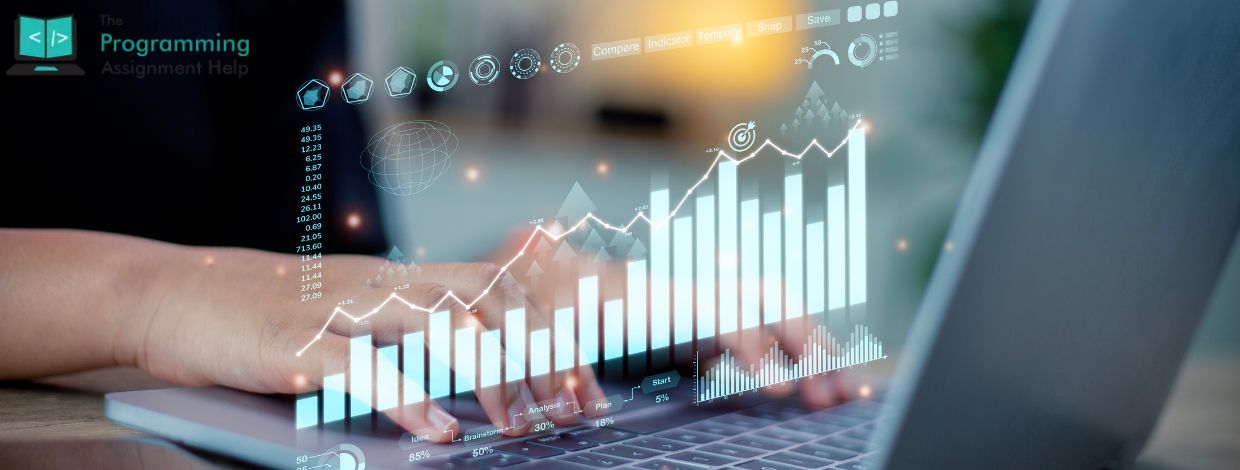
- 11th Jul 2024
- 09:35 am
- Admin
Need help with your LinkedList class? We can assist in adding methods to remove nodes, swap head and tail nodes, and randomly remove nodes. Our guidance also extends to joining letters, removing duplicates, counting vowels, merging sorted lists, and enhancing DoublyLinkedList functions. Let us help you optimize your code and improve your data structures.
Problem 1: In the LinkedList class, add a method remove(n) to remove the specified node n from the list and return its element. You may assume the linked list contains the specified node.
Then, add a swap_head_tail() method to swap the head and tail nodes (not just elements) in the linked list and a random_remove() method to randomly remove a node from the linked list and return its element.
Problem 2: Given a LinkedList of letters s, write the following functions:
- join(s) to join all the letters together in the linked list and return the joined string.
- remove_duplicate(s) to remove all the duplicate letters, join all the unique letters together, and return the joined string, for example, if the input linked list is ‘a’ → ‘c’ → ‘b’ → ‘a’ → ‘a’ → ‘c’ → ‘d’, your method should return “acbd” or “bacd”.
- count_vowels(s) to return the number of vowels in the linked list.
Problem 3: Given two sorted SinglyLinkedList of integers, write a function
- merge2lists(linked_list1, linked_list2) to merge the two linked lists into a new sorted linked list and return it
For example:
Before merging: list1: 2→11→19→21→23→24
list2: 3→9→15→16→22
After merging: 2→3→9→11→15→16→19→21→22→23→24
Problem 4: In the DoublyLinkedList class, add the following public methods:
- get_first() to return the first node (not the header) in the linked list
- get_last() to return the last node (not the trailer) in the linked list
- contains(e) method to return true if the linked list contains a specified element e, false otherwise
- add_before(e, n) to insert the specified element e before that specified node n
- add_after(e, n) to insert the specified element e before that specified node n
You may assume the linked list contains the specified node for add_before() and add_after()
Object & Structure & Algorithm - CISC311 - Get Assignment Solution
Please note that this is a sample assignment solved by our Python Programmers. These solutions are intended to be used for research and reference purposes only. If you can learn any concepts by going through the reports and code, then our Python Tutors would be very happy.
- Option 1 - To download the complete solution along with Code, Report and screenshots - Please visit our Programming Assignment Sample Solution page
- Option 2 - Reach out to our Python Tutors to get online tutoring related to this assignment and get your doubts cleared
- Option 3 - You can check the partial solution for this assignment in this blog below
Free Project Solution - Object & Structure & Algorithm - CISC311
from re import T
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def push(self, new_data):
new_node = Node(new_data)
new_node.next = self.head
self.head = new_node
def get_first(self):
return self.head
def get_last(self):
temp=self.head
while(temp.next!=None):
temp=temp.next
return temp
def contains(self, key):
temp=self.head
while(temp):
if(temp.data==key):
return True
return False
def add_before(self, e, n):
temp=self.head
while(temp.next.data!=e):
temp=temp.next
new_node = Node(n)
new_node.next=temp.next
temp.next=new_node
def add_after(self, e, n):
temp=self.head
while(temp.data!=e):
temp=temp.next
new_node = Node(n)
new_node.next=temp.next
temp.next=new_node
def deleteNode(self, key):
temp = self.head
if (temp is not None):
if (temp.data == key):
self.head = temp.next
temp = None
return
while(temp is not None):
if temp.data == key:
break
prev = temp
temp = temp.next
if(temp == None):
return
prev.next = temp.next
temp = None
def printList(self):
temp = self.head
while(temp):
print (" %d" %(temp.data)),
temp = temp.next
def join(self):
temp = self.head
ret=""
while(temp):
ret+=temp.data
temp = temp.next
return ret
def remove_duplicates(self):
temp = self.head
ret=""
elements=set([])
while(temp):
if(elements.__contains__(temp.data)==False):
ret+=temp.data
elements.add(temp.data)
temp = temp.next
return ret
def count_vowels(self):
temp = self.head
cnt=0
while(temp):
if(temp.data=='a' or temp=='e' or temp.data=='i' or temp.data=='o' or temp.data=='u')
cnt=cnt+1
temp = temp.next
return cnt
def merge2lists(headA, headB):
dummyNode = Node(0)
tail = dummyNode
while True:
if headA is None:
tail.next = headB
break
if headB is None:
tail.next = headA
break
if headA.data <= headB.data:
tail.next = headA
headA = headA.next
else:
tail.next = headB
headB = headB.next
tail = tail.next
return dummyNode.next
Get the best Object & Structure & Algorithm - CISC311 - Assignment help and tutoring services from our experts now!
About The Author - John Smith
John Smith is an experienced software developer specializing in data structures and algorithms. With a strong focus on LinkedLists and DoublyLinkedLists, John excels in creating efficient solutions for complex data management problems. His expertise includes methods for node removal, swapping, merging sorted lists, and optimizing linked list operations for better performance.