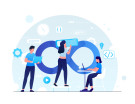
- 31st Oct 2023
- 22:27 pm
TheProgrammingAssignmentHelp excels in providing exceptional assistance with Python dictionaries. Our team of experienced Python developers offers comprehensive guidance on dictionaries, one of Python's fundamental data structures. We cover dictionary creation, manipulation, key-value pair operations, and in-depth knowledge on dictionary methods. Whether it's iterating through dictionary elements, sorting, or performing complex operations, their experts ensure you master this powerful data structure. They also offer insights into dictionary comprehension and efficient ways to work with nested dictionaries. Their guidance empowers you to harness the full potential of Python dictionaries for efficient data storage, retrieval, and manipulation, making your code more effective and organized.
Python Dictionary
A Python dictionary is a built-in data type that functions as a unique key-value store. Dictionaries are mutable unordered collections of keys that are mapped against values.
What is a Python Dictionary?
A Python dictionary is an unordered collection of key-value pairs used to store data values like a map, where keys act as unique identifiers mapping to their associated values.
Creating Dictionaries
Dictionaries in Python can be created by placing comma-separated key-value pairs between curly braces {} or using the dict() constructor, like dict(key1=val1, key2=val2), where keys and values can be of any immutable data type.
Accessing Elements
Individual elements can be accessed by key name using square bracket notation. Trying to access a non-existent key will result in a KeyError exception. The keys(), values() and items() dictionary methods can be used to return iterables over the contents.
Nested Dictionaries
Dictionaries can contain other dictionaries to create nested or hierarchical data structures. Inner dictionaries can be accessed using chained indexing on the keys.
Deleting Elements
- Use the del statement to remove a key-value pair by specifying the key. For example:
del dict['key']
- Use the pop() method to remove and return the value of the specified key. For example:
value = dict.pop('key')
- Use the popitem() method to remove and return an arbitrary key-value pair from the dictionary. For example:
key, value = dict.popitem()
- Use the clear() method to empty the entire dictionary and remove all key-value pairs. For example:
dict.clear()
Reassign the dictionary to an empty dictionary {} to create a new empty dictionary.
Dictionary Methods
Useful dictionary methods include get() to access keys, pop() and popitem() to remove elements, update() to add multiple items, and clear() to empty a dictionary. There are also methods like keys(), values(), items() for accessing dictionary contents.
Method | Description |
dict.clear() | Removes all elements from dictionary |
dict.copy() | Returns shallow copy of dictionary |
dict.get(key, default) | Get value for key, default if not found |
dict.items() | Returns list of (key, value) tuples |
dict.keys() | Returns list of dictionary keys |
dict.update(dict2) | Updates dict with dict2's key-values |
dict.values() | Returns list of dictionary values |
dict.pop(key) | Removes key and returns value |
dict.popitem() | Removes and returns last inserted key-value |
dict.setdefault(key, default) | Sets key to default if key not in dict |
dict.has_key(key) | Returns True if key in dict, else False |
dict.get(key, default) | Get value for key, default if key not found |
Properties of Dictionary Keys
Here are some key properties of dictionary keys in Python:
- Dictionaries store data values in key-value pairs, like a map or hash table.
- Dictionaries are enclosed in curly braces {} and have keys and values separated by a colon:
- Keys are unique identifiers and must be immutable objects like strings or numbers.
- Values can be any Python data type and can duplicate.
- Dictionaries are unordered - the items have no specific order.
- Items are accessed via keys, not numeric indexes like lists.
- Dictionaries are mutable and dynamic - keys and values can be added, updated or deleted.
- Useful dictionary methods include get(), pop(), update(), keys(), values() etc.
- Dictionaries allow efficient lookup and storage for data access and manipulation.