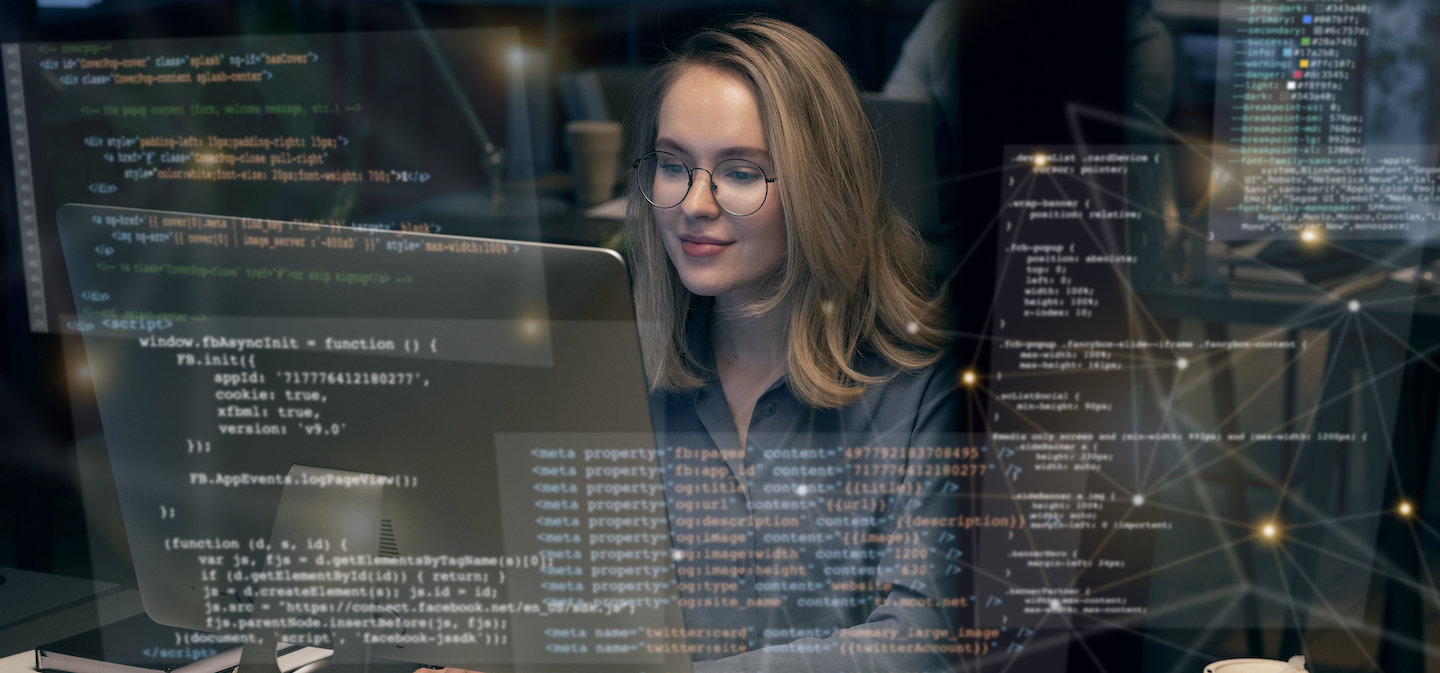
- 19th Dec 2023
- 14:37 pm
- Admin
JavaScript, often abbreviated as JS, stands out as a robust programming language extensively employed for crafting dynamic content on websites. Initially designed for execution within web browsers, JS has undergone significant evolution, expanding its utility to encompass server-side programming through platforms like Node.js. Conceived by Netscape, this language swiftly emerged as a pivotal element in web development, valued for its capability to enhance user interfaces and introduce interactivity to online platforms.
JavaScript, known for its versatility, is a sophisticated and user-friendly programming language. Developers leverage its high-level, interpreted nature to implement real-time changes to online content seamlessly, eliminating the need for page reloads. The language's adaptability spans a diverse range of programming paradigms, such as object-oriented, imperative, and functional techniques. This broad flexibility makes JavaScript a top pick for crafting engaging and responsive online applications.
The appeal of JavaScript lies in its ability to cater to various programming styles, providing developers with creative freedom. Whether building object-oriented structures, implementing imperative logic, or utilizing functional programming concepts, JavaScript accommodates diverse approaches. This adaptability contributes to its popularity in the construction of modern, user-centric software.
JavaScript's role extends beyond mere functionality—it is a key player in creating dynamic and entertaining online applications. Its extensive use in modern software development attests to its efficacy and good impact on the field of web development. JavaScript is a vital tool for creating feature-rich, responsive, and pleasant web applications because of its ongoing expansion, which is supported by a large ecosystem of libraries and frameworks.
Asynchronous programming, which is essential for activities like fetching data from servers without freezing the user interface, is included in modern JS.
JSON (JavaScript Object Notation):
JSON, an acronym for JavaScript Object Notation, serves as a straightforward and human-readable data interchange format. Its design facilitates readability and writability by humans while also enabling machines to efficiently parse and synthesize data. Although not tied to any specific programming language, JSON's syntax is grounded in JavaScript object notation.
In the JSON structure, data is expressed as key-value pairs enclosed in curly braces, with the inclusion of arrays and nested objects. Its user-friendly nature makes it a prevalent choice for facilitating data exchange between servers and web applications. JavaScript provides built-in methods, such as 'JSON.parse()' and 'JSON.stringify()', to seamlessly convert JSON data to and from JavaScript objects.
Developers widely employ JSON to streamline communication and interaction between servers and web applications. Its simplicity, clarity, and compatibility with various programming languages have positioned it as a preferred format for APIs (Application Programming Interfaces). As a result, JSON has become an integral component of modern web development, fostering efficient data transmission and interoperability across diverse software environments.
What are the uses of JS JSON
JSON is a lightweight data exchange format popular in web development and beyond. Here are some JavaScript and JSON usage examples:
- Data Exchange Format:
JSON is a data format used to transfer data between a server and a web application. Because of its simplicity and accessibility, JSON is frequently used for data transport in APIs (Application Programming Interfaces).
- AJAX Communication:
Asynchronous JavaScript and XML (AJAX) requests, which are routinely used to update parts of a web page without reloading the entire page, frequently employ JSON to communicate data between the web browser and the server. JSON and JavaScript's asynchronous nature enables for more dynamic and responsive user experiences.
- Configuration Files:
JSON is used in a variety of applications for configuration files. Its simple syntax makes it simple for developers to create and manage configuration settings for software, databases, and other systems.
- Local Data Retrieval and Storage:
JSON is used in web browsers to store and retrieve data locally. LocalStorage and sessionStorage are web storage systems that use JSON to format and manage data stored in the form of key-value pairs.
- Serialisation and Deserialization:
JSON is used to serialise complicated data structures into an easily transferred or saved format. Serialisation is the process of translating data into a JSON string, while deserialization is the process of converting a JSON string back into the original data structure. This comes in handy when transferring data to a server or saving it to a file.
- JavaScript Object Configuration:
JSON syntax is quite similar to JavaScript object notation, which makes configuring and initialising JavaScript objects simple. Developers typically use JSON to declare and build object instances with predefined characteristics and values.
- Cross-Origin Communication:
In web development, JSON is essential for cross-origin communication. Web browsers implement a same-origin policy to prevent direct communication between web pages from different domains due to security concerns. JSONP (JSON with Padding) and Cross-Origin Resource Sharing (CORS) are JSON-based approaches for facilitating safe cross-origin communication.
- Server Data Exchange:
JSON is often used to send and receive data between a client (web browser) and a server. JSON provides a standardised format for data interchange when a client delivers data to a server or requests data from a server.
JSON is important in many parts of web development because it provides a comprehensible and effective way of arranging and sending data between different components of an online application.
Characteristics of JSON
JSON (JavaScript Object Notation) is a lightweight data transmission format noted for its ease of use, readability, and simplicity. Here are some of JSON's important characteristics:
- Human-Readable Format: JSON data is simple to read and write for humans. Its syntax is simple and straightforward, making it suitable for both coders and non-programmers.
- Lightweight: JSON is a lightweight format, which means it doesn't have any needless overhead. This makes it suitable for data transfer and storage, particularly in web-based environments.
- Textual Representation: Because JSON is represented as plain text, it is platform agnostic. This text-based format is simple to generate, interpret, and debug, and it is compatible with a wide range of programming languages.
- Key-Value Pairing: JSON organises data using key-value pairs. Each item of data is assigned a unique key, resulting in a systematic means of representing information.
- Supports Nested Structures: JSON supports nested structures, allowing complicated data hierarchies to be represented. Arrays can include objects, and arrays can contain other objects, allowing for the building of flexible data structures.
- Data kinds: JSON supports a variety of data kinds such as texts, numbers, booleans, arrays, objects, and null. This adaptability enables the depiction of a wide range of facts.
- Language-Independent: Despite its name implying a connection to JavaScript, JSON is a language-independent format. It is compatible with almost every computer language, giving it a versatile option for data interchange.
- Simple Syntax: JSON has an easy-to-understand syntax with few rules. It is made up of key-value pairs, objects surrounded by curly braces '', and arrays denoted by square brackets '[]'. This simplicity helps to its usability and acceptance.
- Widely Supported: JSON is supported by a wide range of programming languages, making it the de facto standard for web data transmission. For working with JSON, most current programming languages include libraries or built-in functions.
Overall, these traits help to explain JSON's extensive adoption and effectiveness as a data transfer format in a variety of disciplines, including web development and API design.
JS JSON Program
A simple JavaScript program that uses JSON. In this example, we'll create a list of books, convert it to a JSON string, and then parse it back to a JavaScript object.
```
// Define a list of books
const books = [
{ title: 'The Great Gatsby', author: 'F. Scott Fitzgerald', year: 1925 },
{ title: 'To Kill a Mockingbird', author: 'Harper Lee', year: 1960 },
{ title: '1984', author: 'George Orwell', year: 1949 },
{ title: 'Pride and Prejudice', author: 'Jane Austen', year: 1813 }
];
// Convert the list of books to a JSON string
const booksJSONString = JSON.stringify(books);
// Display the JSON string
console.log("JSON String:");
console.log(booksJSONString);
// Parse the JSON string back to a JavaScript object
const parsedBooks = JSON.parse(booksJSONString);
// Display the parsed JavaScript object
console.log("\nParsed JavaScript Object:");
console.log(parsedBooks);
```
Explanation:
Make a Reading List: We made an array called 'books' that contains objects representing various books. Each book includes information such as "title," "author," and "year."
- JSON String Conversion: The array of books is converted to a JSON-formatted string using
- the 'JSON.stringify()' method. This string representation is saved in the variable 'booksJSONString'.
- Display JSON String: We report the JSON string to the console to demonstrate how the data appears serialised.
- JSON.parse(): The 'JSON.parse()' method is used to turn the JSON text back into a JavaScript object. The outcome is saved in the variable 'parsedBooks'.
- Display Parsed JavaScript Object: We log the parsed JavaScript object to the console to show how quickly the serialised JSON text can be converted back to a useable data structure.
We can quickly send and store structured data using JSON, making it a useful format for a variety of applications, particularly when dealing with data exchange between different areas of a programme or talking with external APIs.