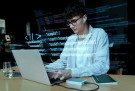
- 3rd Nov 2023
- 23:03 pm
Python, renowned for its versatility and power, provides a diverse set of data structures for efficient data manipulation. Among these, the deque, a shortened form of "double-ended queue," stands out. Deques serve as an essential tool for handling data collections that necessitate swift insertion and removal of elements from both the front and rear ends. This flexibility and performance make deques a valuable addition to Python's arsenal of data structures.
What is Python Deque?
Within Python, a versatile and robust programming language, an array of data structures exists to effectively manage and manipulate data. Among these, the deque, a shortened form of "double-ended queue," stands out. Deques offer a dynamic means of working with collections of elements, facilitating quick insertions and removals from both ends. This distinctive feature endows deques with exceptional capabilities for acting as a double-ended queue, making data manipulation remarkably straightforward.
Deques are mutable and thread-safe data structures that provide bidirectional access to elements, empowering you to perform queue and stack operations with remarkable efficiency. These structures enable swift insertions and deletions, with the flexibility of adding or removing items from both ends. Whether your task demands implementing a queue, a stack, or handling data with specific requirements, the Python deque proves invaluable. Its capacity to provide rapid access to both ends of a collection grants it unrivaled efficiency, rendering it an ideal choice for a broad spectrum of applications.
Features of a Python Deque
Deques boast several essential features:
- Double-ended Operations: Deques provide operations for adding and removing elements from both the front and rear ends, allowing for efficient queue and stack implementations.
- Efficiency: Deques are designed to perform these operations in constant time, making them an excellent choice for situations that require fast inserts and removals.
- Mutable: Deques are mutable, which means you can modify their content by adding or removing elements.
- Thread-safe: Deques are thread-safe, allowing for safe multithreaded use and data manipulation.
Applications of a Deque
Deques find applications in various scenarios, including:
- Queue and Stack Implementations: Deques are ideal for creating both queues and stacks efficiently, allowing for rapid insertion and deletion of elements.
- Memory Management: Situations requiring efficient memory management, especially when dealing with dynamically changing data, often benefit from the use of deques.
- Algorithm Optimization: In specific algorithms, deques are used to optimize performance, primarily due to their ability to provide fast access to both ends of the collection.
Creating and Initializing a Deque:
In Python, initializing a deque is essential for managing data effectively. You have two primary methods for creating a deque:
- Using the deque() Function
Utilize Python's built-in deque() function from the collections module:
from collections import deque
my_deque = deque()
This creates an empty deque named my_deque, ready for data.
- Initializing a Deque with Elements
Alternatively, you can initialize a deque with predefined elements using a list or iterable. This method is particularly useful when you have a specific dataset in mind and want to start working with it immediately.
my_deque = deque([1, 2, 3, 4, 5])
It enables you to populate your deque with essential data right from the beginning, tailored to your requirements.
Performing Common Deque Operations:
Deques in Python provide a versatile and efficient way to manage data. They support various operations for adding, removing, and accessing elements, making them a powerful data structure. Below are essential operations for working with deques:
- Adding Elements to a Deque:
Deques offer straightforward methods to insert elements. The append() method adds elements to the right end, while appendleft() adds to the left. These functions are handy for building deques dynamically.
deque.append(42)
deque.appendleft(11)
Using append() adds an element to the right end, making it the last element. On the other hand, appendleft() inserts the element at the left end, becoming the first.
- Inserting Multiple Data Elements:
Deques support efficient addition of multiple elements. To add elements to the right end, extend() is the method to use. If you want to add items at the left end, employ extendleft(). These operations are beneficial for combining data or inserting multiple items simultaneously.
deque.extend([1, 2, 3])
deque.extendleft([4, 5, 6])
Using extend(), you can concatenate a list of elements efficiently to the right end. Similarly, extendleft() appends elements to the left.
- Removing Elements:
Removing elements is straightforward. The pop() method eliminates and returns the rightmost element. Alternatively, popleft() removes the leftmost element and returns it, adhering to the First-In-First-Out (FIFO) order.
rightmost = deque.pop()
leftmost = deque.popleft()
With pop(), you eliminate the last-added element. Conversely, popleft() gets rid of the first inserted element.
- Clearing a Deque:
You may need to clear a deque entirely. The clear() method accomplishes this by removing all elements, leaving an empty deque.
deque.clear()
Clearing a deque ensures it contains no elements and can be useful when you need to reset it to its initial state.
- Accessing Elements:
To access elements within a deque, use indexing, which allows retrieval based on position.
element = deque[3]
Indexing begins at 0, so deque[3] gets the fourth element. It's useful when you need to work with specific data in the deque.
- Modifying Elements:
Deques enable efficient element modification using indexing and assignment. To update an element, directly assign a new value.
deque[2] = 99
Here, the element at index 2 is modified with the value 99. Modifying elements is crucial for updating data in the deque.
Working with Deques as a Queue:
Deques are ideal for implementing queues following a First-In-First-Out (FIFO) order. Use append() to enqueue elements at the right end and popleft() to dequeue from the left, ensuring the order of processing matches the order of addition.
deque.append(10)
deque.append(20)
item = deque.popleft()
Following FIFO, you can manage a queue efficiently, ensuring the first element added is the first to be processed.
- Checking for an Empty Deque Queue:
To determine if a deque queue is empty, use a conditional statement.
if not deque:
print("The deque is empty")
Verifying if a deque is empty is crucial for managing its state and avoiding attempts to dequeue elements when there are none.
Working with Deques as a Stack:
Deques are also suitable for stack operations, adhering to a Last-In-First-Out (LIFO) order. Push elements onto the stack with append() at the right end and pop elements using pop() to retrieve the rightmost element.
deque.append(100)
element = deque.pop()
Deques as stacks are valuable when elements must be processed in the reverse order of addition.
Verifying an Empty Deque:
Check if a deque is empty by using a conditional statement to confirm its state.
if not deque:
print("The deque is empty")
Checking for an empty deque is essential to manage its state and prevent operations on an empty deque.