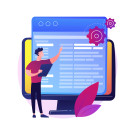
- 31st Oct 2023
- 22:52 pm
- Admin
The Programming Assignment Help offers exceptional assistance in Python Exception Handling. With a team of experienced Python developers, they provide top-notch support for handling exceptions. We understand the intricacies of error management and ensure your code runs smoothly. We offer guidance on try-except blocks, raising and catching custom exceptions, and best practices for error handling. Our experts help you identify and rectify various exceptions like Name Error, Value Error, Index Error, and more. They ensure your code is robust and resilient to errors, enhancing its reliability. TheProgrammingAssignmentHelp's dedicated service ensures you master Python's Exception Handling, making your code more efficient and dependable.
What is Python Exception Handling?
Exception handling is a set of instructions or commands that are used to handle exceptions/errors in your Python programs through the use of try, except, else, and finally blocks.
Exception handling in Python allows you to fix errors that occur during program execution. This makes programs bug-free and functional.
Python Exception Handling is a crucial aspect of programming that allows developers to manage and respond to runtime errors or exceptional situations gracefully. It involves using try, except, and optionally, finally blocks to handle potential exceptions without crashing the program. When an exception occurs, the program shifts to the corresponding exception block, enabling error-specific actions like logging, custom error messages, or graceful program termination. Exception handling enhances code robustness, making it less prone to unexpected errors, and facilitates debugging. Developers can catch and handle exceptions like ValueError, FileNotFoundError, and more, ensuring that their Python programs remain stable and resilient even in the face of unexpected issues.
Different types of exceptions in Python
Python has many built-in exception types like:
- ZeroDivisionError
- FileNotFoundError
- KeyError etc.
You can also define custom exception types by creating new Exception classes.
Some common built-in exceptions include:
- SyntaxError - Thrown when code has issues like missing colon or quotes.
- TypeError - Produced when using an object incorrectly, like adding a number and string.
- NameError - Created when using a variable name not defined.
- IndexError - Generated when accessing a list index that doesn't exist.
- KeyError - Triggered when accessing a dictionary key that is missing.
- ValueError - Caused when passing an invalid argument to a function.
- AttributeError - Occurs when accessing a non-existent attribute or method for an object.
- IOError - Happens when a problem opening or writing a file.
- ZeroDivisionError - Resulted from dividing a number by zero, which is not possible.
- ImportError - Caused when issue importing a module or library.
Difference between Syntax Error and Exceptions
Syntax errors occur when Python can't parse the code. Exceptions occur when syntactically correct code results in errors during execution.
- Try and Except Statement – Catching Exceptions
The try and except blocks allow you to catch exceptions that occur in the try block and handle them in the except block.
- Catching Specific Exception
You can specify which exception types to catch after the except statement. This allows for handling different exceptions differently.
- Try with Else Clause
The else block executes only when no exceptions are raised in the try block.
- Finally Keywords in Python
The final block always executes after the try and except blocks, even if an unhandled exception occurs. This allows for cleaning up resources.
- Raising Exception
You can forcibly raise exceptions in your code using the raise keyword.
- Developing Custom Exceptions
You can create custom exception classes by subclassing Exception. This allows for raising and catching application-specific exception types.
- Logging Exceptions
Logging exceptions to a monitoring service allows you to aggregate and analyze errors across your application.
Advantages of Exception Handling
Exception handling makes code cleaner by separating error handling from regular code. It also allows the program to continue executing after exceptions.
- AssertionError Exception
AssertionError is raised when an assert statement fails. This can be used to check for conditions that should be true.
- The try and except Block: Handling Exceptions
The try/except block catches and handles exceptions in Python.
- Cleaning Up After Using finally
The final block always executes after trying/except to do any cleanup.
FAQs
- Is it possible to handle multiple different exceptions within the same except block?
Yes, you can catch multiple exceptions in a single except block by specifying them as a tuple. For example: except (ExceptionType1, ExceptionType2) as e:.
- What is the difference between except and else blocks in exception handling?
The except block is used to catch and handle exceptions when they occur, while the else block is executed only when no exceptions are raised within the try block.