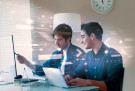
- 1st Nov 2023
- 15:24 pm
In Python programming python operators are symbols or special keywords that perform various operations on data values or variables. They include arithmetic operators for math, comparison operators to compare values, and logical operators for combining conditions, among others. Operators are fundamental for data manipulation and control flow in Python programming.
In this blog, we will look into different types of Python operators.
Types of Operators in Python
-
Arithmetic Operators
Arithmetic operators in Python are used to perform mathematical operations on numeric values. They include addition (+), subtraction (-), multiplication (*), division (/), modulus (%), and exponentiation (**).
Addition (+): It combines two values to produce their sum. Example:- 5 + 3 equals 8.
Subtraction (-): It subtracts the right operand from the left operand.
Example:- 10 - 4 results in 6.
Multiplication (*): This operator multiplies two values together. Example:- 6 * 7 equals 42.
Division (/): It divides the left operand by the right operand.
Example:- 15 / 3 equals 5.
There are two types of division operators:
- Float Division (/): The Float division operator / performs floating-point division, which means it returns a floating-point number (a number with a decimal point) even if the division results in a whole number. For example:
# python program to demonstrate the use of "/"
print(15/5)
print(100/2)
print(-30/2)
print(20.0/2)
Output:
3.0
50.0
-15.0
10.0
- Floor Division (//): The floor division operator // performs integer division, and it returns the largest integer that is less than or equal to the result of the division. It essentially truncates the decimal part. For example:
# python program to demonstrate the use of "//"
print(100//3)
print (-40//2)
print (5.0//2)
print (-5.0//2)
Output:
33
-20
2.0
-3.0
These two division operators are used in Python depending on the desired output. Standard division is used when you want a floating-point result, floor division is used when you want an integer result without rounding up.
Modulus (%): This operator returns the remainder of the division of the left operand by the right operand. 10 % 3 equals 1, as 10 divided by 3 leaves a remainder of 1.
Exponentiation ()**: It raises the left operand to the power of the right operand. 2 ** 3 equals 8 because 2^3 is 8.
Precedence of Arithmetic Operators in Python
The precedence of arithmetic operators in Python follows the standard order of operations in mathematics. Here's a list of arithmetic operators in order of precedence, from highest to lowest:
Exponentiation (**): This operator has the highest precedence and is evaluated first.
Multiplication (*), Division (/), Floor Division (//), and Modulus (%): These operators have the same precedence and are evaluated from left to right.
Addition (+) and Subtraction (-): These operators also have the same precedence and are evaluated from left to right.
You can use parentheses to override the default precedence and explicitly specify the order of operations. Operations within parentheses are always evaluated first. For example:
Example showing how different Arithmetic Operators in Python work:
# Examples of Arithmetic Operator
a = 100
b = 4
# Addition of numbers
add = a + b
# Subtraction of numbers
sub = a - b
# Multiplication of a number
mul = a * b
# Modulo of both numbers
mod = a % b
# print results
print(add)
print(sub)
print(mul)
print(mod)
Output:
104
96
400
25
-
Comparison Operators:
Comparison operators in Python are used to compare values and return either True or False. They allow you to make decisions and control the flow of your programs based on conditions. Here are some common comparison operators:
== (Equal): Checks if two values are equal.
a = 5
b = 5
result = (a == b) # True
!= (Not Equal): Checks if two values are not equal.
Example
a = 5
b = 7
result = (a != b) # True
< (Less Than): Checks if the left operand is less than the right operand.
Example
a = 5
b = 7
result = (a < b) # True
> (Greater Than): Checks if the left operand is greater than the right operand.
An example of Comparison Operators in Python.
x = 10
y = 5
# Equal to
print(x == y)
# Output: False
# Not equal to
print(x != y)
# Output: True
# Greater than
print(x > y)
# Output: True
# Less than
print(x < y)
# Output: False
# Greater than or equal to
print(x >= y)
# Output: True
# Less than or equal to
print(x <= y)
# Output: False
-
Logical Operators:
Logical operators in Python are used to perform logical operations on values or conditions. They are often used to combine or manipulate conditions. Common logical operators include:
and (Logical AND): Returns True if both conditions are true.
Example
x = True
y = False
result = (x and y) # False
or (Logical OR): Returns True if at least one condition is true.
Example
x = True
y = False
result = (x or y) # True
not (Logical NOT): Returns the opposite of the condition.
Code shows how to implement Logical Operators in Python:
# Define two boolean variables
x = True
y = False
# Logical AND
result_and = x and y
print(result_and)
# Output: False
# Logical OR
result_or = x or y
print(result_or)
# Output: True
# Logical NOT
result_not_x = not x
print(result_not_x)
# Output: False
result_not_y = not y
print(result_not_y)
# Output: True
-
Bitwise Operators:
Bitwise operators are used for manipulating individual bits in binary numbers. They are not as commonly used as other operators but can be useful in low-level operations. Some common bitwise operators include:
& (Bitwise AND): Performs a bitwise AND operation between two numbers.
Example
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a & b # Result: 0001 (1 in decimal)
| (Bitwise OR): Performs a bitwise OR operation between two numbers.
Example
a = 5 # Binary: 0101
b = 3 # Binary: 0011
result = a | b # Result: 0111 (7 in decimal)
^ (Bitwise XOR): Performs a bitwise XOR operation between two numbers.
An example showing how Bitwise Operators in Python work:
# Define two integer variables
a = 5 # Binary: 0101
b = 3 # Binary: 0011
# Bitwise AND
result_and = a & b
print(result_and)
# Output: 1 (Binary: 0001)
# Bitwise OR
result_or = a | b
print(result_or)
# Output: 7 (Binary: 0111)
# Bitwise XOR
result_xor = a ^ b
print(result_xor)
# Output: 6 (Binary: 0110)
# Bitwise NOT
result_not_a = ~a
print(result_not_a)
# Output: -6 (Binary: 11111111111111111111111111111010)
# Bitwise Left Shift
result_left_shift = a << 2
print(result_left_shift)
# Output: 20 (Binary: 10100)
# Bitwise Right Shift
result_right_shift = a >> 1
print(result_right_shift)
# Output: 2 (Binary: 0010)
-
Assignment Operators:
Assignment operators in Python are used to assign values to variables. They can also perform operations while assigning values. Common assignment operators include:
= (Assignment): Assigns the value on the right to the variable on the left.
Example
a = 5
+= (Add and Assign): Adds the value on the right to the variable on the left.
Example
a = 5
a += 3 # a is now 8
-= (Subtract and Assign): Subtracts the value on the right from the variable on the left.
An example of how assignment operators work in Python:
# Initialize a variable
x = 10
# Using assignment operators
x += 5 # Equivalent to x = x + 5
print(x)
# Output: 15
x -= 3 # Equivalent to x = x - 3
print(x)
# Output: 12
x *= 2 # Equivalent to x = x * 2
print(x)
# Output: 24
x /= 4 # Equivalent to x = x / 4
print(x)
# Output: 6.0
x %= 5 # Equivalent to x = x % 5
print(x)
# Output: 1.0
x **= 3 # Equivalent to x = x ** 3
print(x)
# Output: 1.0
x //= 2 # Equivalent to x = x // 2
print(x)
# Output: 0.0
-
Identity Operators and Membership Operators:
Identity Operators:
is: Checks if two variables refer to the same object in memory.
Example
a = [1, 2, 3]
b = a
result = (a is b) # True
is not: Checks if two variables do not refer to the same object in memory.
Example
a = [1, 2, 3]
b = [1, 2, 3]
result = (a is not b) # True
-
Membership Operators:
in: Checks if a value is present in a sequence (e.g., a list, tuple, or string).
In Python, these operators play a vital role in making decisions, performing logical operations, manipulating bits, and efficiently assigning values to variables. Understanding their use and precedence is essential for effective programming.
- Precedence and Associativity of Operators in Python
In Python, Operator precedence and associativity determine the priorities of the operator.
- Operator Precedence in Python
Operator precedence in Python determines the order in which operators are evaluated in expressions. For example, in 3 + 4 * 2, multiplication has higher precedence, so it's evaluated first (4 * 2 = 8), and then addition is done (3 + 8 = 11). Parentheses can be used to override precedence.
Here's an example that demonstrates operator precedence in Python:
Example
result = 3 + 4 * 2
print(result)
In this example, * has a higher precedence than +. Therefore, the multiplication operation is performed before addition.
4 * 2 is calculated first, resulting in 8.
Then, 3 + 8 is evaluated, resulting in the final value of 11.
Output: 11
This showcases how operator precedence dictates the order in which operators are evaluated within an expression.
- Operator associativity in Python
In Python, operator associativity determines the order in which operators with the same precedence are evaluated. Most operators, including addition and multiplication, are left-associative, meaning they're evaluated from left to right. For example, in 5 - 3 - 1, it's evaluated as (5 - 3) - 1, not 5 - (3 - 1).
Let's demonstrate operator associativity in Python with an example:
Example
result = 10 - 2 - 1
print(result)
In this example, the - operator is left-associative, so the expression is evaluated from left to right.
First, 10 - 2 is evaluated, resulting in 8.
Then, 8 - 1 is evaluated, resulting in the final value of 7.
Output: 7
This illustrates how the left-associative nature of the operator ensures that the operations are performed in a left-to-right order.