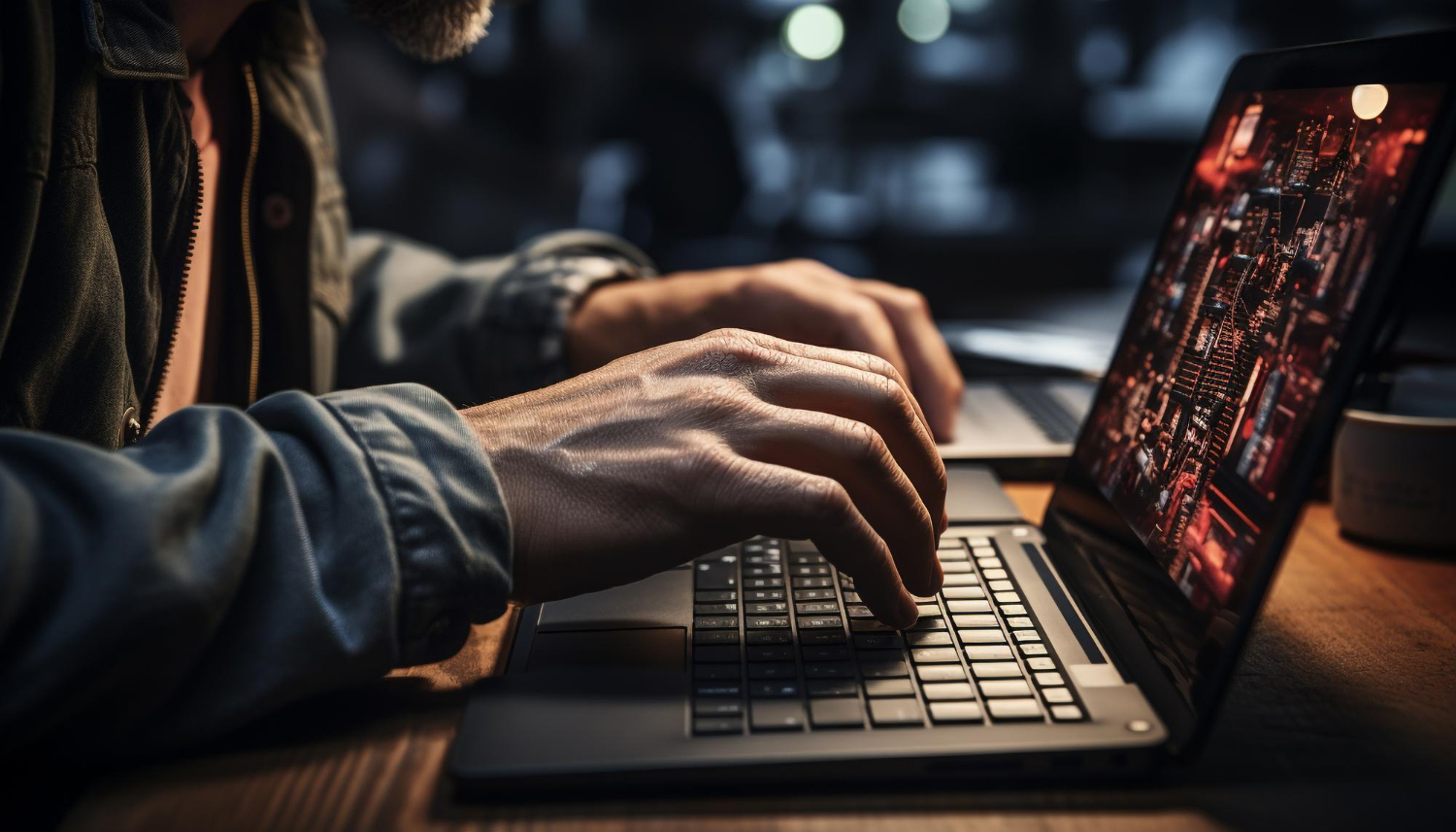
- 27th Dec 2023
- 14:24 pm
- Admin
In Python programming, filtering lists is a fundamental operation that empowers developers to selectively extract elements based on specific conditions. Whether working with numeric values, strings, objects, or dictionaries, Python offers diverse and efficient methods for filtering lists.
Python Filter List: Methods To Filter A List in Python
Filtering lists in Python is a fundamental skill that empowers developers to extract specific data efficiently. This comprehensive guide explores diverse methods and provides examples for filtering lists across various data types and conditions.
- Python Filter List of Objects:
When dealing with lists containing objects, developers often encounter the need to filter based on custom conditions. Consider a scenario with a list of Person objects:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
people = [Person("Alice", 25), Person("Bob", 30), Person("Charlie", 22)]
filtered_people = [person for person in people if person.age > 25]
In this example, the list of Person objects is filtered to include only individuals older than 25, showcasing the application of custom conditions for object-based filtering.
- Python Filter List By Condition:
This section explores methods for filtering lists based on specific conditions. For instance, consider filtering a list to include only positive numbers:
numbers = [1, -2, 3, -4, 5, -6]
positive_numbers = [num for num in numbers if num > 0]
Here, the list comprehension filters out negative numbers, demonstrating the flexibility of Python in conditional filtering.
- Python Filter List of Strings:
Filtering lists containing strings often involves conditions related to the string elements. An example includes filtering words longer than three characters:
words = ["apple", "banana", "kiwi", "grape"]
long_words = [word for word in words if len(word) > 3]
This demonstrates the application of string-based filtering to extract words meeting specific length criteria.
- Python Filter List of Strings Using Another List:
Filtering a list of strings using elements from another list is a common requirement. For instance, filtering names based on a reference list of allowed names:
all_names = ["Alice", "Bob", "Charlie", "David"]
allowed_names = ["Bob", "David", "Eve"]
filtered_names = [name for name in all_names if name in allowed_names]
Here, external references are used for precise filtering, showcasing the flexibility of Python in managing complex conditions.
- Python Filter() function
The built-in filter() function is a powerful tool for list filtering. This section delves deep into the filter() function, demonstrating its diverse applications in real-world scenarios.
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
The filter() function, combined with a lambda function, efficiently filters even numbers from the list.
- Python Filter List lambda() Function:
Introduction to the lambda() function for creating quick filtering functions. An example showcases filtering words starting with "a":
words = ["apple", "banana", "kiwi", "grape"]
a_words = list(filter(lambda x: x.startswith("a"), words))
The lambda function provides a concise way to create on-the-fly filtering conditions.
- Python Filter List Comprehension:
List comprehension is a powerful and concise way to filter lists. Consider extracting odd numbers using list comprehension:
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
odd_numbers = [num for num in numbers if num % 2 != 0]
List comprehension enhances both the efficiency and readability of the code.
- Python Filter List of Dictionaries
When dealing with lists containing dictionaries, filtering often involves conditions based on dictionary keys or values. Consider extracting people with age greater than 25:
people = [{"name": "Alice", "age": 25}, {"name": "Bob", "age": 30}, {"name": "Charlie", "age": 22}]
filtered_people = [person for person in people if person["age"] > 25]
This example demonstrates strategies for filtering lists with complex data structures.
Applications of Python List Filtering
List filtering is a versatile technique that finds valuable applications across diverse fields. Let's explore its impact in various domains:
- Data Science: Enhances data cleaning in projects by refining datasets through list filtering, ensuring accuracy.
- Web Development: Focuses on validating user input to secure web applications and maintain reliability.
- Information Retrieval: Crucial for efficient user interaction, enabling quick and relevant responses to queries.
- Financial Analysis: Supports informed investment decisions and risk management through analysis of extensive financial data.
- Business Analytics: Facilitates customized report generation, delivering targeted information for strategic planning.
- System Administration: Utilized in log analysis for troubleshooting, system monitoring, and maintaining IT system health.
- Manufacturing: Ensures quality control by filtering out defective items from production data.
- GUI Applications: Plays a central role in event-driven programming, enabling swift and seamless responses of Graphical User Interfaces (GUIs) to user interactions.
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.