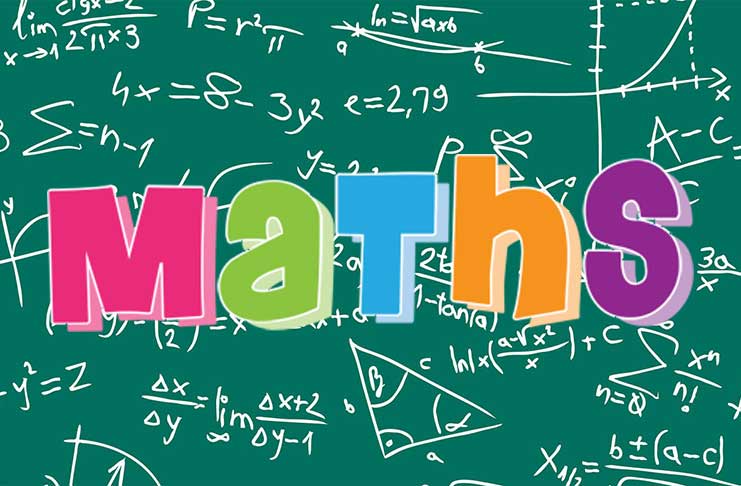
- 12th May 2019
- 00:01 am
Challenge - Write a program that reads integers, finds the largest of them, and counts its occurrences. Assume that the input ends with number 0. Suppose that you entered 3525050; the program finds that the largest is 5 and the occurrence count for 5 is 3. (Hint: Maintain
two variables, max and count. max stores the current max number, and count stores its occurrences. Initially, assign the first number to max and 1 to count. Compare each subsequent number with max. If the number is greater than max, assign it to max and reset count to 1. If the number is equal to max, increment count by 1.)
The program should support up to 50 numbers as input. Only numbers 0-9 will be checked
Java Program-
import java.util.Scanner; public class problem4 { public static void main(String args[]) { Scanner input = new Scanner(System.in); int largest = 0; int occurrence = 0; int number; //storing command line argument in string s String s=args[0]; //splitting s into array of individual letters String arr[]=s.split(""); //going through each letter in array for(int i=0;i //parsing each string into integer number=Integer.parseInt(arr[i]); //if the current integer is greater than the largest number till now, then update the largest with current number if(number>largest){ occurrence=1; largest=number; } //if the largest number repeats then increment occurrence variable by 1 else if(number==largest){ occurrence++; } } //Displaying the results System.out.println("The largest number is " + largest+" The occurrence count is " + occurrence); } }