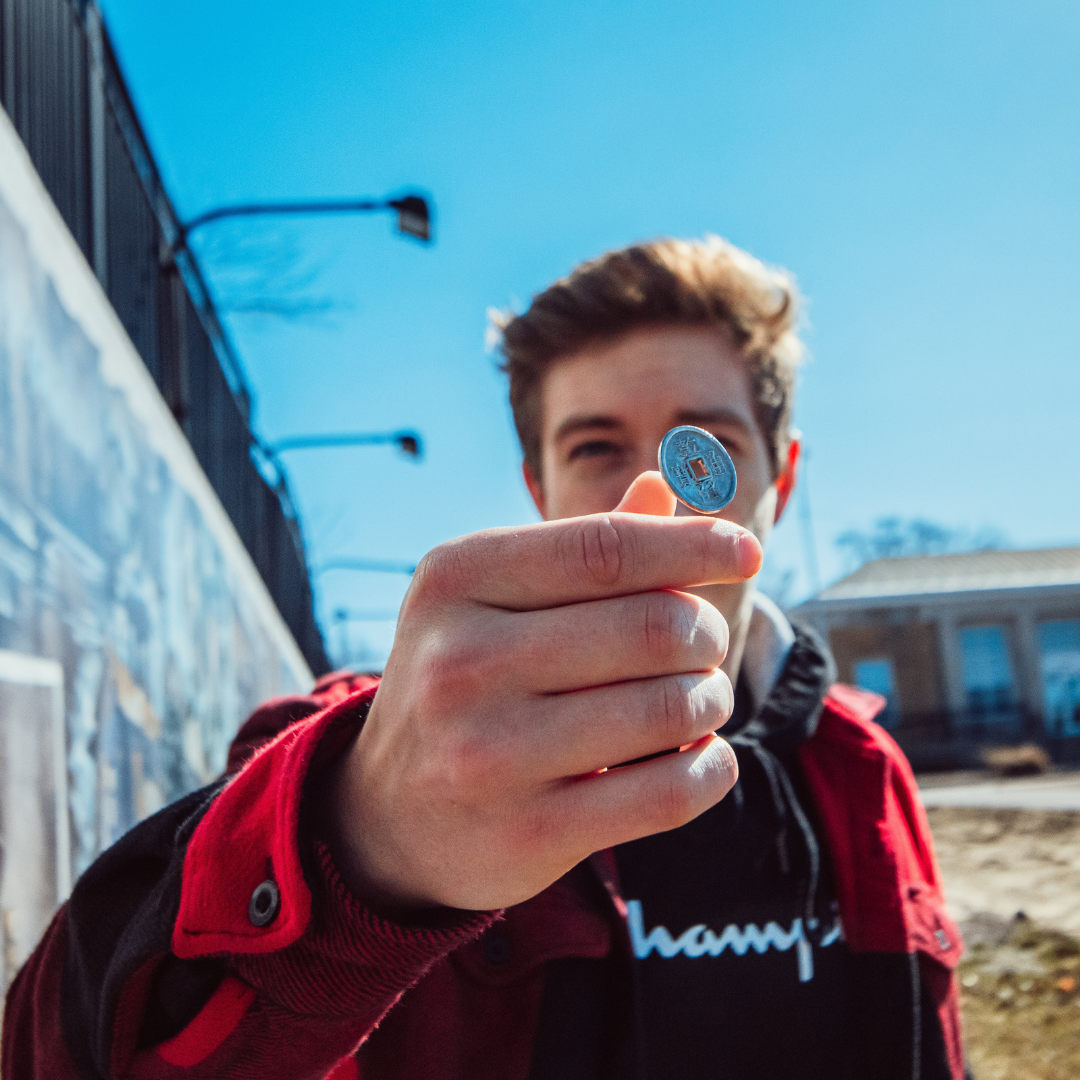
- 2nd Apr 2023
- 06:46 am
- Admin
C++ Homework Help
C++ Homework Question
C++ Simulating Coin Toss (C++ Functions and Loops) - Write a function named coinToss that simulates the tossing of a coin. When you call the function, it should generate a random number in the range of 1 through 2. If the random number is 1, the function should display "heads", if it is 2, it should display "tails". Demonstrate the function in a program that asks the user how many times the coin should be tossed, and then simulates the tossing of the coin that number of times.
C++ Homework Solution
#include
#include // for rand() and srand()
#include // for time()
using namespace std;
// function to simulate a coin toss
void coinToss() {
int num = rand() % 2 + 1; // generate random number 1 or 2
if (num == 1) {
cout << "heads" << endl;
} else {
cout << "tails" << endl;
}
}
int main() {
srand(time(0)); // seed the random number generator with the current time
int numTosses;
cout << "How many times do you want to toss the coin? ";
cin >> numTosses;
for (int i = 0; i < numTosses; i++) {
cout << "Toss " << i+1 << ": ";
coinToss();
}
return 0;
}
The coinToss() function uses rand() to generate a random number in the range of 1 through 2, and then displays "heads" if the number is 1 and "tails" if the number is 2. In the main() function, the user is prompted for how many times they want to toss the coin, and then a loop calls the coinToss() function that number of times.