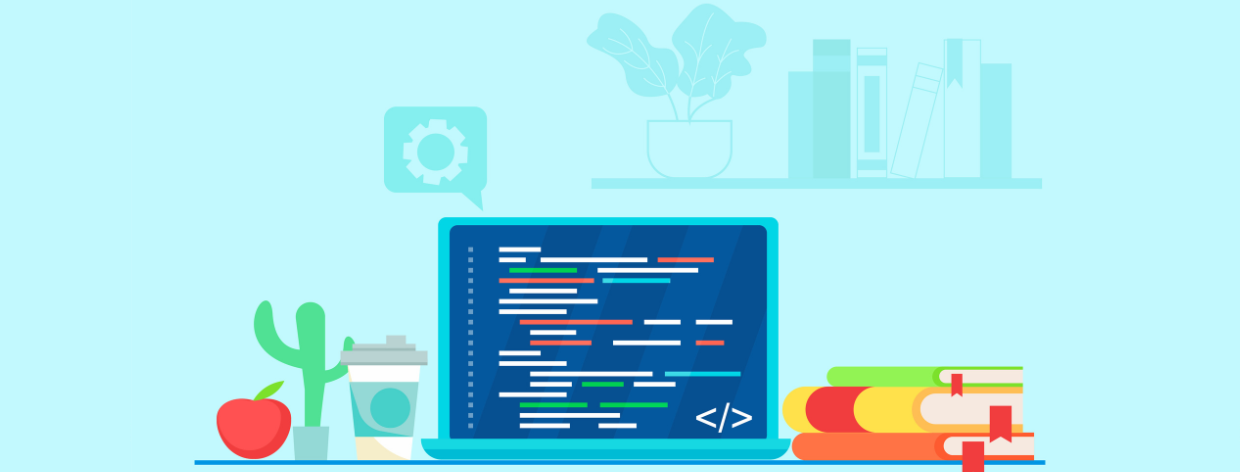
- 14th Feb 2024
- 16:50 pm
- Adan Salman
In the world of Python data manipulation, understanding how to effectively convert sets to lists is crucial for various tasks. While both data structures hold values, their core characteristics differ, making specific situations require the conversion from one to the other. Let's delve into the world of sets and lists, exploring the need for conversion and the different methods available.
Sets: Imagine a collection of unique items, like a basket of distinct fruits. That's what sets represent in Python. They guarantee no duplicates, ensuring each element exists only once. This focus on uniqueness makes them excellent for tasks like removing duplicates from data or checking membership efficiently.
Lists: Now, picture a shopping list where order matters. That's the essence of lists. They store elements in a specific sequence, allowing you to access and manipulate them based on their position. This ordered nature makes them ideal for tasks like iterating through data or preserving the original order during processing.
Why the Conversion?
While both sets and lists are powerful tools, certain scenarios demand the versatility of lists. Here are some common reasons for set-to-list conversion:
- Duplication Removal: Eliminate identical elements from a set to get a unique list representation.
- Subsetting and Manipulation: Work with specific portions of a set by converting it to a list and applying indexing or slicing.
- Integration with Libraries: Some libraries or APIs might expect data in list format for analysis or visualization.
- Temporary Processing: Convert a set to a list for calculations or transformations, preserving the original set for future use.
Methods for Converting Sets to Lists
Sets and lists are two fundamental data structures in Python, each serving different purposes. Each set has its own definition and purpose. A set collects elements uniquely, without any order, but a list collects elements in an order with duplication. Now, while working with both, oftentimes you will have a set and need to convert it to a list. Here are the methods you can use, with some of the pros and cons of each:
- Direct Conversion:
The simplest way to convert a set to a list is using the list() constructor. It takes any iterable (like a set) as input and returns a new list containing its elements.
my_set = {1, 2, 3}
my_list = list(my_set)
print(my_list) # Output: [1, 2, 3] (order might be different)
You recall that the result is a set; hence, in list comprehension, the order of the final list might not be expected, more so with versions of Python earlier than 3.7. In general, since Python 3.7, order reflects insertion order.
- Explicit Looping:
For more control over the conversion process and the resulting list's order, you can iterate through the set elements using a loop and add them to a list. You can achieve this using two methods:
a) for loop:
my_set = {4, 5, 6}
my_list = []
for element in my_set:
my_list.append(element)
print(my_list) # Output: [4, 5, 6] (order reflects iteration)
b) List comprehension:
my_set = {7, 8, 9}
my_list = [element for element in my_set]
print(my_list) # Output: [7, 8, 9] (order reflects iteration)
Both methods offer flexibility and control over the order. You can modify the loop or list comprehension to filter or transform elements during conversion.
- sorted() Function:
Want a guaranteed ordered list? The sorted() function sorts elements and returns a new list. This method preserves the natural order of the elements (e.g., numerical, alphabetical).
my_set = {"apple", "banana", "cherry"}
my_list = sorted(my_set)
print(my_list) # Output: ["apple", "banana", "cherry"] (alphabetical order)
While providing order, sorted() might be less efficient for large sets due to the sorting overhead.
- Using map() Function:
The map() function applies a function to each element in an iterable. You can use it with an anonymous function or lambda expression to convert each set element to a new element in the list.
my_set = {10, 20, 30}
my_list = list(map(lambda x: x * 2, my_set))
print(my_list) # Output: [20, 40, 60] (doubling each element)
This method is versatile for custom transformations during conversion. However, using it for simple conversions might be less readable than other methods.
- List Comprehension:
The map() function applies a function to each element in an iterable. You can use it with an anonymous function or lambda expression to convert each set element to a new element in the list.
my_set = {10, 20, 30}
my_list = list(map(lambda x: x * 2, my_set))
print(my_list) # Output: [20, 40, 60] (doubling each element)
This method is versatile for custom transformations during conversion. However, using it for simple conversions might be less readable than other methods.
- Unpacking with *set,:
This advanced technique uses the unpacking operator (*) to expand a set as arguments while creating a new list. You can add additional elements to the list during conversion.
my_set = {15, 16, 17}
my_list = [0, *my_set, 18]
print(my_list) # Output: [0, 15, 16, 17, 18] (adding elements)
This method is less common but useful for specific scenarios where you want to modify the list during conversion.
- Using copy.copy() or copy.deepcopy():
For cautious conversions, you can use these functions from the copy module to create a shallow or deep copy of the set as a list.
a. copy.copy(): Creates a shallow copy, where changes to the list directly affect the original set, and vice versa. Use this when you want modifications to reflect in both entities.
import copy
my_set = {19, 20, 21}
my_list = copy.copy(my_set)
my_list.append(22)
print(my_set) # Output: {19, 20, 21, 22} (set is modified due to shallow copy)
b. copy.deepcopy(): Creates a deep copy, where changes to the list don't affect the original set, and vice versa. Use this when you want independent manipulation.
my_set = {23, 24, 25}
my_list = copy.deepcopy(my_set)
my_list.append(26)
print(my_set) # Output: {23, 24, 25} (set remains unchanged due to deep copy)
- Clearing the Set:
After converting the set to a list, consider using the clear() method to remove all elements from the set. This helps manage memory, especially for large sets.
my_set = {27, 28, 29}
my_list = list(my_set)
my_set.clear()
print(my_set) # Output: set() (set is empty now)
Remember, clearing the set permanently removes its elements, so use this method only if you don't need the original set anymore.
Choosing the Right Method:
The best method for converting sets to lists depends on your specific needs:
- For quick and simple conversion, use list() or explicit looping.
- For guaranteed order, use sorted().
- For custom transformations, consider map() or list comprehension.
- For modifying the list during conversion, use unpacking with *set,.
- For shallow or deep copies, use copy.copy() or copy.deepcopy().
- If memory usage is a concern, consider clearing the set after conversion.
By understanding these methods and their considerations, you can confidently choose the best approach for your Python projects.
Advanced Techniques and Considerations
While the basic methods for converting sets to lists in Python are straightforward, several advanced techniques and considerations come into play for specific scenarios:
Preserving Order:
- Insertion order: If you want the resulting list to reflect the order elements were added to the set (especially relevant in Python 3.7+), you have two options:
- Explicit looping: Iterating through the set using a for loop or list comprehension will maintain the insertion order by adding elements sequentially to the list.
- copy.copy(): Although creating a shallow copy doesn't guarantee order preservation in theory, its implementation often reflects the insertion order in practice. However, rely on this behavior with caution.
- Sorted order: Use the sorted() function if you require a specifically ordered list. You can sort based on the elements' natural order (numerical, alphabetical) or define a custom sorting function. Remember that sorting introduces overhead, potentially impacting performance for large datasets.
Performance Considerations:
- Simple conversions: When speed is crucial, especially for large sets, direct conversion with list() or explicit looping is generally efficient. Avoid map() and sorted() unless strictly necessary.
- Custom transformations: If you need complex transformations during conversion, list comprehension offers a good balance between readability and performance. However, for highly customized logic, map() might be more performant with proper optimization.
Error Handling:
- Non-hashable elements: Sets only accept hashable elements like numbers, strings, and tuples. If your set contains non-hashable elements like lists or dictionaries, attempting conversion will raise a TypeError. Ensure proper data cleansing before conversion.
- Memory limitations: Converting large sets might strain memory, especially when using methods like sorted() that create temporary copies. Be mindful of your system resources and adjust your approach accordingly.
Context-Specific Approaches:
The optimal method depends heavily on the data and the desired outcome:
- Simple data structure: For small sets and basic conversions, direct methods like list() or looping are perfectly suitable.
- Preserving insertion order: If the order matters, explicit looping or copy.copy() (with caution) are your go-to options.
- Specific sorting: When dealing with large datasets and requiring sorted lists, evaluate the trade-off between sorted()'s guaranteed order and its potential performance impact.
- Complex transformations: List comprehension excels at combining filtering, transformations, and conversion in a single, readable statement, making it ideal for these scenarios.
Applications of Converting Sets to Lists in Python
Converting sets to lists in Python goes beyond mere data representation. Here are some key applications across different domains:
Data Cleaning and Deduplication:
- Removing duplicates: Working with data often involves eliminating redundant entries. Converting a set to a list ensures unique elements, effectively tackling duplicates.
- Identifying unique values: Analyzing datasets often requires finding distinct values. Transforming a set to a list facilitates iterating through and manipulating unique elements for further analysis.
Sorting and Reordering:
- Custom sorting: Sets themselves are unordered, but converting to a list allows you to apply custom sorting logic (e.g., based on specific attributes) using functions like sorted() or list comprehension.
- Rearranging data: Sometimes, data needs restructuring based on specific criteria. Converting to a list empowers you to reorder elements using indexing, slicing, or list methods like append() and insert().
Data Transformation and Visualization:
- Applying transformations: Converting sets to lists enables applying transformations to each element using map or list comprehension. This is vital for tasks like data scaling, normalization, or feature engineering.
- Preparing data for visualization: Plotting libraries often require ordered data structures. Converting sets to lists facilitates creation charts, graphs, and other visual representations for effective data exploration.
Data Validation and Verification:
- Checking for specific elements: Converting to a list allows efficient searching for specific values within the set using the in operator or list methods like index(). This helps verify data integrity and identify potential anomalies.
- Creating frequency tables: Counting the occurrences of unique elements is crucial for understanding data distribution. Converting to a list allows building frequency tables using techniques like counting occurrences within the list.
Integration with other Data Structures:
- Combining sets with lists: Sometimes, you might need to merge or process data alongside existing lists. Converting sets to lists enables seamless integration and manipulation within complex data structures.
- Feeding lists to algorithms: Many algorithms, like sorting algorithms or search algorithms, operate on ordered data structures. Converting sets to lists enables feeding them as input for various computational tasks.
By understanding these applications and choosing the appropriate conversion method, you can unlock the potential of sets and lists in your Python projects for diverse data management and analysis tasks.