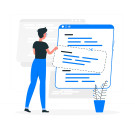
- 3rd Nov 2023
- 22:24 pm
- Admin
In Python, a list is a fundamental data structure, offering the versatility to store an ordered collection of items. These items can encompass various data types, such as integers, strings, or even other lists. Enclosed within square brackets [] and separated by commas, lists provide simplicity and adaptability, making them a fundamental component in Python programming.
Lists are widely used in Python programs for various purposes. You can create lists to store information, from basic data storage to complex data manipulation and transformation. Lists serve as the foundation for many other Python data structures, such as stacks and queues.
Create a List:
In Python, the process of creating a list involves enclosing the desired elements behind square brackets. For example:
my_list = [1, 2, 3, "apple", "banana"]
This essential operation frequently serves as the foundation for numerous Python programs. Lists, with their ability to encompass a variety of data types, offer remarkable flexibility in data representation. Lists can seamlessly combine numbers and strings, or they can even include lists within lists, constructing a hierarchical structure of data.
Access List Elements:
Accessing elements within a list is a common operation in Python. It involves using indexing to retrieve specific items. In Python, indexing begins with 0 for the first element, 1 for the second, so on etc. For instance:
first_element = my_list[0] # Retrieves the element with index 0
Accessing specific elements within a list is essential for a wide range of tasks, spanning from basic data retrieval to intricate data processing and data analysis. Indexing stands as a foundational capability that enhances the power and versatility of lists.
Negative Indexing in Python:
Negative indexing enables the retrieval of elements from the terminal position of a list. For example:
last_element = my_list[-1] # Retrieves the last element
second_last = my_list[-2] # Retrieves the second-to-last element
Negative indexing simplifies working with the last elements in a list, which can be particularly useful when you want to access the most recent data or the last item in a dataset.
Slicing of a List:
Python provides the capability to slice a list, allowing you to obtain a portion or sublist of it. Slicing involves specifying a range of indices using the start:stop:step notation. For instance:
my_slice = my_list[1:4] # Retrieves elements from index 1 to 3
Slicing is a versatile and powerful technique for efficiently working with lists. It's used for various tasks such as data filtering, data partitioning, or creating new lists from existing ones.
Add Elements to a List:
- Using append():
Employ the append() method to add elements to a list's end, like this:
my_list.append("cherry")
This method proves valuable for dynamically growing lists, especially when dealing with real-time or evolving data.
- Using extend():
The extend() method adds elements from an iterable, such as another list, to the list's end:
my_list.extend([4, 5, 6])
The extend() is indispensable when combining two lists or when adding multiple items to an existing list at once.
- Using insert():
The insert() method accurate insertion of elements at a designated point inside the list., as demonstrated here:
my_list.insert(2, "grape") # Inserts "grape" at index 2
The insert() method proves to be particularly advantageous when there is a need to insert an element at a precise position inside the list.
Change List Items:
Lists in Python are mutable, meaning their contents can be modified. You can change the value of an element in a list by assigning a new value to that element's index. For instance:
my_list[0] = "orange" # Changes the first element to "orange"
Modifying list items is fundamental when working with dynamic data, real-time data streams, or when you need to update information within your lists, especially in cases where data may change over time.
Remove an Item From a List:
- Using del Statement:
The del statement removes an element from a list based on its index. For example:
del my_list[1] # Removes the element at index 1
The del statement is a straightforward way to get rid of elements you no longer need in your list, which is essential for memory management and data cleanliness.
- Using remove():
The remove() method is designed to remove the initial instance of a specified element. For example:
my_list.remove("banana") # Removes the first "banana" found in the list
The remove() method is particularly useful when you want to eliminate specific items by their values rather than their positions in the list.
List Methods:
Python's built-in methods tailored for lists simplify common list operations, enhance data management, and boost code efficiency. These functions, designed to work seamlessly with lists, are powerful tools for any Python programmer.
Here are some essential list methods:
- sort(): The sort() method is used to arrange the elements of a list in ascending order. This method works for lists containing elements of the same data type.
- reverse(): The reverse() method reverses the order of elements within a given list.
- count(): The count() method is utilized to determine the frequency of occurrences of a particular element within a given list.
- index(): The index() method retrieves the index of the initial instance of a specified element.
Iterating through a List:
Iterating is a core concept in programming, especially when working with data stored in lists. Python offers a convenient method for iterating through lists using for loops. For example:
for item in my_list:
print(item)
This process allows you to sequentially access and manipulate each element. Whether you're transforming data, aggregating values, or filtering items, iteration plays a crucial role in data processing.
Check if an Element Exists in a List:
You can check whether a specific element exists within a list using the in keyword. For instance:
if "apple" in my_list:
print("Yes, 'apple' is in the list.")
Checking for the existence of elements in a list is crucial, especially when you need to determine whether specific data is present within your dataset. This operation is frequently used for data validation or searching tasks.
List Length:
The len() function can be used to ascertain the length of a list, which is the number of elements it contains. For example:
list_length = len(my_list)
Understanding the length of a list has significant importance in a multitude of list-processing tasks. It helps you understand the size of your dataset and can be used to allocate resources, plan memory usage, or validate data consistency.
List Comprehension:
List comprehensions offer an elegant and succinct approach to crafting lists from pre-existing data. They empower you to fashion a fresh list by applying a specified expression to each element within an existing list or another iterable. Here's an illustration:
squared_numbers = [x**2 for x in my_list]
List comprehensions present a concise syntax for producing new lists. They enable you to outline the process of extracting elements from existing ones. These handy tools frequently find application when you aim to reshape or sift through data in a manner that's both clear and efficient, elevating the conciseness and readability of your code.