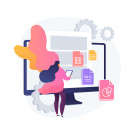
- 31st Oct 2023
- 23:08 pm
- Admin
The Programming Assignment Help delivers outstanding assistance for Python file handling. Our team comprises skilled developers proficient in managing files in Python. We offer expert guidance on opening, reading, writing, and closing files, helping you manipulate data effectively. We cover various file formats, such as text, CSV, JSON, and more. Our expertise extends to handling exceptions related to file operations and ensuring robust code. They aid in tasks like file I/O, file manipulation, and error handling, empowering you to work with files seamlessly. The Programming Assignment Help comprehensive support equips you with the skills to navigate and manage files in Python, facilitating efficient data processing and storage.
Python file handling allows reading from and writing to files on disk through Python code, enabling persistent storage and retrieval of program data in text or binary formats.
Advantages of File Handling
Key benefits of file handling in Python include:
- Ease of Use: Python's simple and intuitive syntax makes it accessible to both novice and experienced programmers. This user-friendliness reduces the learning curve and speeds up development.
- Rich Library Ecosystem: Python boasts a rich ecosystem of libraries and modules for file operations, such as pandas for data analysis, openpyxl for Excel files, and requests for web interactions, enhancing productivity and code efficiency.
- Cross-Platform Compatibility: Python's cross-platform compatibility ensures that code can run on various operating systems without modification, promoting portability and collaboration across different environments.
- Active Community Support: Python's large and active community provides extensive documentation, forums, and resources, facilitating rapid development and problem-solving in file-handling tasks.
Disadvantages of File Handling
Disadvantages of File Handling in Python:
- Error-Prone: Handling files in Python can be error-prone, with potential risks of data corruption or loss if not managed carefully. Developers must implement robust error-handling mechanisms to mitigate these risks.
- Performance: When dealing with large files, Python's file-handling operations can be relatively slow, consuming significant memory and resulting in reduced performance. This can be a drawback in applications that require high-speed data processing.
- Complexity: Working with complex file formats or data structures can be challenging, requiring developers to write intricate code for parsing and manipulation. Binary files, databases, and non-standard file formats can introduce complexity.
- Security Risks: Insufficient file permission settings and improper handling of sensitive information can lead to security vulnerabilities, potentially exposing the system to unauthorized access and data breaches.
- Platform Dependencies: Python's file handling may exhibit platform-specific behavior, making code less portable across different operating systems. Developers need to consider these differences when designing cross-platform applications.
Syntax
The open() function opens a file. It takes in the file path and mode as parameters. File modes include:
- 'r' for read,
- 'w' for write, and
- 'a' for append.
Method | Description |
close() | Flushes and closes the file object. |
detach() | Separates buffer from text stream and returns the buffer. |
fileno() | Returns the file's descriptor if available. |
flush() | Flushes the write buffer. Not available for read-only objects. |
isatty() | Check if a file stream is interactive. |
read() | Read number of characters at most. |
readable() | Check if an object is readable. |
readline() | Reads from the object until a newline or end of the file. |
readlines() | Returns a list of lines from the file object, where is the approximate character number. |
seek(, ) | Changes the pointer position to relative to the . |
seekable() | Check if the file object supports random access. |
tell() | Prints the current stream position. |
truncate() | Resizes the file stream to (or current position if unstated) and returns the size. |
write() | Writes to the file object and returns the written number of characters. |
writable() | Check whether the file object allows writing. |
writelines() | Writes a of lines to the stream without a line separator. |
Working of open() Function
The open() function returns a file object that includes methods,for example, read(), write(), close() etc. to work with the file.
file = open('data.txt') # open file for reading
file = open('data.txt', 'w') # open file for writing
file = open('img.png', 'rb') # open binary file for reading
- Close Files
file.close()
- Read and Write Files
text = file.read() # read full file contents
line = file.readline() # read one line
file.write('Hello world') # write string to file
-
File Object Attributes
The file object provides attributes like mode, name, closed etc. that contain metadata about the file.
-
File Object Methods
Methods like read(), write(), seek(), close() etc. on the file object can access and modify the file.
- Context Manager Protocol
The file object follows the context manager protocol and can be used with the 'with' statement for auto-resource handling.
- File Modes
Python supports file modes like 'r', 'w', 'a', 'r+', etc. with different read, write and append permissions.
- Binary vs Text Files
Python distincts between binary and text files. Binary files should be opened in binary mode which does not do encoding.
- With Statements
with open('data.txt') as file:
data = file.read()