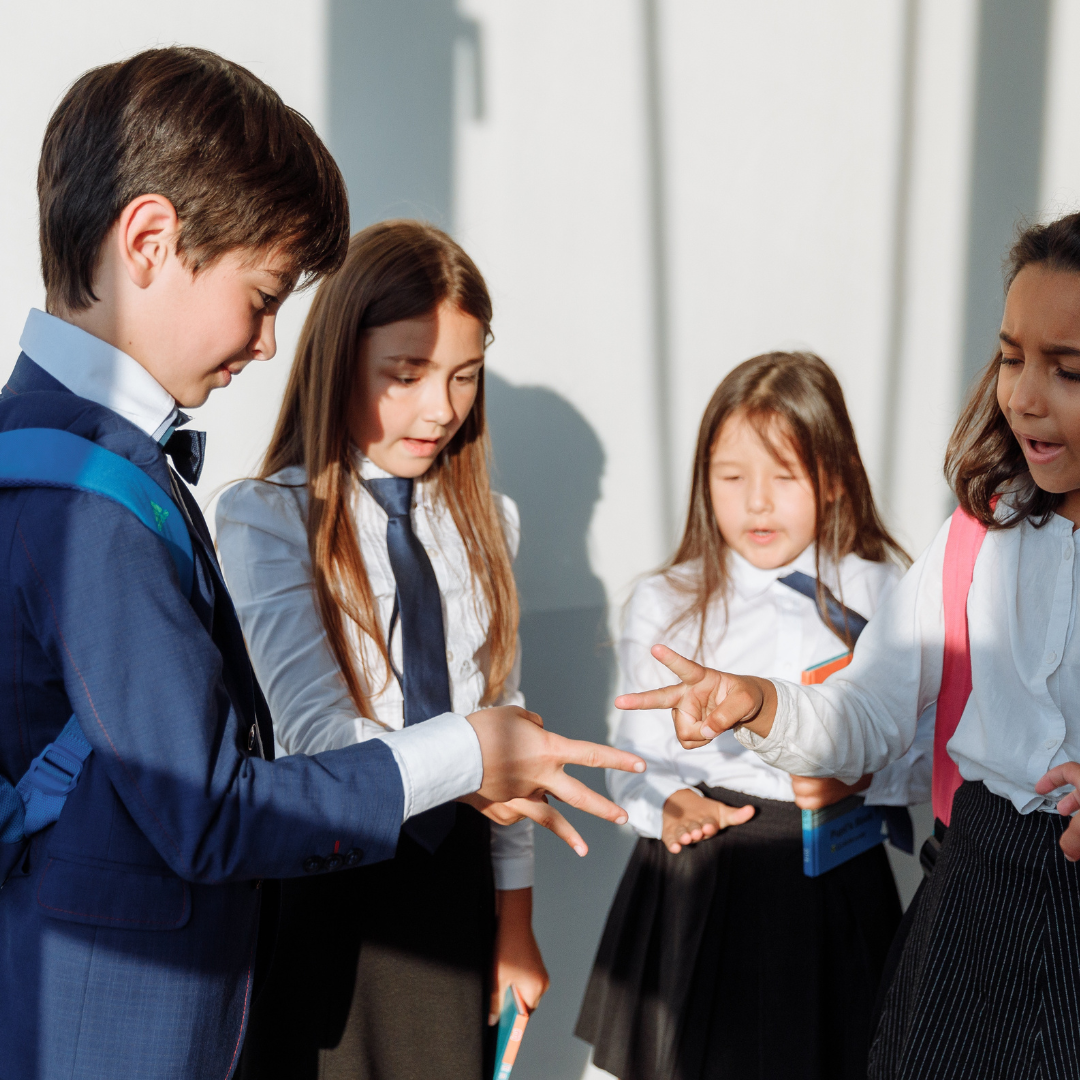
- 3rd Apr 2023
- 09:49 am
Java Homework Question
Rock Paper Scissors (Enum, Inheritance, Java OOP) - Design and implement an application that plays the Rock Paper Scissors game against the computer. The program should randomly choose one of the three options, then prompt the user's selection. At that point, the program reveals both choices and prints a statement indicating if the user won, the computer won or if it is a tie. Continue playing until user chooses to stop, then print the number of wins, losses, and ties.
Java Homework Solution
Sure, here's an implementation of the Rock Paper Scissors game in Java using enums, inheritance, and OOP principles:
import java.util.Random;
import java.util.Scanner;
enum Choice {
ROCK, PAPER, SCISSORS;
}
class Player {
private String name;
private int score;
public Player(String name) {
this.name = name;
this.score = 0;
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
public void incrementScore() {
score++;
}
public void resetScore() {
score = 0;
}
}
class Computer extends Player {
public Computer() {
super("Computer");
}
public Choice generateChoice() {
Random random = new Random();
return Choice.values()[random.nextInt(3)];
}
}
public class RockPaperScissors {
private static Scanner scanner = new Scanner(System.in);
public static void main(String[] args) {
Player player = new Player("Player");
Computer computer = new Computer();
boolean continuePlaying = true;
while (continuePlaying) {
Choice playerChoice = getPlayerChoice();
Choice computerChoice = computer.generateChoice();
System.out.printf("Player chose %s%n", playerChoice);
System.out.printf("Computer chose %s%n", computerChoice);
int result = getResult(playerChoice, computerChoice);
if (result == 1) {
System.out.printf("%s wins!%n", player.getName());
player.incrementScore();
} else if (result == -1) {
System.out.printf("%s wins!%n", computer.getName());
computer.incrementScore();
} else {
System.out.println("It's a tie!");
}
System.out.printf("%s: %d | %s: %d | Ties: %d%n",
player.getName(), player.getScore(),
computer.getName(), computer.getScore(),
getTotalTies(player, computer));
continuePlaying = askToContinue();
}
System.out.println("Thanks for playing!");
}
private static Choice getPlayerChoice() {
System.out.print("Enter your choice (rock, paper, scissors): ");
String input = scanner.nextLine().toUpperCase();
return Choice.valueOf(input);
}
private static int getResult(Choice playerChoice, Choice computerChoice) {
if (playerChoice == computerChoice) {
return 0;
} else if (playerChoice == Choice.ROCK && computerChoice == Choice.SCISSORS ||
playerChoice == Choice.PAPER && computerChoice == Choice.ROCK ||
playerChoice == Choice.SCISSORS && computerChoice == Choice.PAPER) {
return 1;
} else {
return -1;
}
}
private static boolean askToContinue() {
System.out.print("Do you want to continue playing? (y/n): ");
String input = scanner.nextLine().toLowerCase();
return input.equals("y") || input.equals("yes");
}
private static int getTotalTies(Player player1, Player player2) {
int totalTies = 0;
totalTies += 3 - player1.getScore();
totalTies += 3 - player2.getScore();
return totalTies;
}
}
This implementation of the Rock Paper Scissors game has an enum called Choice that represents the three options - rock, paper, and scissors.
We also have two classes: Player and Computer. The Player class has a name and a score, as well as methods to increment and reset the score.
The Computer class is a subclass of the Player class, which means it inherits all of the properties and methods of the Player class. The Computer class has an additional method called getMove, which returns a random move (rock, paper, or scissors) for the computer to play.
In the main method of the RockPaperScissors class, we create instances of the Player and Computer classes and prompt the user to enter their name. We then use a while loop to allow the user to play multiple rounds of the game.
For each round, we prompt the user to enter their move (rock, paper, or scissors) and use the valueOf method of the Choice enum to convert the user's input to an enum value.
We then call the getMove method of the Computer class to get the computer's move, and use an if-else block to determine the winner of the round. If the user wins, we increment their score using the incrementScore method of the Player class.
At the end of each round, we print the result (tie, user wins, or computer wins) and the current scores of the user and computer. We continue playing rounds until the user enters "quit", at which point we print the final scores and exit the program.