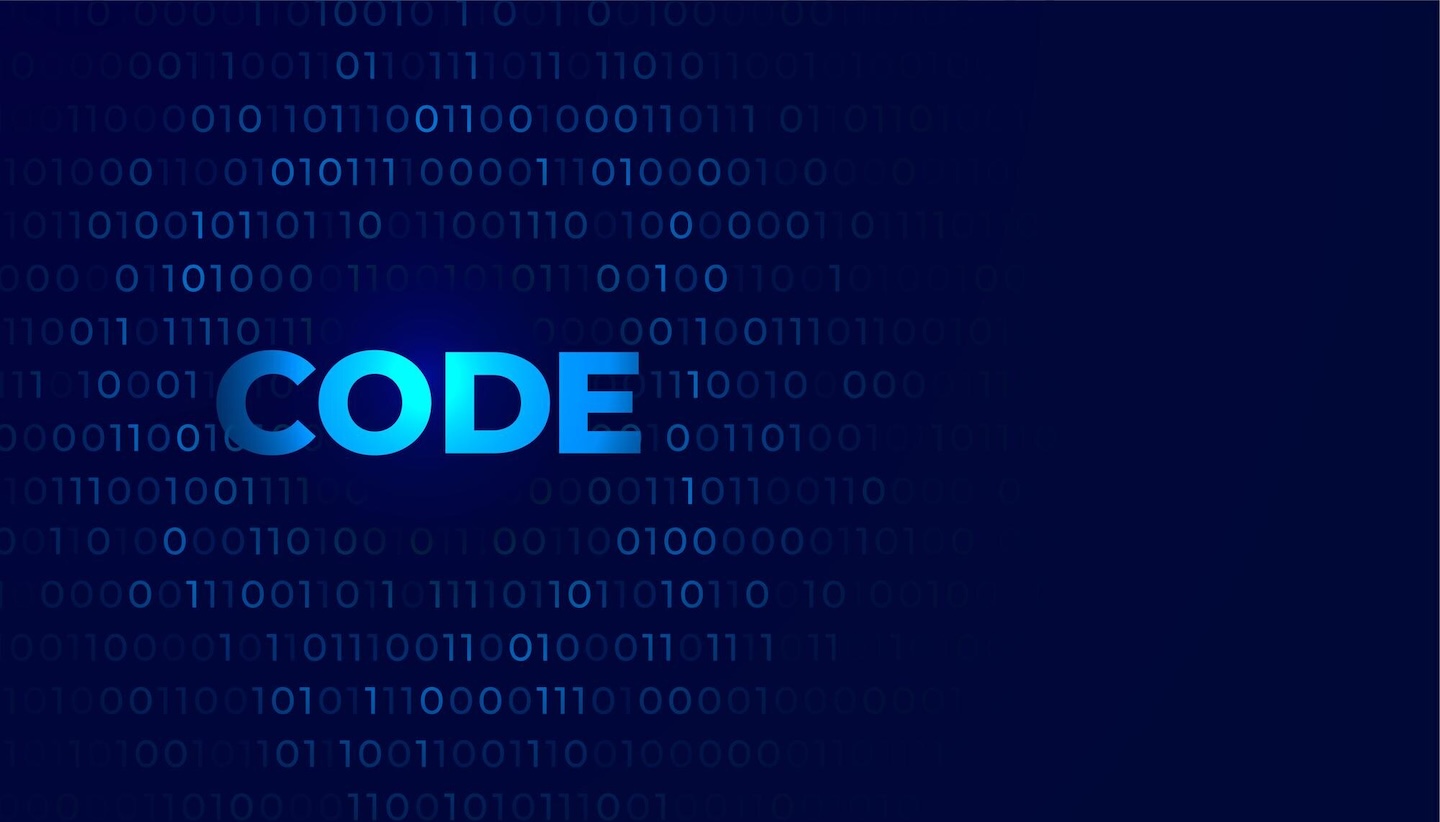
- 27th Dec 2023
- 14:39 pm
- Admin
In the domain of Python data processing, a key operation involves converting a list into a DataFrame. DataFrames, made possible by the popular Pandas library, present a structured and effective method for analyzing and exploring data.
- Creating a List:
Before diving into the conversion process, let's understand the simplicity of creating a list. A Python list is a straightforward collection of elements, representing data in various forms, such as numbers or text.
# Creating a list
my_list = [1, 2, 3, 4, 5]
- Using pandas.DataFrame() Method:
To turn a list into a DataFrame, you can use the pandas.DataFrame() method. This handy function organizes the list into a table-like structure, laying the groundwork for further data analysis.
# Converting a list to a DataFrame
import pandas as pd
my_list = [1, 2, 3, 4, 5]
df = pd.DataFrame(my_list, columns=['Column_Name'])
- Column Naming and Indexing:
A significant perk of using a DataFrame is the ability to assign meaningful names to columns. During DataFrame creation, specifying column names enhances data organization.
# Specifying column names and setting an index
df = pd.DataFrame(my_list, columns=['Value'], index=['Row1', 'Row2', 'Row3', 'Row4', 'Row5'])
- Handling Nested Lists:
In practical scenarios, data complexity may involve nested lists. Pandas addresses this by allowing the creation of hierarchical DataFrames.
# Creating a DataFrame from nested lists
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
df_nested = pd.DataFrame(nested_list, columns=['A', 'B', 'C'])
- Customizing Data Types:
DataFrames offer flexibility in dealing with different data types. Explicitly specifying the data type for each column during DataFrame creation is a straightforward process.
# Specifying data types for each column
df = pd.DataFrame(my_list, columns=['Values'], dtype=float)
- Appending Multiple Lists:
When data is scattered across multiple lists, combining them into a single DataFrame is achievable using methods like zip() or pandas.concat().
# Combining multiple lists into a DataFrame
list1 = [1, 2, 3]
list2 = ['A', 'B', 'C']
df_combined = pd.DataFrame(list(zip(list1, list2)), columns=['Numeric', 'Alphabetic'])
- Dealing with Missing Data:
Managing missing values is a crucial aspect of data processing. Pandas provides methods to fill or exclude missing values during DataFrame creation.
# Handling missing values during DataFrame creation
list_with_missing = [1, 2, None, 4, 5]
df_with_missing = pd.DataFrame(list_with_missing, columns=['Values']).dropna()
Example Scenarios:
The versatility of converting lists to DataFrames shines in real-world applications. Whether analyzing sales data, cleaning datasets, or integrating external information, DataFrames streamline these processes.
# Real-world example: Creating a DataFrame from a CSV file
import pandas as pd
# Assuming 'data.csv' contains tabular data
df_from_csv = pd.read_csv('data.csv')
Comparisons with Other Data Structures:
While lists serve well for storing simple sequences of data, DataFrames outshine them in terms of structure and functionality. Comparing DataFrames with other data structures like lists, arrays, or dictionaries underscores the advantages of using DataFrames for complex data manipulations.
Benefits of List to DataFrame in Python
- Structured Representation: DataFrames offer a structured and tabular format for clear data representation. Enables organized indexing, slicing, and filtering, facilitating efficient data analysis.
- Meaningful Column Names: Users can assign meaningful names to columns, enhancing data clarity. Allows for intuitive referencing and improved communication of data semantics.
- Customized Data Types: DataFrames support specifying data types for each column, ensuring data integrity. Facilitates efficient handling of various types of data within the same DataFrame.
- Effective Handling of Missing Data: Pandas provides methods for handling missing values during DataFrame creation. Offers flexibility in addressing null values to maintain data completeness.
- Seamless Data Merging: Enables the seamless merging of multiple lists or DataFrames. Facilitates the integration of diverse datasets for comprehensive and holistic analysis.
- Simplified Data Analysis: DataFrames streamline Data analysis tasks through built-in functions and methods. Simplifies common data manipulation operations, enhancing developer productivity.
- Interoperability with Pandas Ecosystem: It works well with other pandas features, broadening the possibilities for manipulating data. This is part of a larger system that supports tasks like visualizing data, conducting statistical analyses, and working on machine learning projects.
- Enhanced Readability and Documentation: Enhances code readability and promotes collaboration among developers when working with DataFrames. Also aids in documenting data processing steps, contributing to a clearer understanding of the project.
About the Author - Jane Austin
Jane Austin is a 24-year-old programmer specializing in Java and Python. With a strong foundation in these programming languages, her experience includes working on diverse projects that demonstrate her adaptability and proficiency in creating robust and scalable software systems. Jane is passionate about leveraging technology to address complex challenges and is continuously expanding her knowledge to stay updated with the latest advancements in the field of programming and software development.