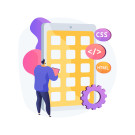
- 1st Nov 2023
- 13:43 pm
What is Python Generator
Generators in Python are like skilled magicians, capable of producing data on the fly without the need to store it all in memory at once. They offer an elegant solution to manage large datasets and infinite sequences while conserving resources. Let's dive into the world of Python generators and discover their unique abilities.
At the core of generators lies the `yield` keyword, which transforms an ordinary function into a generator function. When a generator function encounters `yield`, it temporarily suspends its execution, remembers its state, and delivers the current value. Unlike traditional data structures, generators generate data dynamically, one piece at a time, which is particularly advantageous when dealing with extensive data.
Creating a generator is straightforward. Here's a simple example:
```
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
```
Using this generator, you can efficiently retrieve data one item at a time, resulting in minimal memory usage:
```
for num in gen:
print(num) # Output: 1, 2, 3
```
Generators excel in scenarios such as reading large files, processing streams of data, or generating sequences that continue indefinitely. Their ability to generate data dynamically, combined with their memory efficiency, makes them invaluable tools for programmers, like magic tricks for managing data with finesse.
Generator Object
In Python, a generator object is an instance of a generator, a unique kind of iterable that creates values dynamically to save memory and enhance speed. Calling generator functions or using generator expressions produces generator objects. They eliminate the need to keep the entire sequence of data in memory at once and let you iterate over potentially massive amounts of data.
Here's a brief explanation of generator objects:
- Creation: Generator expressions encased in parenthesis or generator functions that contain the `yield} keyword are used to construct generator objects.
- Lazy Evaluation: Objects that generate are indolent. They create values as you want them to, rather than producing all of the values at once. This is very helpful for creating numerical sequences, analyzing big datasets, and streaming data.
- Iteration: You may use the `next()` method or a `for` loop to iterate across a generator object. With each `next()` call or iteration, the generator moves on to the subsequent `yield` statement.
- State Maintenance: Generator objects are able to resume generating values from their last pause point since they retain a memory of their internal state. The `yield` keyword allows for this by momentarily stopping the function's execution and preserving its state.
This is an illustration of how to make and use a generator object:
```
def simple_generator():
yield 1
yield 2
yield 3
gen = simple_generator()
for num in gen:
print(num) # Output: 1, 2, 3
```
The generator object 'gen' in this illustration was produced using the'simple_generator' function. The generator saves memory by producing values one at a time as you cycle over it, which makes it effective for handling big datasets or sequences.
Python Generator Expression
Creating generator objects is simple and memory-efficient when you use a generator expression in Python. While the square brackets [] are replaced with parentheses {}, the concept is identical to a list comprehension. Generator expressions work well for huge datasets and endless sequences because they allow you to produce a succession of values without having to store them all in memory at once.
Here's the syntax for a generator expression:
```
(generator_expression)
```
And here's how you can use a generator expression to create a generator:
```
gen_expr = (expression for variable in iterable)
```
Let's break it down:
- `expression` is the value or computation you want to generate for each item in the iterable.
- `variable` is a variable that takes on each item from the iterable.
- `iterable` is any iterable, such as a list, range, or another generator.
Here's an example of a generator expression that generates the squares of numbers from 1 to 5:
```
gen_expr = (x 2 for x in range(1, 6))
```
You can then iterate through the `gen_expr` generator, just like you would with any other generator, to obtain the computed values one at a time:
```
for num in gen_expr:
print(num) # Output: 1, 4, 9, 16, 25
```
When you need to develop generators with as little code and memory overhead as possible, generator expressions come in useful. When working with huge datasets or generating value sequences without precompiling and memory-storing them, they are very helpful. A Python generator expression is a concise way to create generator objects using a syntax similar to list comprehensions. Generator expressions use parentheses `()` instead of square brackets `[]`. Here's the basic syntax:
```
(generator_expression)
```
Within the parentheses, you specify the expression that generates values for the generator, typically using a loop or an iterable. Here's the general structure of a generator expression:
```
(expression for variable in iterable [if condition])
```
- `expression`: The value or computation that generates each item in the iterable.
- `variable`: A variable that takes on each item from the iterable.
- `iterable`: An iterable, such as a list, range, or another generator, over which you want to iterate.
- `condition` (optional): An optional condition that filters items from the iterable. Only items that satisfy the condition are included in the generator.
Here's an example that creates a generator expression to generate squares of numbers from 1 to 5:
```
gen_expr = (x 2 for x in range(1, 6))
```
In this example, `x 2` is the expression that generates values (squares of `x`), `x` is the variable taking on values from the iterable `range(1, 6)`, and there is no condition, so all numbers from 1 to 5 are included in the generator.
You can then iterate through the `gen_expr` generator using a `for` loop to obtain the computed values one at a time:
```
for num in gen_expr:
print(num) # Output: 1, 4, 9, 16, 25
```
When working with huge datasets or generating sequences without storing them in memory, generator expressions are a more memory-efficient method of creating generator objects than standard loops.
Applications of Python Generator
Python generators' memory-efficient and lazy evaluation features make them useful in a variety of programming contexts. The following are some typical uses for Python generators:
- Massive Data Handling: When processing massive datasets that are too big to fit fully in memory, generators are perfect. Without putting the complete dataset into memory, you may read data one piece at a time from a file, database, or network stream and process it as you go.
- Infinite sequences: A never-ending stream of sensor data or an infinite list of prime numbers are two examples of infinite sequences that generators are excellent at creating. Their ability to generate values on demand allows them to operate forever without running out of memory.
- Crawling and Scraping the Web: Crawling or scraping websites may expose you to enormous volumes of data. In order to ensure that you don't use up all of the system resources, generators can assist you in parsing and processing the data in an effective manner.
- In-Mean Time Processing: In circumstances involving parallel processing, generators can be employed. Data may be produced by creating a generator, and data can be consumed concurrently by several threads or processes without causing conflicts.
- Transformation and Filtering of Data: Generators are effective in filtering and transforming data. You may design a generator to iteratively alter data or filter out particular items from a series.
- Virtualization: Generators can be used to process data as it flows, enabling the usage of data streams of unlimited size in data stream processing applications like log analysis and real-time analytics.
- High-Memory Algorithms: Generic algorithms and other memory-intensive algorithms, such as simulations, can be implemented with the aid of generators. They lower memory needs by enabling you to produce and analyze data progressively.
- Intricate Data Frameworks: For the creation and exploration of intricate data structures, such as trees and graphs, generators are helpful. Step-by-step generation and exploration of nodes or components is possible.
- Mocking and Testing: Generators are useful for mimicking and testing. To increase the effectiveness of unit tests, you may use them to create test data, impersonate data sources, or mimic certain situations.
- Assortments and Permutations: For the purpose of creating permutations, combinations, and other combinatorial issues, generators are useful tools. Without creating every potential combination at once, you can effectively investigate every option.
Python generators are flexible tools that let you work with big datasets, write beautiful and fast code, and manage challenging data processing jobs. They are an important tool in the toolbox of every Python coder because of their memory efficiency and on-the-fly data production capabilities.