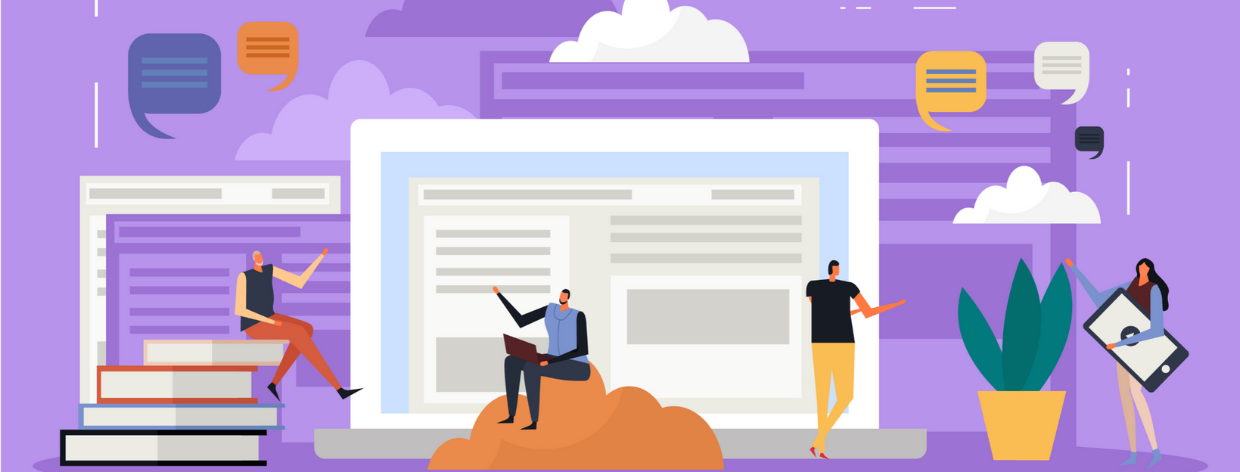
- 9th Feb 2024
- 15:58 pm
- Admin
Extracting information from text files is fundamental in Python, driving numerous tasks like data analysis, log parsing, and configuration management. Reading files line by line allows us to process data incrementally, efficiently handling large files and avoiding memory overload.
Popular use cases include:
- Data processing: Cleaning and transforming CSV files, extracting features from log files, or loading datasets line by line.
- Configuration parsing: Reading settings from INI files, parsing JSON configurations, or extracting environment variables one at a time.
- Text analysis: Analyzing sentiment in reviews, performing line-by-line text processing, or building word frequency dictionaries.
Basic Methods of Read Files Line by Line in Python
Let's delve into the core methods for reading files line by line in Python:
- open() Function:
This versatile function serves as the gateway to files. Use open("filename.txt", "r") to open a file in read mode, returning a file object. Remember to close the file later to release resources.
- for Loop and readline():
A classic combination! Iterate through the file object line by line using a for loop:
with open("data.txt", "r") as file:
for line in file:
# Process each line (e.g., print(line.strip()))
readline() reads a single line, including the trailing newline character. Remember to remove it with .strip() if needed.
- readlines():
This method reads all lines in the file into a list:
with open("config.json", "r") as file:
lines = file.readlines()
# Access specific lines later: line_content = lines[2]
For smaller files, this method efficiently stores all lines for later processing.
Pitfalls to Avoid:
- Trailing newlines: readline() includes the newline, so .strip() might be necessary.
- Unexpected formats: Ensure the file format (e.g., CSV, JSON) is compatible with your processing logic.
Efficient Iteration with "with Statement"
Embrace the magic of the with statement for smooth file handling! This powerful construct automatically closes the file when the code block finishes, ensuring proper resource management and avoiding potential errors or leaks.
Combine with and for loops for concise and efficient line-by-line reading:
with open("data.csv", "r") as file:
for line in file:
# Process each line
No more manual file.close() calls! This approach streamlines your code and guarantees proper file closure even in case of exceptions.
Beyond simplicity, with excels in error handling. If an exception occurs within the block, the file still gets closed. You can use try-except within the with for graceful exception handling, making your code robust and reliable.
Advanced Line-by-Line Reading Techniques
For power users, Python offers advanced methods for nuanced file traversal:
- Precise targeting: itertools.islice() empowers you to read specific line ranges, perfect for skipping headers, analyzing sections, or even reading lines in reverse order.
- Custom line processing: Unchain the flexibility of lambda functions or generator expressions to perform on-the-fly data cleaning, transformations, or filtering as each line is encountered.
- Large file efficiency: When dealing with giants, explore lazy loading techniques to read only the necessary portions or leverage generators for memory-efficient processing, ensuring smooth handling of even the most colossal files.
Handling Different File Formats
Text files are just the beginning of Python's file-handling prowess. Mastering different formats expands your horizons:
- Encoding Maestro: Conquer garbled text by understanding encoding. Specify the correct encoding parameter in open() for non-ASCII characters. Remember, consistency is key!
- Binary Byte Dance: Binary files require different moves. Open them in binary mode ("rb") and read data in manageable chunks (e.g., 1024 bytes) for larger files. Interpret byte data based on the specific format (images, audio, etc.).
- Specialized Library Leverage: Libraries like csv effortlessly handle comma-separated values, while json parses JSON files with ease. Utilize their built-in functionalities for efficient access and processing.
Error Handling and Troubleshooting
Reading files isn't always smooth sailing. Robust error handling is crucial to prevent unexpected crashes and ensure data integrity.
Common foes include:
- FileNotFoundError: The file simply doesn't exist. Check file paths and permissions.
- PermissionError: You lack read access. Verify user permissions and file ownership.
Embrace try-except blocks for graceful error handling:
try:
with open("my_file.txt", "r") as file:
# Process lines
except FileNotFoundError:
print("File not found!")
except PermissionError:
print("Insufficient permissions to access file.")
This approach catches specific errors, provides informative messages, and prevents program termination.
Remember to log errors for further analysis and implement appropriate recovery mechanisms. Embrace error handling as a safety net, ensuring your file reading operations run smoothly and reliably.