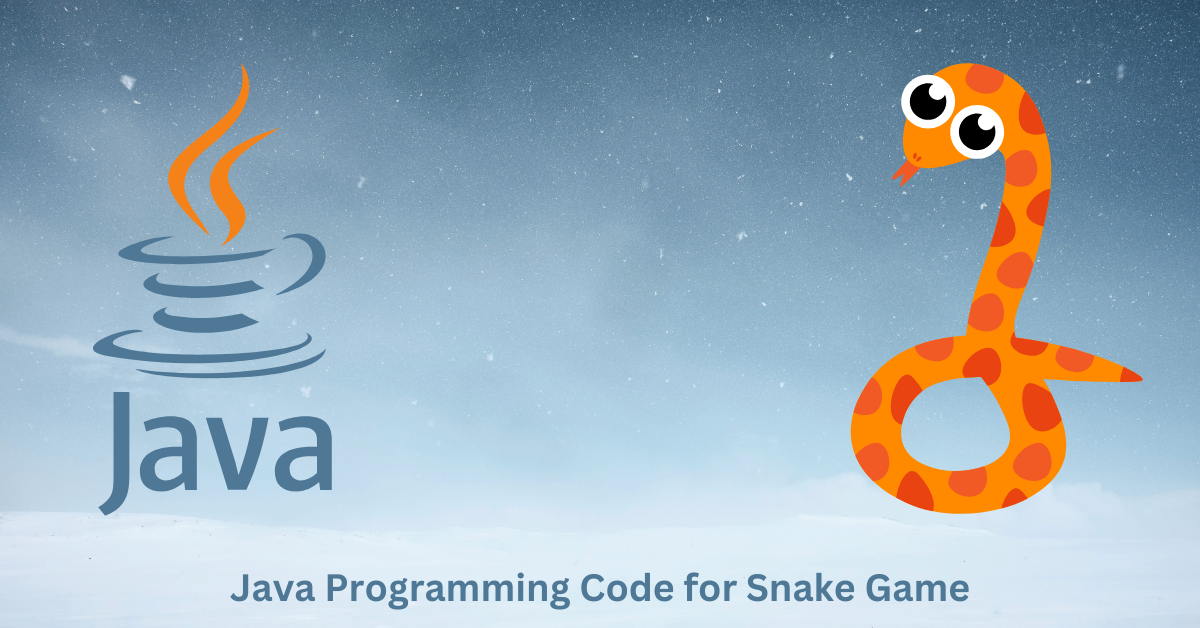
- 15th Jan 2024
- 18:01 pm
- Admin
The Programming Assignment Help website is one of the most popular Java helper online. We provide help with Java assignments, homework and projects. We also provide Java tutoring services to students looking to clear their doubts. Many students have come to us asking for Snake Game code in Java programming. We thought we can share a sample code which the student can modify as per his requirement.
What is a Snake Game?
Snake game is a classic, simple, and addictive game that can be developed in Java Programming. Users control a snake that grows with each eaten point, navigating a maze-like environment without hitting walls or its own tail.The game window will appear, and you can control the snake's direction using the arrow keys. The snake will grow longer each time it eats the blue food. If the snake collides with the window boundaries or itself, the game will end.
Java Code - Snake Game
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
public class SnakeGame extends JFrame implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private final int gridSize = 20;
private int rows, cols;
private int[] snakeX, snakeY;
private int snakeLength;
private int direction;
private int foodX, foodY;
private Timer timer;
public SnakeGame() {
setTitle("Snake Game");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
setSize(400, 400);
setLocationRelativeTo(null);
rows = getHeight() / gridSize;
cols = getWidth() / gridSize;
snakeX = new int[rows * cols];
snakeY = new int[rows * cols];
snakeLength = 1;
direction = KeyEvent.VK_RIGHT;
addKeyListener(this);
setFocusable(true);
timer = new Timer(100, this);
timer.start();
spawnFood();
}
public void spawnFood() {
Random rand = new Random();
foodX = rand.nextInt(cols) * gridSize;
foodY = rand.nextInt(rows) * gridSize;
}
public void move() {
for (int i = snakeLength - 1; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
switch (direction) {
case KeyEvent.VK_UP:
snakeY[0] -= gridSize;
break;
case KeyEvent.VK_DOWN:
snakeY[0] += gridSize;
break;
case KeyEvent.VK_LEFT:
snakeX[0] -= gridSize;
break;
case KeyEvent.VK_RIGHT:
snakeX[0] += gridSize;
break;
}
checkCollision();
checkFood();
}
public void checkCollision() {
if (snakeX[0] < 0 || snakeX[0] >= getWidth() || snakeY[0] < 0 || snakeY[0] >= getHeight()) {
gameOver();
}
for (int i = snakeLength - 1; i > 0; i--) {
if (snakeX[0] == snakeX[i] && snakeY[0] == snakeY[i]) {
gameOver();
}
}
}
public void checkFood() {
if (snakeX[0] == foodX && snakeY[0] == foodY) {
snakeLength++;
spawnFood();
}
}
public void gameOver() {
timer.stop();
JOptionPane.showMessageDialog(this, "Game Over!\nYour score: " + (snakeLength - 1));
System.exit(0);
}
@Override
public void actionPerformed(ActionEvent e) {
move();
repaint();
}
@Override
public void paint(Graphics g) {
super.paint(g);
g.clearRect(0, 0, getWidth(), getHeight());
// Draw snake
for (int i = 0; i < snakeLength; i++) {
g.setColor(i == 0 ? Color.GREEN : Color.RED);
g.fillRect(snakeX[i], snakeY[i], gridSize, gridSize);
}
// Draw food
g.setColor(Color.BLUE);
g.fillRect(foodX, foodY, gridSize, gridSize);
}
@Override
public void keyPressed(KeyEvent e) {
int newDirection = e.getKeyCode();
if ((newDirection == KeyEvent.VK_UP && direction != KeyEvent.VK_DOWN) ||
(newDirection == KeyEvent.VK_DOWN && direction != KeyEvent.VK_UP) ||
(newDirection == KeyEvent.VK_LEFT && direction != KeyEvent.VK_RIGHT) ||
(newDirection == KeyEvent.VK_RIGHT && direction != KeyEvent.VK_LEFT)) {
direction = newDirection;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
public static void main(String[] args) {
SwingUtilities.invokeLater(() -> {
new SnakeGame().setVisible(true);
});
}
}
Blog Author - Janhavi Gupta
Janhavi Gupta is a highly skilled Java programmer with a passion for developing robust and efficient software solutions. With a strong foundation in Java programming, she excels in designing and implementing scalable applications. Janhavi is adept at solving complex coding challenges and has a keen eye for detail. Her expertise extends to various Java frameworks, ensuring the delivery of high-quality, performance-optimized software.