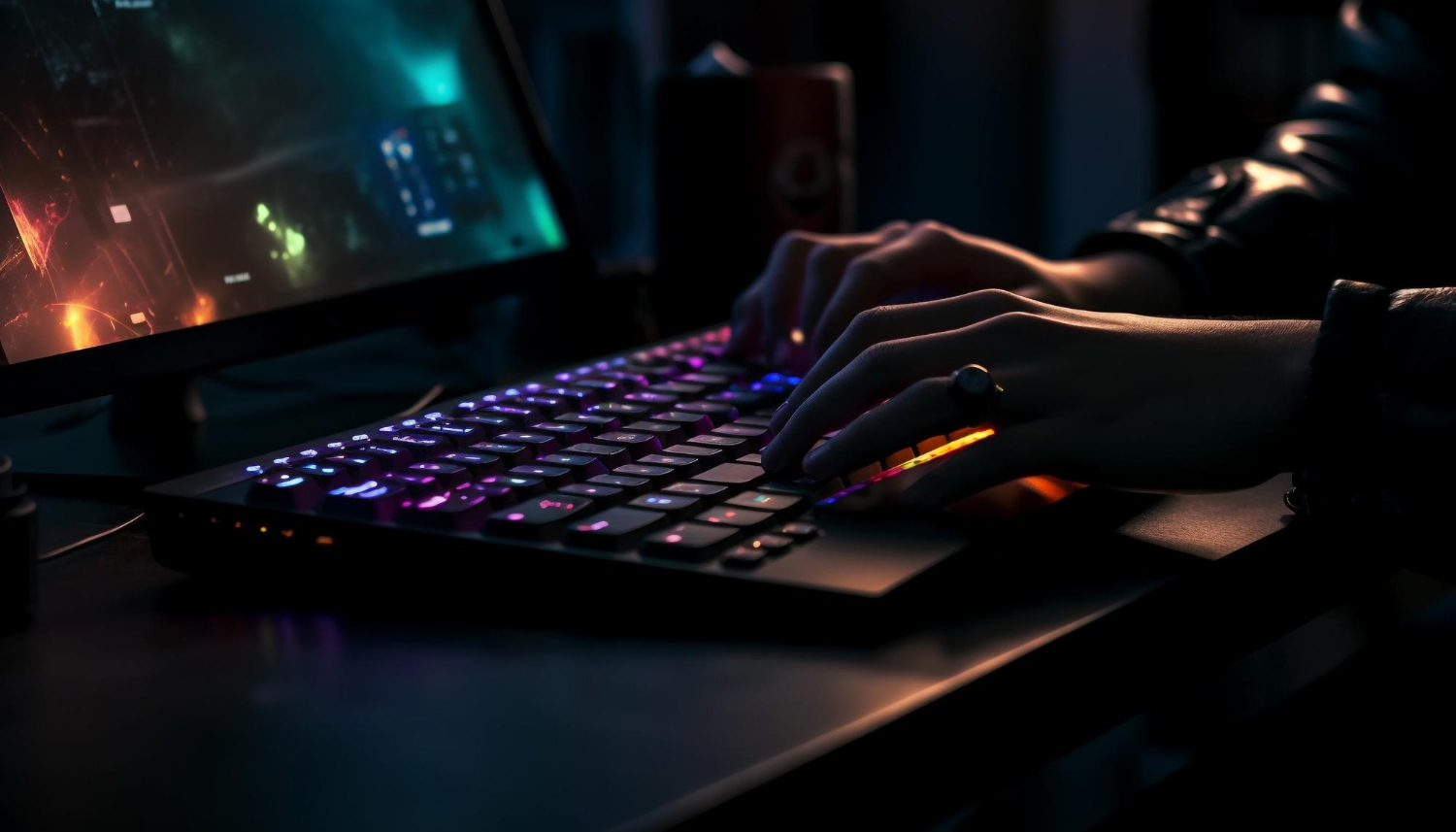
- 19th Dec 2023
- 15:27 pm
- Admin
Handling events in JavaScript entails dealing with user actions or movements on a webpage. These actions can include clicks, keystrokes, mouse movements, form submissions, and more. Events are similar to signals that inform the program that something has occurred. When an event occurs, JavaScript can be configured to respond to it, allowing the webpage to become interactive.
Consider events to be actions taken by users on a website, such as clicking a button or completing a form. Handling occurrences is analogous to preparing the code to respond when certain events occur. It requires scheduling particular activities or functions to take place when certain occurrences take place.
Imagine a moment when you click a "Submit" button on a form—that's an event. JavaScript can step in and manage this event by checking what you put in the form and doing things like sending it to a server.
Knowing how to handle events is crucial when you're making web pages that respond right away. It's like a trick to make a website more lively and interesting. Users can interact with the content, making their time on the site more fun and enjoyable.
Types of Event Handling in JavaScript
In JavaScript, there are many types of event handling that allow developers to respond to various user actions on a webpage. These categories contribute to the creation of dynamic and interactive internet applications. Here are a couple of such examples:
- Clicking on Events: When a user clicks on an element like a button or a link, something happens. It is frequently used to start actions such as submitting forms or moving to another page.
- Main Events: Keyboard activities such as pressing and releasing keys cause key events to occur. This is useful for creating keyboard shortcuts or validating user input in forms.
- Mouse Events: When you use the mouse by moving it, clicking, or letting go of a button, mouse events happen. These events are really important for making things like drag-and-drop work on a webpage.
- Form Events: Form events occur when users interact with form components, such as submitting or resetting them. These events are commonly utilised in data validation and submission.
- Events of Change: When the value of an input field or a dropdown menu changes, a change event occurs. This is commonly used to dynamically change the material based on user input.
- Load and Unload Events: Load events happen when a webpage finishes loading, and unload events happen when a user navigates away from the page. These events can be used to start or stop resources.
For developers aiming to make friendly and responsive web apps, it's crucial to grasp these types of events. When developers use these events well, it helps enhance how users experience the website, making it more interesting and engaging.
Applications of Cases of Event Handling in JavaScript
Event handling in JavaScript serves a variety of practical functions, including improving the user experience on websites. Here are some examples of common applications:
- Form Validation: Validating user input in forms requires event management. Developers can check if entered information fits specified criteria before processing or sending it by listening for events such as form submission.
- Interactive Buttons: When a button on a webpage is clicked, it frequently triggers actions or changes. Developers can describe what happens when a button is clicked, making it interactive and responsive.
- Real-time Updates: Keystrokes or mouse movements can cause real-time updates on a webpage. This is useful in applications where users want immediate feedback, such as chat interfaces or collaborative editing.
- Dynamic Content Loading: Event handling is used to dynamically load extra content. For example, when a user scrolls to the bottom of a page, an event can cause more content to load without requiring a page refresh.
- Image Sliders and Carousels: Image sliders and carousels leverage events like as clicks and swipes. These events allow visitors to travel through a sequence of photos, making the experience dynamic and entertaining.
- Modal Windows and Popups: Modal windows and popups frequently rely on events to work. For example, clicking a button may cause a modal window to appear, allowing for a concentrated interaction without leaving the website.
- Form Autofill Suggestions: Autofill suggestions can be triggered by events connected to input fields, boosting user convenience when filling out forms.
- User Authentication: During the user authentication procedure, event handling is used. For example, handling events on a "Log In" button to validate user credentials and begin a login sequence.
Developers may design dynamic, responsive, and user-friendly web apps that accommodate to a wide range of user interactions and circumstances by efficiently implementing event handling.
JavaScript Program for Handling Events
The program uses a method called addEventListener to connect a click event listener to a button. When someone clicks the button, the program runs a function that alters the text in a paragraph.
```
Event Handling Example
Click Me
Waiting for a click...
```
Explanation:
- The HTML has a button with the ID `myButton` and a paragraph with the ID `output`.
- In the JavaScript part, 'document.getElementById' is used to get references to the button and paragraph.
- When the button is clicked, the 'handleClick' function is ready to change the text in the paragraph.
- The 'addEventListener' method sticks a click event listener to the button, saying that when it's clicked, the 'handleClick' function should do its thing.
If you open this HTML in a web browser and click the "Click Me" button, the paragraph text changes to "Button clicked! It's a basic event-handling situation where a function gets activated when a user does something.