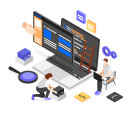
- 15th Nov 2023
- 23:03 pm
- Admin
In Java, methods are vital for organizing and structuring code, allowing the implementation of functional blocks to accomplish specific tasks. They encapsulate sets of instructions and routines, fostering code reusability and improving the maintainability of Java programs.
What is a Method in Java?
In Java, a method represents a defined block of code within a class designed to execute a specific task or operation. It encapsulates a sequence of statements, functions, or procedures, facilitating reusability and modularity in programming. Methods are instrumental in promoting a structured approach to coding, enabling the division of complex operations into smaller, more manageable units. They offer a way to organize code logically and efficiently, fostering ease in the development, maintenance, and readability of programs.
Methods in Java can handle data manipulation or execute actions without repetition, enhancing the clarity, efficiency, and scalability of Java programs. Their functionality spans from basic mathematical computations to complex operations, empowering developers to construct sophisticated and well-organized applications.
Types of Methods in Java
In Java, methods are categorized into two primary types: Predefined Methods and User-defined Methods.
- Predefined Methods: These are inherent functionalities provided within Java's libraries. They include a variety of operations for tasks such as mathematical calculations, string manipulations, file handling, and more. Predefined methods are readily available for use and serve as foundational building blocks for Java programs.
- User-defined Methods: Created by developers to fulfill specific needs within their programs, user-defined methods encapsulate custom functionalities. These methods offer the flexibility to define unique operations, promoting code reusability, organization, and the creation of tailored functionalities aligned with specific program requirements.
public class Example {
// User-defined method without parameters and return type
public void greet() {
System.out.println("Hello, World!");
}
// User-defined method with parameters and return type
public int add(int a, int b) {
return a + b;
}
public static void main(String[] args) {
Example obj = new Example();
obj.greet(); // Calling the greet method
int sum = obj.add(5, 3); // Calling the add method with parameters
System.out.println("Sum: " + sum);
}
}
Method Declaration
In Java, method declaration involves various components that collectively define the structure and functionality of a method.
- Modifier: This component specifies the accessibility level (public, private, protected, or default) of the method. It controls the method's visibility and accessibility within the program.
- Return Type: A method's return type specifies the type of value the method will deliver upon execution. Methods can offer various data types as returns, including integers, strings, or custom objects. When a method has a return type of 'void,' it signifies that the method doesn't return any value.
- Method Name: The method name serves as the identifier, uniquely representing the method and allowing it to be called within the code.
- Parameter List: Parameters are variables passed to the method, enabling it to receive inputs necessary for its execution. Parameters define the type and number of arguments a method expects.
- Exception List: It specifies the exceptions that the method might throw during execution. It allows the method to declare specific exceptions it can handle or propagate.
- Method Body: The method body contains the set of statements or code block that defines the actual functionality of the method. It executes the operations and tasks specified by the method.
2 Ways to Create Method in Java
Within Java, methods are classified into two primary types: instance methods and static methods. Each of these method types serves specific roles in Java, offering versatility and enabling various functionalities within a program.
- Instance Method: These methods are linked to specific object instances within a class. They operate on instance variables and have access to other instance methods and properties. Invocation of instance methods requires the creation of an object. These methods are crucial for altering the state of an object, performing operations based on its properties, and implementing object-specific functionalities.
- Static Method: Static methods belong to the class itself rather than any specific object. They operate independently of object creation and are invoked using the class name. These methods cannot access instance variables but are ideal for performing general computations, utility functions, or tasks that do not rely on object-specific data.
public class StaticMethodExample {
// Static method
public static void displayMessage() {
System.out.println("This is a static method.");
}
public static void main(String[] args) {
// Calling a static method directly using class name
StaticMethodExample.displayMessage();
}
}
Method Overloading
Method overloading in Java involves defining multiple methods within the same class, all sharing the same name but differing in the number or type of parameters they accept.
- Versatile Functionality: Method overloading permits multiple methods with the same name but different parameters, offering varied functionalities.
- Parameter Variation: Methods differ based on the number, order, or types of parameters they accept.
- Enhanced Readability: Provides a clearer, more organized code structure, making the program more comprehensible.
- Flexibility in Implementation: Developers can create methods to perform similar tasks with different inputs, catering to diverse requirements.
- Efficient Code: Reduces complexity by consolidating related functionalities under a single method name, promoting cleaner and more concise code.
public class OverloadingExample {
// Method overloading with different parameter types
public int multiply(int a, int b) {
return a * b;
}
public double multiply(double a, double b) {
return a * b;
}
public static void main(String[] args) {
OverloadingExample obj = new OverloadingExample();
int productInt = obj.multiply(5, 3);
double productDouble = obj.multiply(2.5, 4.0);
System.out.println("Product (int): " + productInt);
System.out.println("Product (double): " + productDouble);
}
}
Method Signature
In Java, a method's signature comprises its name and parameter list, serving as a distinctive identifier for the method.
- Unique Identifier: The method's identity is defined by its name and parameter list.
- Parameter Details: Includes the number, types, and order of parameters accepted by the method.
- Excludes Return Type: Does not encompass the return type or exceptions in its definition.
- Enables Overloading: Allows for the creation of multiple methods with the same name but different signatures.
- Determines Method Execution: The method signature differentiates methods and guides Java in selecting the appropriate method to execute based on provided arguments.
- Critical for Distinction: Crucial in Java to identify and distinguish between methods within a class.
How to Name a Method?
To name a method in Java, follow established naming conventions for improved clarity and consistency.
- Clarity in Naming: Choose names that clearly convey the method's purpose and functionality.
- Camel Case Convention: Begin the method name with a lowercase letter and capitalize the initial letters of subsequent words for better readability.
- Descriptive and Concise: Opt for descriptive yet succinct names, avoiding excessive length or vagueness.
- Reflective of Function: Ensure the method name reflects the operation it performs within the code.
- Consistency: Follow naming conventions consistently across the codebase for uniformity and easier comprehension.
- Enhanced Readability: Well-chosen, clear names contribute to code understanding, aiding maintenance and collaboration.
Method Calling
Method calling in Java involves invoking or executing a method within the program's code.
- Execution Trigger: Method calling initiates the execution of a specific method's set of instructions.
- Method Reference: Calls involve referencing the method's name within the code, along with necessary arguments if it's a parameterized method.
- Function Invocation: Initiates the method's functionality, allowing reuse of encapsulated code and promoting modular programming.
- Inter-method Calls: Methods can call other methods within the same class or different classes, promoting structured, reusable code.
- Flow Control: Facilitates the structured flow of code execution by invoking specific functionalities at designated points in the program.
- Repetitive Use: Methods' repetitive use through calls offers a streamlined, efficient, and modular approach to implementing program logic.
public class MethodCallExample {
// Method to calculate the area of a rectangle
public int calculateArea(int length, int width) {
return length * width;
}
public static void main(String[] args) {
MethodCallExample obj = new MethodCallExample();
int length = 5;
int width = 10;
int area = obj.calculateArea(length, width); // Method call to calculate area
System.out.println("Area of the rectangle: " + area);
}
}
Memory Allocation for Methods Calls
When methods are called in Java, memory allocation takes place to execute the instructions within the method.
- Memory Assignment: Method calls trigger memory allocation for variables, arguments, and execution contexts required during method execution.
- Local Variables Allocation: Reserved space for local variables, parameters, and the method's execution context.
- Call Stack Management: Memory allocation for the call stack, organizing method execution flow and handling method invocations.
- Resource Release: Upon method completion, allocated memory is released, ensuring efficient resource utilization.
- JVM Management: Java Virtual Machine (JVM) oversees memory allocation and deallocation for method calls, maintaining system efficiency.
- Optimized Resource Use: Proper management of memory allocation and deallocation promotes efficient use of system resources during method execution.
Advantages to using methods in Java
Utilizing methods in Java offers numerous advantages vital to efficient programming:
- Modularity: Methods promote code organization by breaking down complex tasks into smaller, manageable units, enhancing code modularity and readability.
- Reusability: Encapsulating logic within methods enables reuse, reducing redundancy, and allowing the same code to be invoked at multiple points in the program.
- Maintenance: Methods simplify code maintenance by isolating specific functionalities, enabling easier modification or debugging without affecting the entire codebase.
- Scalability: They facilitate scalability by allowing additional functionality to be easily added or modified without altering the entire program structure.
- Readability: Well-structured methods significantly enhance code comprehension, resulting in more understandable programs that are easier to maintain. This contributes to improved collaboration and overall software development.