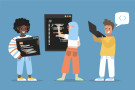
- 11th Dec 2023
- 21:40 pm
'isinstance()' is a built-in Python function that determines whether an object is an instance of a given class or a tuple of classes.
The syntax of the `isinstance()` function is as follows:
```
isinstance(object, classinfo)
```
- object: The checked object.
- classinfo: A single class or a set of classes. If the object is an instance of any of the classes in the tuple, the function will return 'True'.
Before performing specific operations on an object, the 'isinstance()' function is widely used for type checking and guaranteeing that it is of the desired type.
Uses of Python isinstance() Function
The 'isinstance()' function in Python is frequently used for type checking, and its primary purpose is to determine whether an object is an instance of a specified class or a tuple of classes. Here are some common applications for the 'isinstance()' function:
- Type Checking and Validation:
It is commonly used to validate the type of an object before performing certain operations. This helps to ensure that the object is of the expected type, avoiding potential runtime difficulties.
```
# Example: Check if a variable is an integer before performing arithmetic operations
x = 5
if isinstance(x, int):
result = x * 2
print(result)
```
- Polymorphism and Duck Typing:
Polymorphism and duck typing are frequent in Python since the emphasis is on the behaviour of objects rather than their explicit types. However, 'isinstance()' might still be beneficial when dealing with specific scenarios based on an object's type.
```
# Example: Implement a function that behaves differently based on the type of the input
def process_data(data):
if isinstance(data, int):
print("Received an integer:", data)
elif isinstance(data, str):
print("Received a string:", data)
else:
print("Unknown data type")
process_data(42) # Output: Received an integer: 42
process_data("hello") # Output: Received a string: hello
```
- Object Initialization and Factories:
You may want to generate objects based on their type in some cases. The function 'isinstance()' can be used to determine the type of an object and then initialise or construct objects accordingly.
```
# Example: Create an object based on its type
def create_instance(obj):
if isinstance(obj, int):
return MyClass1()
elif isinstance(obj, str):
return MyClass2()
else:
raise ValueError("Unknown object type")
my_object = create_instance(42)
```
- Handling Inheritance and Subtypes:
When working with class hierarchies and inheritance, 'isinstance()' can be used to determine whether an object is an instance of a given class or any of its subclasses.
```
# Example: Check if an object is an instance of a base class or its subclass
class Animal:
pass
class Dog(Animal):
pass
my_dog = Dog()
if isinstance(my_dog, Animal):
print("It's an animal or a subclass of Animal")
```
- Library and API Validation:
Libraries and APIs may require specific sorts of input in various circumstances. The use of 'isinstance()' ensures that the provided input conforms to the required types.
```
# Example: Validate input types in a function
def calculate_square(input_value):
if not isinstance(input_value, (int, float)):
raise ValueError("Input must be an integer or float")
return input_value ** 2
```
- Type-Based Dynamic Behaviour:
'isinstance()' can be used to dynamically modify the behaviour of a function or method based on the type of its input.
```
# Example: Adjust behavior based on the type of the input
def process_input(input_data):
if isinstance(input_data, str):
print("Processing a string:", input_data.upper())
elif isinstance(input_data, int):
print("Processing an integer:", input_data * 2)
process_input("hello") # Output: Processing a string: HELLO
process_input(5) # Output: Processing an integer: 10
```
Overall, `isinstance()` is a versatile tool for type checking in Python, and it's particularly useful in scenarios where you need to ensure the compatibility of objects with expected types or classes.
Advantage of Python isinstance() Function
Python's 'isinstance()' method has various advantages:
- Type Checking: It gives a simple and easy way to check the type of an object, guaranteeing that it is of the anticipated type before performing operations on it.
- Variability: 'isinstance()' can be used with a single class or a tuple of classes, enabling more flexible type checking scenarios.
- Polymorphism Support: It adheres to polymorphism principles, allowing code to be written in a way that concentrates on the behaviour of objects rather than their specific types.
- Duck Typing Compatibility: While Python supports duck typing, in which the focus is on the behaviour of an object rather than its type, 'isinstance()' can still be used when explicit type verification is required.
- Clear and Readable Code: By explicitly declaring the intended type, it improves code clarity, making it more readable and self-explanatory.
- Avoiding Runtime problems: Developers can avoid potential runtime problems when working with unusual data types by using 'isinstance()' to validate types before conducting operations.
- Handling Inheritance: It can be used to determine whether an object is an instance of a certain class or one of its subclasses, allowing for more advanced type checking in class hierarchies.
- Library and API Compatibility: When interacting with libraries or APIs, 'isinstance()' ensures that the provided inputs conform to the anticipated types, improving compatibility and preventing misuse.
- Dynamic Behaviour: 'isinstance()' enables for dynamic code behaviour adjustment based on the type of input, allowing for more flexible and adaptable programmes.
- Error Handling: It can be used in error handling to catch and handle different types of errors depending on the type of exception encountered.
- Improved Code Maintainability: 'isinstance()' type checking improves code maintainability by making it easier to comprehend the expected types of variables and parameters.
- Helping with Code Documentation: Using 'isinstance()' in code informs other developers or code readers about the expected kinds of variables and parameters, which contributes to better documentation.
'isinstance()' is a useful tool for enforcing type constraints in Python, adding to code robustness, clarity, and maintainability. It is critical in enabling polymorphism, handling inheritance, and maintaining compatibility in a variety of programming environments.