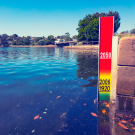
- 13th Feb 2020
- 05:43 am
- Adan Salman
Programming Assignment Question
a. Tell the user that the program uses data from the Jason-2 Satellite Altimeter to predict sea level from the years 2020 to 2050.
b. Store the sea level data in an array. You only need to use one data point for each year from 1993 to the present year. Follow the directions on the web page to zoom in to the years from 1980 - present and then drag your pointer over each year from 1993 to present, noting the sea level for that year. I gathered the data for the array. - 12.94, 13.61, 14.17, 13.95, 13.86, 14.80, 14.07, 15.07, 15.42, 15.67, 16.53, 16.18, 16.95, 17.12, 16.83, 17.56, 17.65, 18.17, 17.64, 18.67, 19.43, 19.42, 20.08, 21.39, 20.87, 21.36
c Find the average annual change in sea level over all the years specified in the data. (Hint - use a loop to store the annual change in an array over the years, and then use a loop to compute the average annual change).
d. Assume a linear increase and compute the predicted sea-level rise for the years 2020, 2025, 2030, 2035, 2040, 2045, and 2050. Store these results in their own array. (Hint - just use the average you computed in part c as the annual change for the future years).
e. Display the results for the user and be sure to reference the data set as specified in the data file so the user knows where the data came from.
Sample output: The predicted Global Mean Sea Level is 2020 64.32 2025 68.98 2030 73.51 2035 78.12 2040 83.43 2045 88.12 2050 93.04
Programming Assignment Solution
#include #include #include void main() { printf("This program uses data from the Jason-2 Satellite Altimeter to predict sea level from the years 2020 to 2050.\n"); float arr[] = { 12.94, 13.61, 14.17, 13.95, 13.86, 14.80, 14.07, 15.07, 15.42, 15.67, 16.53, 16.18, 16.95, 17.12, 16.83, 17.56, 17.65, 18.17, 17.64, 18.67, 19.43, 19.42, 20.08, 21.39, 20.87, 21.63 }; float y2020[5]; float y2025[5]; float y2030[5]; float y2035[5]; float y2040[5]; float avg2020 = 0.0; float avg2025 = 0.0; float avg2030 = 0.0; float avg2035 = 0.0; float avg2040 = 0.0; for (int i = 0; i <5; i++) { y2020[i] = arr[i]; y2025[i] = arr[i+5]; y2030[i] = arr[i+10]; y2035[i] = arr[i+15]; y2040[i] = arr[i+20]; } for (int i = 0; i < 5; i++) { avg2020 = avg2020 + y2020[i]; avg2025 = avg2025 + y2025[i]; avg2030 = avg2030 + y2030[i]; avg2035 = avg2035 + y2035[i]; avg2040 = avg2040 + y2040[i]; } float sum = 0.0; avg2020 = avg2020 / 5; avg2025 = avg2025 / 5; avg2040 = avg2040 / 5; avg2030 = avg2030 / 5; avg2035 = avg2035 / 5; printf("Avg Yearly Change : \t");printf(" %.2f", (avg2020 + avg2025 + avg2030 + avg2035 + avg2040) / 5); printf("\n"); avg2020 = avg2020*4.72; sum = sum + avg2020; printf("2020:\t"); printf("%.2f", sum ); printf("\n"); sum = sum + 4.72; printf("2025:\t"); printf("%.2f", sum ); printf("\n"); sum = sum + 4.72; printf("2030:\t"); printf("%.2f", sum ); printf("\n"); sum = sum + 4.72; printf("2035:\t"); printf("%.2f", sum); printf("\n"); sum = sum + 4.72; printf("2040:\t"); printf("%.2f", sum ); printf("\n"); sum = sum + 4.72; printf("2045:\t"); printf("%.2f", sum ); printf("\n"); sum = sum + 4.72; printf("2050:\t"); printf("%.2f", sum ); printf("\n"); }