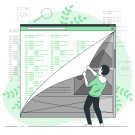
- 2nd Nov 2023
- 00:20 am
Python's "contains" method is a versatile tool for string manipulation. It determines whether a specific substring is present within a given string, returning a simple true or false result. This functionality is invaluable for various programming tasks, from validating user inputs to text analysis and content filtering.
What is Python String Contains?
In the realm of Python, the "contains" method stands as a fundamental tool for manipulating strings. It serves the crucial role of determining whether a specific substring exists within a given string. This method operates by meticulously scanning the original string to assess if the desired substring is present. The outcome is binary: a resolute "True" when the substring is detected, and a definitive "False" when it eludes identification.
While this function may appear deceptively simple, its true power emerges in a wide range of applications. Python's string contains become invaluable when you need to validate user inputs, hunt for specific keywords in a body of text, sift through data according to predefined patterns, or unearth meaningful insights from extensive textual content. Moreover, it serves as a foundational element for crafting intricate search algorithms, content retrieval systems, and handling web form inputs. With its straightforward yet highly effective nature, Python's string contains has secured its place in the toolkit of Python programmers, from novices to seasoned developers.
How Does Python String Contains Work?
Python's contains method is a workhorse for string manipulation. It operates by systematically analyzing a given string to determine whether a designated substring is present. This process begins with the method iterating through the original string, character by character, and checking if the substring matches a segment of the string. The search is exact and case-sensitive, meaning that it distinguishes between uppercase and lowercase characters. If a match is detected during the iteration, the method returns True. However, if the entire string is traversed without finding the substring, it returns False.
This straightforward yet powerful mechanism provides a versatile way to perform string validation and data extraction. The ability to discern the existence of a particular substring within a string is fundamental in various programming scenarios, from verifying user input in web forms to implementing search algorithms. Python's contains method's efficiency and simplicity make it a valuable tool in the programmer's arsenal for tasks requiring string evaluation and validation.
Implementation of Python String Contains
Python's contains method, often implemented with the in keyword, provides a straightforward way to check for the existence of a specific substring within a given string. The implementation is efficient and simple, making it a popular choice for tasks that require substring validation.
To use Python String Contains, you only need to provide the target string and the substring you want to locate. The method then performs a meticulous scan through the target string, character by character, comparing it with the specified substring. If an exact match is found at any point during this process, Python String Contains returns True, indicating the presence of the substring. Conversely, if the entire string is traversed without a match, it returns False.
This simplicity and efficiency make Python String Contains a powerful tool for string manipulation and data validation.
Python String Contains Code Snippets
Let's see how to use the contains method in Python, Java, and C++:
- Python:
original_string = "This is an example string."
substring = "example"
result = original_string.__contains__(substring)
print(result)
# Output: True
- Java:
String originalString = "This is an example string.";
String substring = "example";
boolean result = originalString.contains(substring);
System.out.println(result); # Output: true
- C++:
#include
#include
using namespace std;
int main() {
string originalString = "This is an example string.";
string substring = "example";
bool result = originalString.find(substring) != string::npos;
cout << result << endl; // Output: 1 (true)
return 0;
}
Advantages of Python String Contains
Python's contains method offers several advantages that make it a preferred choice for string manipulation. These advantages include:
- Simplicity: Python string contains is easy to use and understand, making it accessible to both beginners and experienced programmers. Its straightforward syntax simplifies the process of substring validation.
- Efficiency: The method efficiently scans through the original string to find the specified substring, resulting in quick and reliable validation. This efficiency is crucial for real-time applications and data processing.
- Flexibility: Python string contains is versatile and applicable in various scenarios. Whether you need to check user input, filter data based on specific patterns, or extract information from large text documents, this method can handle a wide range of text-processing tasks.
- Compatibility: Python's wide adoption and cross-platform support ensure that the contains method is available and functional across different operating systems and environments, making it highly reliable for diverse projects.
- Integration: It can be easily integrated into more complex algorithms and processes, providing a building block for advanced search and text analysis tasks.
Disadvantages/Limitations of Python String Contains
While Python string contains is a valuable tool for string manipulation, it has its share of limitations and disadvantages:
- Case Sensitivity: By default, the Python string contains is case-sensitive. It distinguishes between uppercase and lowercase characters, which means it may not detect a substring if the case doesn't match exactly. For case-insensitive searches, additional coding may be necessary.
- Exact Matches: Python string contains searches for exact matches of the specified substring. It does not handle more complex pattern matching or regular expressions. If your task involves complex pattern recognition, you may need to explore other methods or libraries.
- Limited to Single Substring: This method is designed to find a single substring within a string. If you need to search for multiple substrings or perform more intricate text analysis, you may require additional scripting and looping.
- Position Information: Python string contains does not provide information about the position of the found substring within the string. If you need to know the index or position of the substring, additional coding is necessary.
Applications of Python String Contains
Python's contains method finds its utility in various practical applications, making it a versatile tool for string manipulation and data validation. Some of the key applications include:
- Data Validation: Python string contains is invaluable for verifying and validating user inputs in web forms and applications. It ensures that specific patterns, keywords, or substrings are present or absent, preventing invalid or harmful data from entering a system.
- Text Processing: In the realm of text analysis, this method is frequently used to extract relevant information from extensive text data. Whether you're parsing documents, searching for keywords, or categorizing content, Python string contains simplifies the process.
- Search Algorithms: It serves as a fundamental building block for developing search algorithms. Search engines, content retrieval systems, and database queries use this method to locate specific terms or phrases within documents, enabling efficient and accurate search results.
- Content Filtering: When implementing content filtering mechanisms, Python string contains is crucial for identifying and blocking unwanted content, such as spam, profanity, or prohibited keywords. It ensures that content adheres to predefined guidelines.
- Pattern Matching: Python string contains can be used to detect specific patterns within strings, aiding in tasks such as regular expression matching and the extraction of structured data from unstructured text.