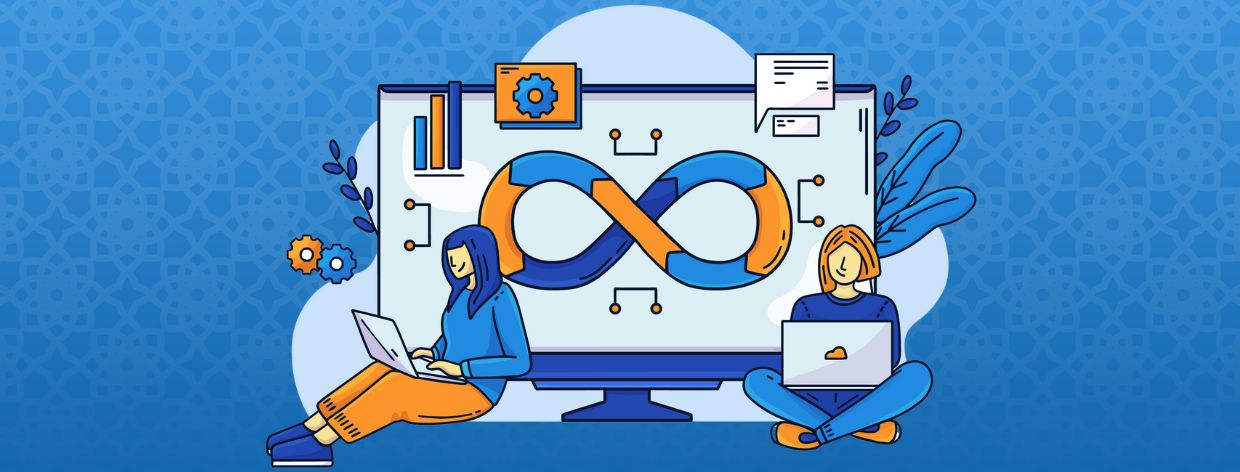
- 15th Feb 2024
- 00:27 am
- Ali Akavis
Dictionaries, fundamental data structures in Python, play a crucial role in managing key-value information. However, situations often arise where combining dictionaries becomes essential, whether it's merging user profiles, consolidating sensor readings, or integrating configuration files. This guide provides a comprehensive overview of various methods to effectively merge dictionaries in your Python projects, empowering you to navigate diverse data integration scenarios with confidence.
Built-in Helpers:
- update(): Merges one dictionary into another, but beware: later values overwrite existing ones.
- Unpacking Operator (**): Combines multiple dictionaries, prioritizing values from later ones. Easy and quick!
- Merge Operator (|) (Python 3.9+): The new way to merge, simple to use, but limited control over combining values.
Fine-Tuning Your Merge:
- Loops and keys(): For granular control, use loops and keys() to strategically merge values based on your needs.
- dict Constructor: Build a new dictionary with combined key-value pairs, but remember: existing keys get overwritten.
- dict() with Union Operator (|): Creates a dictionary with all keys, but needs custom functions to merge values.
- reduce() (Deprecated): While once useful, avoid reduce() as it's less readable and has better alternatives.
Leveraging the Experts:
- pandas and numpy: When dealing with specialized data structures, these libraries offer tailored merging functions for optimal performance.
Merging Dictionaries in Python
We will explores various methods to effortlessly merge dictionaries in your Python projects, empowering you to choose the right approach for your specific needs.
- Using The update() Method:
The update() method seamlessly incorporates one dictionary into another. Simply call dict1.update(dict2) to merge dict2 into dict1. However, be aware that existing keys in dict1 will be overwritten by values from dict2.
Here's an example:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
dict1.update(dict2)
print(dict1) # Output: {'a': 3, 'b': 2, 'c': 4}
To preserve the original dictionary, create a copy before updating:
dict1_copy = dict1.copy()
dict1_copy.update(dict2)
print(dict1_copy) # Output: {'a': 3, 'b': 2, 'c': 4}
print(dict1) # Output: {'a': 1, 'b': 2}
- Using Unpacking Operator (**):
The unpacking operator (**) offers a compact and readable way to combine multiple dictionaries. The expanded key-value pairs are added from left to right, with later values taking precedence. Consider this example:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
dict3 = {'d': 5}
merged_dict = {**dict1, **dict2, **dict3}
print(merged_dict) # Output: {'a': 3, 'b': 2, 'c': 4, 'd': 5}
You can also use conditional unpacking to selectively merge specific keys:
merged_dict = {**dict1, **dict2(a=10)} # Merge dict2 only if 'a' is different
print(merged_dict) # Output: {'a': 10, 'b': 2, 'c': 4}
- Using Union and Intersection with dict.fromkeys() and set.union()
The dict.fromkeys() method allows you to create a new dictionary with keys from both dictionaries and optional default values. Use set.union() to specify keys for union (all keys) or intersection (common keys) before forming the dictionary. Here's how:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
# Union (all keys with None as default value)
union_dict = dict.fromkeys(set.union(dict1.keys(), dict2.keys()))
# Intersection (common keys with default value)
intersection_dict = dict.fromkeys(set.intersection(dict1.keys(), dict2.keys()), 0)
print(union_dict) # Output: {'a': None, 'b': None, 'c': None}
print(intersection_dict) # Output: {'a': 0}
# Combine values with custom function
def combine_values(val1, val2):
return val1 + val2
union_dict.update({key: combine_values(union_dict[key], other_dict.get(key)) for key, other_dict in ((key, dict1), (key, dict2))})
print(union_dict) # Output: {'a': 4, 'b': 2, 'c': 4}
- Using The Merge Operator (|) (Python 3.9+): Simplicity with Limitations
The new | operator offers a streamlined way to merge dictionaries. Simply use dict1 | dict2 to create a new dictionary with combined keys. However, keep in mind that existing keys are overwritten, and there's no direct control over value merging:
dict1 = {'a': 1, 'b': 2}
dict2 = {'c': 3, 'd': 4}
merged_dict = dict1 | dict2
print(merged_dict) # Output: {'a': 1, 'b': 2, 'c': 3, 'd': 4}
- Using Granular Control with Loops and keys()
For fine-grained control over merging and value manipulation, utilize loops and the keys() method. This approach allows you to iterate through keys, compare values, and merge them strategically based on your requirements. Here's an example:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
merged_dict = {}
for key in dict1.keys() | dict2.keys():
value1 = dict1.get(key)
value2 = dict2.get(key)
if value1 is not None and value2 is not None:
merged_dict[key] = value1 + value2 # Combine values here
elif value1 is None:
merged_dict[key] = value2
else:
merged_dict[key] = value1
print(merged_dict) # Output: {'a': 4, 'b': 2, 'c': 4}
- Using The dict Constructor:
The dict constructor can create a new dictionary with combined key-value pairs. However, be cautious as existing keys will be overwritten by values from the latter dictionary in the list:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
merged_dict = dict(dict1, **dict2) # Unpacking operator within
print(merged_dict) # Output: {'a': 3, 'b': 2, 'c': 4}
- Using dict() with Union Operator (|)
While similar in syntax to the merge operator, using dict() with the union operator (|) creates a new dictionary with all keys from both sources. Value merging requires custom functions:
dict1 = {'a': 1, 'b': 2}
dict2 = {'a': 3, 'c': 4}
merged_dict = dict(set.union(dict1.keys(), dict2.keys()))
def combine_values(val1, val2):
return val1 + val2
for key in merged_dict:
merged_dict[key] = combine_values(merged_dict[key], dict1.get(key), dict2.get(key))
print(merged_dict) # Output: {'a': 4, 'b': 2, 'c': 4}
- Using reduce() (Historical Method):
While reduce() can be used for merging, it has been deprecated in Python 3 for readability and complexity reasons. It's recommended to utilize more modern alternatives like loops or unpacking operators.
Advanced Techniques & Considerations
While the core methods provide essential tools for merging dictionaries, advanced scenarios often demand deeper considerations. This section delves into advanced techniques and potential pitfalls to ensure you master the art of dictionary merging in Python.
- Deep vs. Shallow Copies:
Understanding the difference between deep and shallow copies is crucial for maintaining data integrity during merges. A shallow copy creates a new dictionary referencing the original objects, while a deep copy creates independent copies of both keys and values. This distinction matters when modifying nested data structures within dictionaries.
Consider using the copy module for controlled copying during merges. The deepcopy() function creates deep copies, ensuring changes within the merged dictionary don't affect the originals. Remember, libraries like pandas offer specialized deep copy functionalities for their data structures.
- Customizing Value Merging:
The ability to define how values are merged during the process unlocks powerful customization. You can leverage custom functions with update() or list comprehensions to strategically combine values:
def custom_merge(val1, val2):
if isinstance(val1, list):
return val1 + val2
else:
return max(val1, val2)
merged_dict = {**dict1, **dict2}
merged_dict.update({key: custom_merge(merged_dict[key], other_dict.get(key)) for key, other_dict in ((key, dict1), (key, dict2))})
This example highlights scenarios where specific merging logic is needed, like summing values or selecting the maximum.
- Error Handling:
Merging dictionaries can encounter potential errors like non-hashable elements (e.g., sets) or conflicting keys. Implement graceful error handling to prevent your code from crashing:
- Check data types before merging to avoid non-hashable element issues.
- Provide default values for potential missing keys using get() or conditional checks.
- Consider using try-except blocks to catch and handle specific exceptions, providing informative error messages for debugging.
By incorporating these advanced techniques and handling potential pitfalls, you can confidently handle complex dictionary merging scenarios, ensuring data integrity and achieving desired outcomes in your Python projects.
Applications of Python Merge Dictionaries
Merging dictionaries isn't just theoretical; it's a vital tool in various real-world Python applications. Here are some key examples:
1. Data Aggregation and Integration:
- Combining user profiles from different sources such as e-commerce, and social media in order to understand the user overall comprehensive analysis.
- Aggregating sensor data from several devices in order to get an overall understanding of the performance of the system.
- Merging configuration files from different environments for deployment purposes.
2. Machine Learning and Data Science:
- Combining features from various sources into a single dataset for model training.
- Merging different representations of the same data (e.g., text and image features) for enhanced prediction.
- Creating cross-validation folds by merging subsets of data.
3. Web Development and APIs:
- Combining data from multiple API responses into a single structured object for further processing.
- Merging user input with application configurations for personalized experiences.
- Building caching mechanisms by merging recent data with stored values.
4. Network Programming and System Administration:
- Merging information from different network devices for monitoring and troubleshooting.
- Combining configuration files from various servers for consistent management.
- Processing log data from multiple sources for security analysis.
5. Scientific Computing and Simulations:
- Merging results from multiple simulation runs for parameter tuning and analysis.
- Combining data from different physical models for comprehensive understanding.
- Building complex data structures by merging smaller components.